Unlock Excel VBA Secrets: Safeguard Your Sheets Easily

Learning to safeguard your Excel spreadsheets can seem daunting, especially when considering how to manage a large workforce's access to sensitive data securely. Excel VBA (Visual Basic for Applications) offers a robust solution, enabling you to set up password protections, restrict data entry, and even automate tasks to enhance productivity. This comprehensive guide will walk you through the steps to unlock the secrets of Excel VBA for securing your sheets with ease.
Understanding VBA and Security
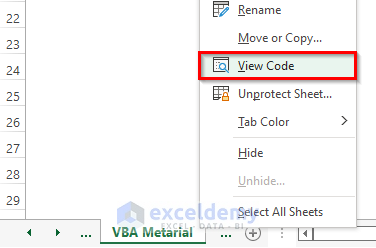
Excel VBA is an integrated development environment (IDE) for programming in Excel. It allows you to automate tasks, create custom user interfaces, and importantly, enhance the security of your spreadsheets. Here’s why securing Excel sheets matters:
- Data Privacy: Protecting sensitive information from unauthorized access.
- Integrity: Preventing accidental or malicious changes to your data.
- Compliance: Ensuring you meet regulatory requirements.
Setting Up Passwords in Excel VBA

Let’s start by exploring how to use VBA to set up a password for your sheets. Here’s a simple example:
Sub ProtectSheet()
ActiveSheet.Protect Password:="YourPassword"
End Sub
This code snippet will password protect the active sheet with the password "YourPassword". Here are some considerations:
- Choose a strong password.
- Remember to update or change passwords regularly for security.
- Ensure you store your passwords securely, especially in a collaborative environment.
🔒 Note: If you forget the password, there is no built-in way to recover it, so backup your VBA scripts and document your passwords.
Controlling User Access

Excel VBA allows you to control what users can and cannot do on your sheets. Here’s how to tailor user permissions:
Setting | Description |
---|---|
AllowFormattingCells | Lets users format cells while keeping cell values intact. |
AllowSorting | Permits users to sort data but not alter it. |
AllowFiltering | Enables data filtering by users. |

Below is the code to apply these settings:
Sub CustomizePermissions()
With ActiveSheet
.Protect Password:="YourPassword", _
AllowFormattingCells:=True, _
AllowSorting:=True, _
AllowFiltering:=True
End With
End Sub
Automating Sheet Protection

Automation can save time by protecting your sheets when specific conditions are met. Here’s an example:
Sub AutoProtectOnSave()
Dim wb As Workbook
Set wb = ThisWorkbook
If wb.Saved = False Then
ActiveSheet.Protect Password:="YourPassword"
wb.Save
End If
End Sub
This macro will protect your active sheet and then save the workbook if it has not been saved yet. Automation like this ensures:
- Consistent security practices.
- Reduced human error.
Using VBA to Hide Formulas

Another aspect of security is hiding formulas, which prevents users from seeing how data is calculated or manipulated. Here's how to achieve this:
Sub HideFormulas()
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Cells.Locked = False
ws.Cells.FormulaHidden = True
ws.Protect Password:="YourPassword"
End Sub
This script will:
- Unlock all cells to change the formula visibility setting.
- Set formulas to hidden.
- Relock and protect the sheet with a password.
Final Thoughts

Securing your Excel workbooks using VBA can significantly enhance the safety and integrity of your data. From setting up passwords to controlling user permissions, and automating tasks, VBA provides versatile tools to meet various security needs. Remember, while these methods can secure your spreadsheets, the security of your information is ultimately your responsibility. Use these techniques wisely, and always keep backups of your scripts and data.
Can I recover a lost password for an Excel sheet?

+
No, there is no built-in way in Excel to recover lost passwords for sheets or workbooks. Always keep secure records of your passwords or consider using third-party recovery tools, but be cautious about their legitimacy and effectiveness.
What can users still do with a protected sheet?

+
Users can still interact with sheets by:
- Entering data into unlocked cells.
- Viewing cell data.
- Sorting or filtering if allowed by the permissions set.
Is there a way to unprotect multiple sheets at once?

+
Yes, you can use VBA to unprotect multiple sheets by iterating through each sheet in the workbook and applying the unprotect method:
Sub UnprotectAllSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Unprotect Password:=“YourPassword”
Next ws
End Sub