5 Ways to Switch Active Sheets in Excel VBA

In the realm of Excel VBA, manipulating workbooks and sheets is a fundamental skill that can significantly boost your efficiency. Whether you're dealing with complex data analysis, reporting, or automating repetitive tasks, knowing how to switch active sheets can streamline your processes. Here's an in-depth exploration of five different methods to switch between active sheets in Excel using VBA:
Method 1: Using the Select Method

The simplest way to switch to another sheet is by using the Select
method. This method directly makes the specified sheet active:
Sub SwitchSheetBySelect()
Sheets("Sheet2").Select
End Sub
This code selects "Sheet2" making it the active sheet. Here's how you can use it effectively:
- Specify the sheet name in the
Sheets()
object. - If the sheet name contains spaces, use single quotes around it, like
Sheets("'Sheet 2'").Select
. - Use caution with this method since it changes the user's focus, potentially disrupting their workflow.
Important Note:
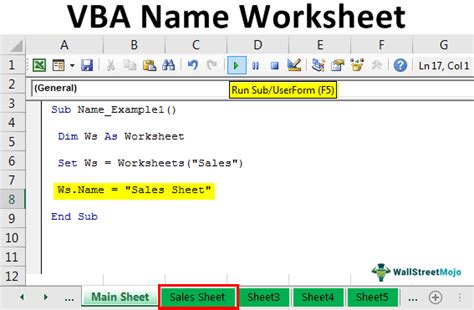
đź’ˇ Note: The Select
method moves the cursor to the selected sheet, which might not always be desired.
Method 2: Activating a Sheet with Activate

Similar to Select
, but more specific to switching sheets without necessarily changing the user’s focus:
Sub ActivateSheet()
Sheets("Data").Activate
End Sub
- This method makes the sheet active without moving the cursor to it, preserving user interaction with other parts of Excel.
- Activate is less disruptive than Select for automation tasks.
Method 3: Using CodeName for More Robust Selection

Using sheet code names provides a more stable way to reference sheets, particularly when sheet names might change:
Sub SwitchByCodeName()
Sheet2.Activate
End Sub
Sheet2
here refers to the sheet code name, not its visible name.- This method is resilient to name changes of the sheet.
Note:

🔧 Note: You can find the sheet’s code name in the Visual Basic Editor under the project explorer.
Method 4: Indexing Sheets with Sheets(Index)

Another method involves using the sheet index within the workbook:
Sub IndexSheetSwitch()
Sheets(2).Activate
End Sub
- This method uses the position of the sheet within the workbook.
- Be cautious, as adding or removing sheets might change this index.
Method 5: Looping Through Worksheets

This method is useful for scenarios where you need to work through multiple sheets:
Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Activate
' Perform tasks on this sheet
Next ws
End Sub
- Loop through all sheets or perform conditional operations.
- Ideal for automation tasks across multiple sheets.
Understanding and implementing these methods in your VBA scripts can vastly improve your productivity in Excel. Here are some general notes for better VBA practice:
🔍 Note: Always ensure you have saved your workbook before running VBA code to avoid data loss.
By employing these techniques, you can manage your data with greater efficiency:
- Choose methods based on your task requirements, considering stability and user interaction.
- Be mindful of user experience, especially with methods like
Select
that can alter the user's active environment.
In wrapping up, Excel VBA provides multiple avenues for managing sheet navigation, each with its benefits and considerations. These methods not only make your Excel VBA scripts more robust but also enhance your data manipulation capabilities, allowing for smoother transitions between sheets and more efficient data management. Whether you're automating a complex report or just organizing your data, these techniques will equip you with the necessary tools to excel in Excel VBA.
Which method is best for automating tasks?

+
For automation, Method 2: Activate or Method 5: Looping Through Worksheets are ideal as they ensure minimal disruption to the user interface.
Can I change the sheet without affecting user focus?

+
Yes, using the Activate method instead of Select will change the active sheet without moving the user’s cursor or altering their focus on the screen.
What if sheet names change or are deleted?

+
Using CodeName (Method 3) provides stability as it references the sheet’s internal name, which doesn’t change with visible name changes or deletions.
Is it possible to switch to a specific cell in a sheet?

+
Yes, you can combine sheet activation with cell selection, like this: Sheets(“SheetName”).Range(“A1”).Select
.
How can I switch to the next or previous sheet?

+
To switch to the next or previous sheet, you can use VBA like this: ActiveSheet.Next.Activate
for the next sheet, or ActiveSheet.Previous.Activate
for the previous one.