5 Ways to Link Sheets in Excel VBA Easily

In the ever-evolving world of data analysis and automation, Microsoft Excel remains a cornerstone tool. Excel VBA (Visual Basic for Applications) provides a powerful platform for automating repetitive tasks, including linking data across multiple worksheets or workbooks. Linking sheets can significantly streamline your workflow, making data management more efficient. Here are five effective ways to link sheets in Excel VBA easily:
1. Using Named Ranges

Named ranges are a simple yet powerful way to reference cells or ranges of cells in different sheets. Here’s how you can use them:
- Define a Named Range: Go to Formulas > Name Manager, and create a named range.
- VBA Code to Use Named Range:
Sub UseNamedRange()
Dim NamedRange As Range
Set NamedRange = Range("SalesFigures") 'Assume "SalesFigures" is the named range
Debug.Print NamedRange.Value
End Sub
🔍 Note: Named ranges make your code more readable and less prone to errors due to cell reference changes.
2. Using the Range.Formula Property

This method lets you directly reference cells in other sheets through formulas:
- Formula Linking: Set a cell’s formula to reference another sheet.
- VBA Example:
Sub FormulaLink()
Range("A1").Formula = "=Sheet2!A1"
End Sub
3. Hyperlink to Another Sheet

Hyperlinks are useful for creating navigational ease within a workbook:
- Create Hyperlink: Use the HYPERLINK function or VBA to link cells.
- VBA Hyperlink:
Sub AddHyperlink()
ThisWorkbook.Worksheets("Sheet1").Hyperlinks.Add Anchor:=Range("B5"), Address:="", _
SubAddress:="Sheet2!A1", TextToDisplay:="Go to Data Sheet"
End Sub
4. Indirect Function for Dynamic References

The INDIRECT function allows for dynamic cell referencing based on text strings:
- Dynamic Reference: Create a reference that can change based on user input or other conditions.
- VBA with INDIRECT:
Sub DynamicReference()
Dim sheetName As String
sheetName = "Sheet2"
Range("A2").Formula = "=INDIRECT(""" & sheetName & "!A1"")"
End Sub
🚨 Note: Using INDIRECT can slow down workbook performance, especially with large datasets.
5. Copy and Paste Data Between Sheets

This method is useful for linking by directly transferring data:
- Copy/Paste Data: You can automate copying data from one sheet to another.
- VBA Copy/Paste: “`vba Sub CopyData() Dim sourceSheet As Worksheet Dim destSheet As Worksheet
Set sourceSheet = ThisWorkbook.Sheets("SourceSheet")
Set destSheet = ThisWorkbook.Sheets("DestinationSheet")
sourceSheet.Range("A1:B10").Copy Destination:=destSheet.Range("A1")
End Sub
```
Each of these methods has its own use cases, making linking sheets in Excel VBA a versatile task tailored to your specific needs. Whether you're aiming for a simple reference or complex dynamic linking, Excel VBA provides the tools to make your data manipulation more dynamic and efficient.
Can I link cells between different Excel workbooks using VBA?
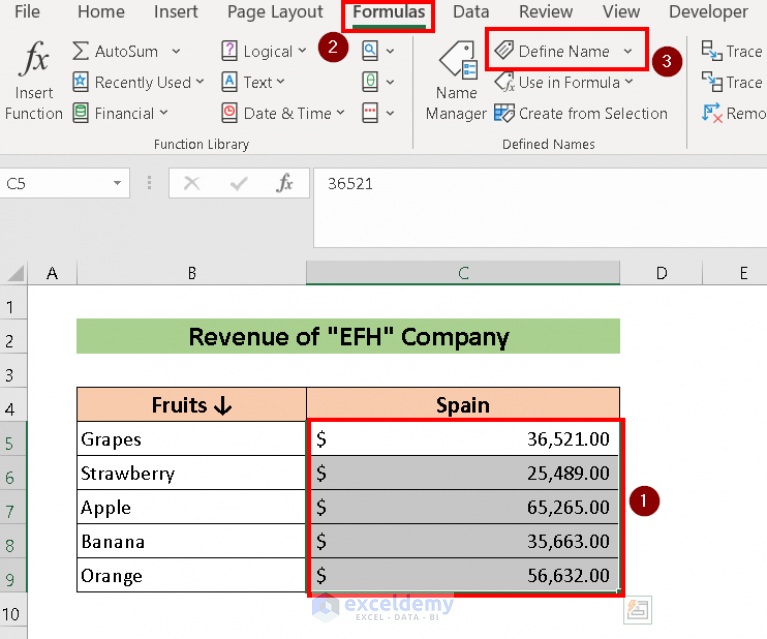
+
Yes, you can link cells between different workbooks using VBA by referencing the workbook and its sheet in the formula or by using dynamic linking methods like INDIRECT. Remember to keep both workbooks open for seamless operation.
What happens if I change the name of a sheet after creating a link with VBA?

+
Excel VBA links created with direct references (e.g., “=Sheet1!A1”) will break if the sheet name changes. Using named ranges or INDIRECT can help mitigate this issue by allowing dynamic references.
How can I make my links dynamic to handle sheet or workbook name changes?

+
Use the INDIRECT function in combination with a cell that holds the sheet or workbook name. This way, even if the names change, your links will still reference the correct location as long as the cell values are updated accordingly.