Create Excel Sheets in Python: Easy Guide
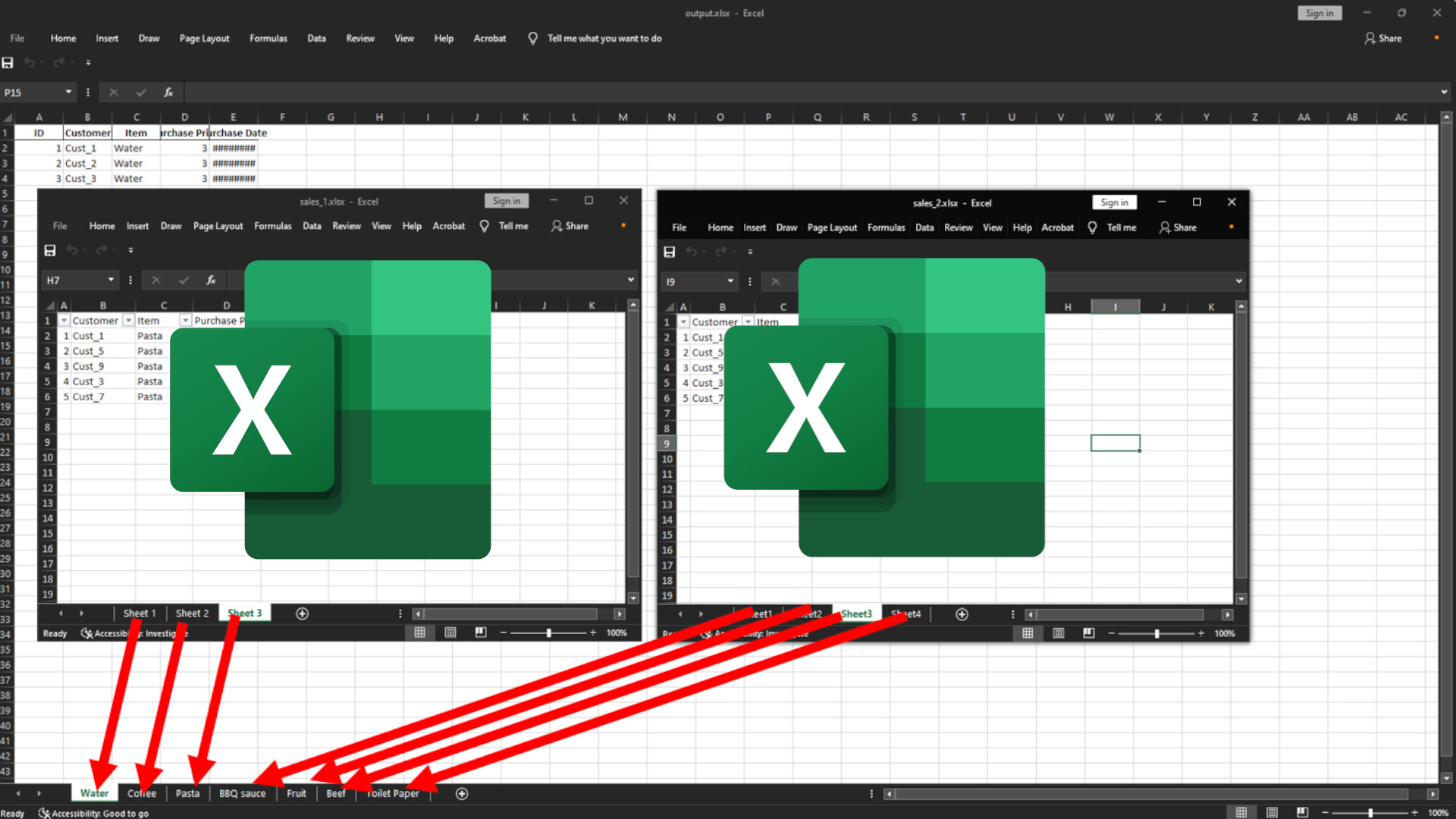
Unlocking the power of Python to create Excel spreadsheets can streamline your data handling tasks, making them more efficient and automated. This guide will walk you through the process of using Python libraries to generate, manipulate, and save Excel files, offering you the flexibility to tailor your spreadsheets to your needs.
What You Need to Know Before You Start

Before diving into the process, ensure you have the following:
- A basic understanding of Python programming.
- Python installed on your system.
- A way to install libraries like pip, the package manager for Python.
Setting Up Your Python Environment

Begin by setting up your Python environment for Excel work:
- Install Python: Ensure you have Python installed on your machine. You can download it from the official Python website if you haven't already.
- Install the Openpyxl library: Open your command prompt or terminal and type:
pip install openpyxl
Creating a Basic Excel Spreadsheet
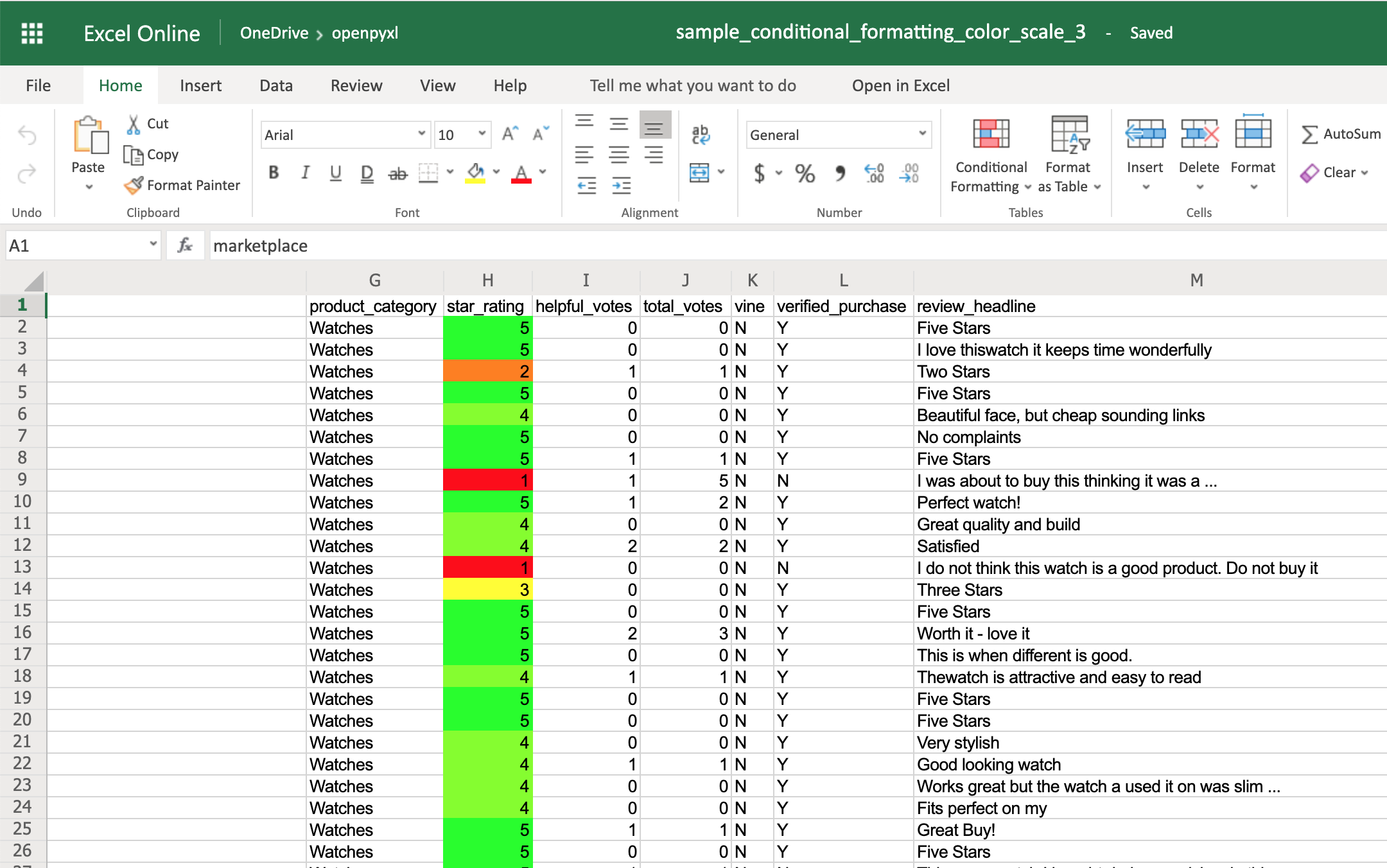
With your setup complete, you can now create a basic Excel sheet:
from openpyxl import Workbook
# Create a workbook
wb = Workbook()
# Get the active worksheet
ws = wb.active
# Add data to the sheet
ws['A1'] = "Hello"
ws['B1'] = "World!"
# Save the workbook
wb.save("helloworld.xlsx")
This script creates a workbook, adds some text to a sheet, and saves it as an Excel file named "helloworld.xlsx".
Adding More Complexity

Inserting Rows and Columns

To insert rows or columns into your Excel sheet:
from openpyxl import Workbook
# Create a workbook and get the active sheet
wb = Workbook()
ws = wb.active
# Insert a row at index 2
ws.insert_rows(2)
# Insert a column at index 2
ws.insert_cols(2)
# Add some data to check where the new row and column were inserted
ws['A1'] = "Header 1"
ws['B1'] = "Header 2"
ws['A2'] = "Row 2"
ws['C1'] = "Column C"
wb.save("complex_sheet.xlsx")
Formatting Cells

You can format cells in various ways, including adjusting font, color, or merging cells:
from openpyxl import Workbook
from openpyxl.styles import Font, Alignment, PatternFill
# Create a workbook and get the active sheet
wb = Workbook()
ws = wb.active
# Set cell values
ws['A1'] = "Welcome!"
ws['A2'] = "To Python and Excel!"
# Apply formatting
for cell in ws['A1:B2']:
for inner_cell in cell:
# Font styling
inner_cell.font = Font(bold=True, italic=True, size=14)
# Fill color
inner_cell.fill = PatternFill(fill_type='solid', start_color='00FF00')
# Merge cells
ws.merge_cells('A1:B1')
ws['A1'].alignment = Alignment(horizontal='center')
wb.save("formatted_sheet.xlsx")
Notes Section

๐ Note: The Openpyxl library handles Excel files (.xlsx) which are different from older formats like .xls. Ensure youโre working with .xlsx for compatibility.
๐ Note: When inserting rows or columns, remember that indices start at 1 for Excel, not 0 like in Python lists.
This guide provides just the basics of using Python with Excel, but there's much more you can do with libraries like openpyxl, such as working with formulas, handling charts, and more. Python offers powerful tools to automate and enhance your Excel-related work, making data manipulation tasks a breeze. The applications for this knowledge extend into fields like finance, project management, and data analysis, where efficiency in spreadsheet creation and manipulation is paramount.
What is the difference between .xlsx and .xls file formats?

+
.xlsx is the newer file format introduced with Microsoft Office 2007, which is more efficient and secure than the older .xls format.
Can I open Excel files created by Python in any spreadsheet software?

+
Yes, as long as the software supports the .xlsx format, you can open and edit the files created with Python.
Why do I need a library like Openpyxl?

+
Libraries like Openpyxl provide an easy-to-use interface to interact with Excel files, allowing automation and programming capabilities not available with manual Excel use.