Loading Specific Excel Sheets into MATLAB: A Quick Guide

Working with Excel data in MATLAB can significantly enhance your data analysis capabilities. MATLAB provides several functions that simplify the process of importing, processing, and analyzing data stored in Excel files. In this detailed guide, we'll explore how to load specific sheets from an Excel file into MATLAB for various data manipulation and analysis tasks.
Understanding Excel File Structures

Before diving into MATLAB, it’s essential to understand how Excel files are organized:
- Workbooks: Excel files are often referred to as workbooks.
- Worksheets or Sheets: Within each workbook, you have sheets or worksheets where data is entered.
- Cell Range: Data within a sheet is referenced by row and column in a cell range format, like A1:B10.
Basic Importing with readtable

One of the simplest ways to import an entire Excel sheet into MATLAB is by using the readtable
function. Here’s how you can do it:
filename = ‘your_excel_file.xlsx’;
sheet = ‘Sheet1’;
data = readtable(filename, ‘Sheet’, sheet);
📝 Note: The readtable
function is versatile and can import different formats, but for Excel, it's particularly useful for structured data with headers.
Loading Specific Ranges

Sometimes you only need data from a specific range within a sheet. The readmatrix
, readcell
, or readtable
functions can be used with the Range
parameter:
filename = ‘your_excel_file.xlsx’;
sheet = ‘Sheet1’;
range = ‘A1:D10’; % Define your specific range here
data = readmatrix(filename, ‘Sheet’, sheet, ‘Range’, range);
You can mix and match readtable
, readmatrix
, or readcell
based on the type of data you expect in your range:
- readmatrix for numeric data.
- readcell for mixed or text data.
- readtable for tabular data with headers.
Multiple Sheets into Cell Array

If you're dealing with several sheets from the same Excel file, you can use a loop to load each sheet into a cell array:
filename = 'your_excel_file.xlsx';
numSheets = length(sheetnames(filename)); % Number of sheets
dataSheets = cell(1, numSheets);
for i = 1:numSheets
sheet = sheetnames(filename, i);
dataSheets{i} = readtable(filename, 'Sheet', sheet);
end
📝 Note: This approach saves time by loading all sheets at once rather than one by one.
Handling Special Cases

Date Time Data

Excel date formats can be problematic due to their conversion to numerical values. MATLAB can handle this by specifying the ‘DatetimeType’
parameter:
filename = ‘your_excel_file.xlsx’;
sheet = ‘Sheet1’;
data = readtable(filename, ‘Sheet’, sheet, ‘DatetimeType’, ‘datetime’);
Merged Cells

Merged cells in Excel might not import correctly if they span across your selected range. Here, you might need to handle them in MATLAB post-import:
- Identify merged cells by looking for repeating values in the relevant columns or rows.
- Use logical indexing or fill missing data with appropriate methods like
fillmissing
.
Formulas

Excel formulas might not directly import the calculated value. You might want to:
- Set Excel to save the workbook with current values instead of formulas.
- Perform necessary calculations within MATLAB after import if you require formula-specific results.
Error Handling
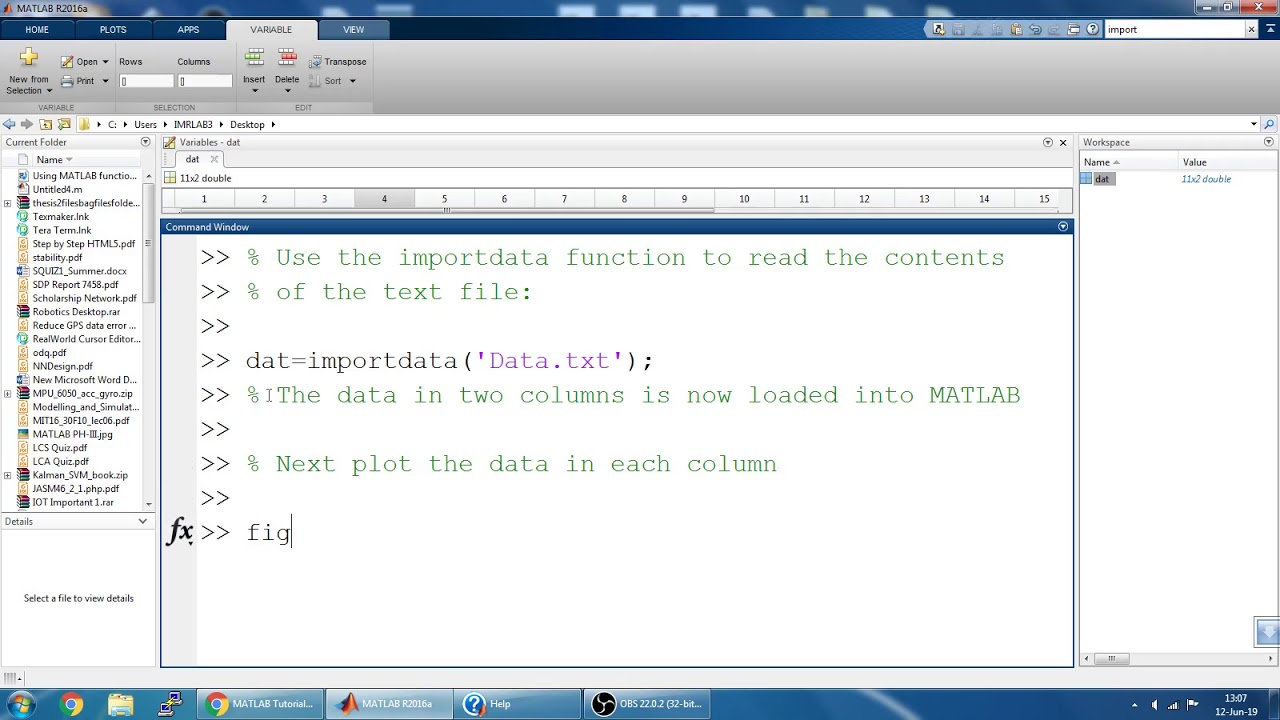
Data import might fail due to various reasons like incorrect filenames, sheet names, or incompatible data. Here are some tips to manage these errors:
- Use
try-catch
blocks to handle exceptions gracefully:
try
data = readtable(filename, ‘Sheet’, sheet);
catch ME
fprintf(‘Error loading data from %s, Sheet: %s. Error Message: %s\n’, filename, sheet, ME.message);
data = []; % or return empty or some default value
end
📝 Note: Error handling ensures your script can proceed even if one part fails, providing a better user experience.
The journey of importing Excel sheets into MATLAB involves understanding how Excel organizes data, using the appropriate functions, and being prepared to handle special cases and potential errors. Whether you need to load one specific range, multiple sheets, or manage data from merged cells, MATLAB provides the tools necessary for seamless data analysis. This guide has hopefully equipped you with the know-how to efficiently bring your Excel data into MATLAB, setting you up for effective data manipulation and analysis.
What should I do if my Excel file has formulas?

+
When your Excel file contains formulas, you might want to save the workbook with current values before importing or use MATLAB to recalculate or interpret the formulas post-import.
How can I specify a range when importing data?

+
Use the ‘Range’ parameter in functions like readmatrix
, readcell
, or readtable
to specify the exact cell range you want to import from your Excel sheet.
How do I handle merged cells?

+
Identify merged cells by looking for repeated values or use logical indexing to fill data appropriately within MATLAB post-import.