Create New Excel Sheets in R: Easy Guide

Managing data in R can be a formidable task, especially when it involves multiple datasets or separate sheets within the same Excel file. Excel is a staple tool in data analysis, and its integration with R can streamline workflows and enhance productivity. This guide will walk you through creating new Excel sheets in R, including setting up the environment, selecting data, and handling operations to manage these sheets effectively.
Setting Up the R Environment for Excel Handling

To start, you’ll need to ensure your R environment is ready for Excel manipulation. Here’s how you can set it up:
- Install Necessary Packages: Use
install.packages(“openxlsx”)
to get the openxlsx package, orinstall.packages(“xlsx”)
for the xlsx package if you prefer using Java for reading/writing Excel files. - Load Libraries: After installation, load the packages using
library(openxlsx)
orlibrary(xlsx)
.
Creating a New Excel Workbook and Adding Sheets
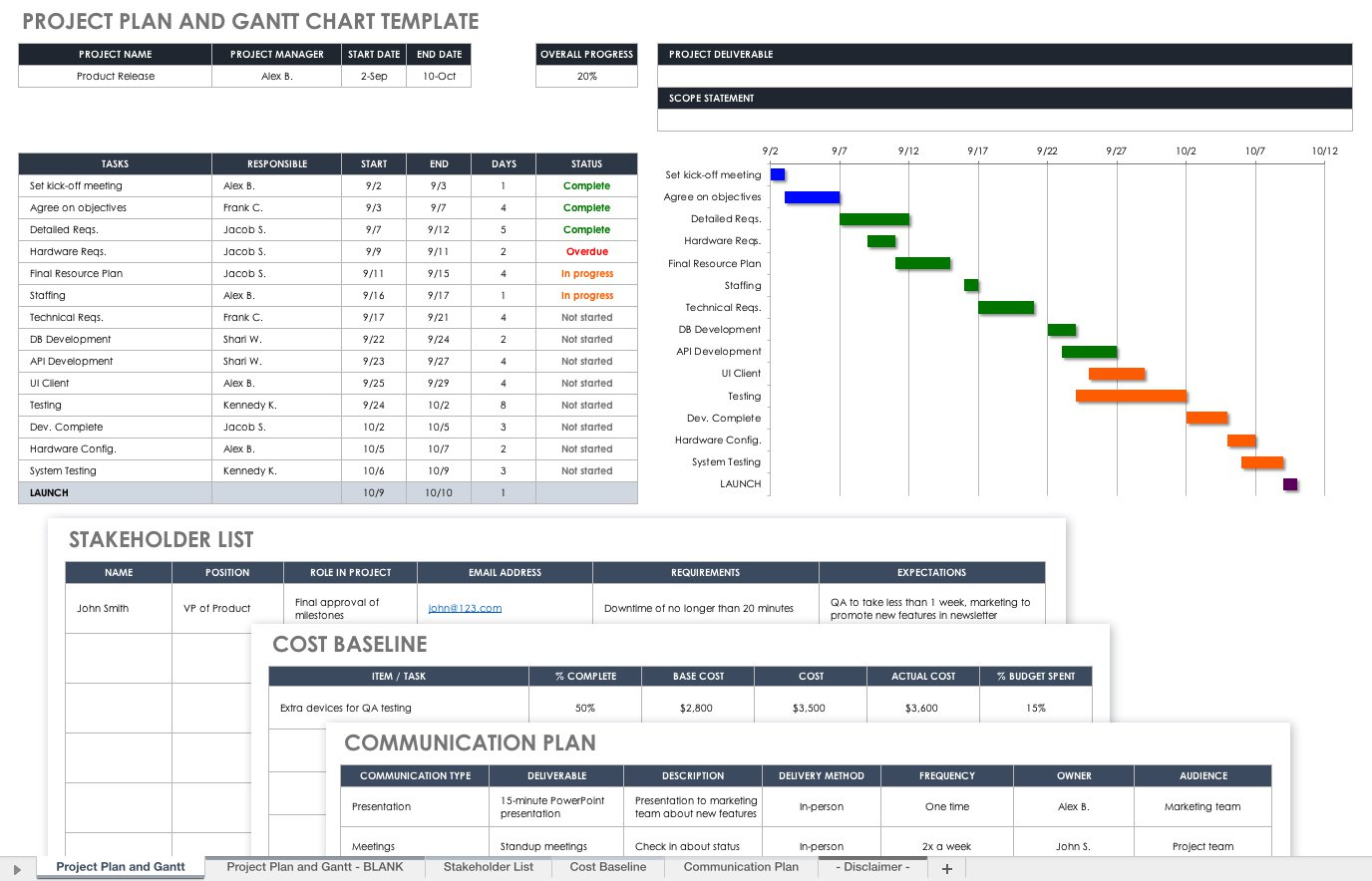
With the environment set up, you can proceed to create and manipulate Excel sheets:
- Creating a New Workbook:
wb <- createWorkbook()
- Adding a New Sheet:
addWorksheet(wb, “Sheet1”)
- Writing Data to the Sheet:
Here,writeData(wb, “Sheet1”, data)
data
is your data frame or data to be written into the sheet.
Advanced Sheet Management in Excel with R

Beyond just creating sheets, R provides functionality for various operations:
- Renaming Sheets: Use
renameWorksheet(wb, old_name, new_name)
to rename a sheet. - Copying Sheets: The
cloneWorksheet(wb, “Sheet1”)
function can be used to create a duplicate sheet. - Modifying Sheet Properties: You can change properties like color or protection using
setSheetProperty(wb, “Sheet1”, …)
.
Adding Tables, Charts, and Formatting

R also allows you to add dynamic tables, charts, and apply different formatting styles to your sheets:
- Creating Tables:
addTable(wb, “Sheet1”, data, styleName = “TableStyleLight8”)
- Inserting Charts:
chart <- addChart(wb, “Sheet1”, type = “column”, data = data, title = “Sales Chart”)
- Formatting Cells: Use functions like
addStyle
to apply styles to cells or ranges of cells.
Handling Data Across Multiple Sheets

When dealing with multiple sheets, R can help you manage them efficiently:
- Selecting Sheets:
getSheets(wb)
retrieves all sheets, andgetSheet(wb, “Sheet1”)
selects a specific one. - Copying Data Between Sheets: Use functions like
writeData
to copy data from one sheet to another. - Batch Sheet Creation:
for (i in 1:5) addWorksheet(wb, paste0(“Sheet”, i))
Saving and Exporting the Excel Workbook
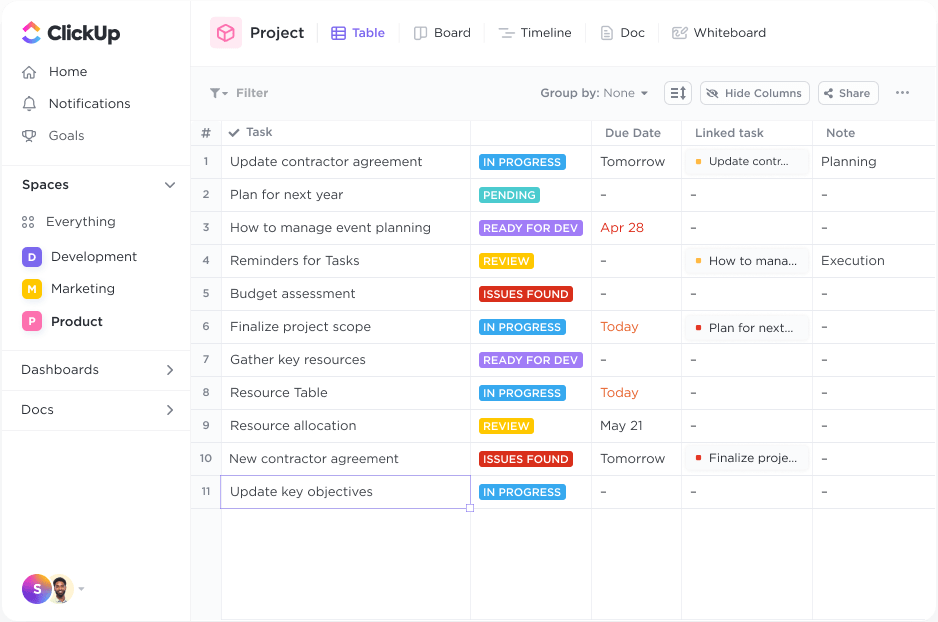
Once you’ve manipulated your data:
- Saving the Workbook:
saveWorkbook(wb, “MyData.xlsx”, overwrite = TRUE)
- Exporting in Different Formats: If needed, R can convert Excel files to CSV, PDF, or other formats with additional libraries.
💡 Note: Ensure to set overwrite = TRUE
in saveWorkbook
if you want to overwrite an existing file.
Mastering the creation and manipulation of Excel sheets in R opens up numerous possibilities for data management and presentation. Whether it's for reporting, data analysis, or sharing results, R's integration with Excel provides powerful tools for data scientists and analysts. Remember to keep your R code clean, leverage functions for modularity, and explore the documentation of packages like openxlsx for more advanced features.
The ability to generate complex spreadsheets programmatically can significantly reduce manual work, improve accuracy, and enhance data visualization. With practice, you can handle even the most complex Excel manipulations directly within R, making your workflow more efficient and your analyses more sophisticated.
What packages are commonly used for Excel manipulation in R?

+
The most commonly used packages for Excel manipulation in R are openxlsx, xlsx, readxl, and writexl. Each has its strengths, with openxlsx being popular for creating workbooks and performing advanced Excel features without Java.
Can R handle Excel files with multiple sheets?

+
Yes, R can easily manage multiple sheets within an Excel file. You can add, remove, rename, and manipulate sheets using functions from packages like openxlsx or xlsx.
Is it possible to add charts to Excel sheets in R?

+
Yes, using libraries like openxlsx, you can create charts, tables, and apply various formatting options directly from R.