Rename Excel Sheet Easily with VBA: Quick Guide

Managing large sets of data in Microsoft Excel often requires renaming multiple sheets to keep things organized. Instead of manually renaming each sheet, you can use Visual Basic for Applications (VBA) to streamline this process. This guide will show you how to rename Excel sheets quickly and efficiently using VBA, enhancing your productivity and ensuring consistency across your work.
Why Use VBA to Rename Sheets?

Visual Basic for Applications, commonly known as VBA, is Excel's integrated programming language that allows you to automate repetitive tasks. Here's why using VBA for renaming sheets can be beneficial:
- Time-Saving: Automate the renaming process for numerous sheets in seconds, instead of manually doing it.
- Consistency: Ensure uniformity in naming conventions, reducing errors.
- Flexibility: Easily implement complex renaming rules that would be tedious to do manually.
Setting Up VBA in Excel
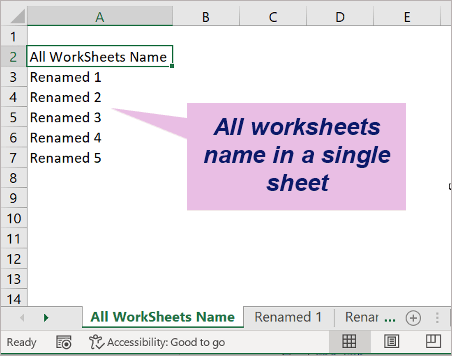
Before diving into the code, you need to ensure VBA is enabled in your Excel:
- Open Excel and go to File > Options.
- Select Customize Ribbon from the left menu.
- Check the box next to Developer in the right column.
- Click OK to add the Developer tab to your Ribbon.
⚠️ Note: Ensure macros are enabled to avoid issues when running VBA scripts.
Writing the VBA Code to Rename Sheets

Here's how you can write a VBA script to rename your Excel sheets:
Open VBA Editor

Click on the Developer tab, then select Visual Basic to open the VBA editor.
Insert a New Module

In the VBA editor:
- Go to Insert > Module to create a new module.
VBA Code for Renaming

Copy and paste the following code into your new module:
Sub RenameSheets()
Dim ws As Worksheet
Dim newName As String
Dim i As Integer
i = 1
For Each ws In ThisWorkbook.Worksheets
newName = "Sheet" & i ' You can customize the naming rule here
If Not ws.Name = "Sheet1" Then ' Skip renaming if the sheet is already named "Sheet1"
ws.Name = newName
i = i + 1
End If
Next ws
End Sub
💡 Note: This script renames all sheets except "Sheet1" to avoid overwriting any default Excel sheet name. Modify the naming rule as needed for your specific use case.
Running the VBA Script

To execute the script:
- Go back to Excel.
- Press ALT + F8 to open the Macro dialog box.
- Select RenameSheets and click Run.
Advanced Sheet Renaming Techniques

For more advanced renaming:
Prefix with Dates or Dynamic Names

You can incorporate dynamic elements like dates or sequential numbers:
Sub RenameWithDate()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = Format(Now(), "yyyy-mm-dd") & "_" & ws.Index
Next ws
End Sub
Batch Rename with User Input

To allow user input for each sheet:
Sub BatchRename()
Dim ws As Worksheet
Dim newName As String
For Each ws In ThisWorkbook.Worksheets
newName = InputBox("Enter new name for " & ws.Name, "Rename Sheet", ws.Name)
If newName <> "" Then
ws.Name = newName
End If
Next ws
End Sub
FAQs

Can VBA rename sheets based on cell values?

+
Yes, VBA can rename sheets dynamically based on the content of specific cells. You would need to reference these cells within the renaming script to fetch the new names.
What if my sheet names are too long or contain invalid characters?

+
Excel sheet names are limited to 31 characters. If your script tries to use a name longer than this, it will be truncated. Invalid characters like *:/?[] will also be rejected. Ensure your script handles these constraints.
Is it possible to reverse the renaming if I make a mistake?

+
Yes, you can write another script to revert names by saving the original names before renaming or keeping a backup of your workbook before any renaming operation.
By automating the renaming of sheets in Excel with VBA, you not only save time but also ensure your spreadsheets are structured and consistent. Whether it’s a simple task or a complex operation involving user input or data-driven names, VBA provides the tools to make Excel work more efficiently for you. Remember, with great power comes great responsibility—always be cautious when running scripts that modify your data, and keep backups where necessary.