5 VBA Tricks to Split Data into Multiple Excel Sheets

Are you struggling with large datasets in Excel and looking for an efficient way to manage them? If your answer is yes, then VBA (Visual Basic for Applications) can be your go-to solution. Here, we'll delve into 5 VBA tricks that will empower you to split your data into multiple Excel sheets effortlessly. This not only helps in organizing data better but also enhances productivity by making information more accessible.
Understanding VBA Macros for Data Management
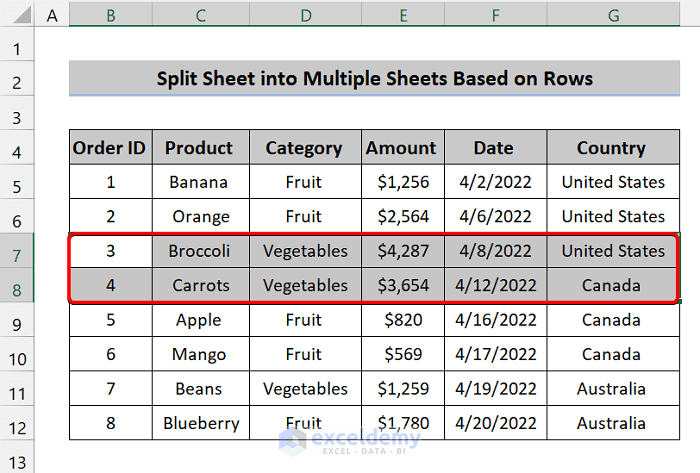
Before diving into the tricks, let’s briefly discuss what VBA macros are:
- VBA is a programming language built into Microsoft Office applications like Excel.
- Macros automate repetitive tasks, which is particularly useful for managing and splitting large datasets.
1. Splitting Data Using AutoFilter

One of the simplest methods to split data into multiple sheets is by using the AutoFilter feature. Here’s how you can do it:
Sub SplitData_AutoFilter()
Dim ws As Worksheet
Dim newWs As Worksheet
Dim filterRange As Range
Dim lRow As Long, lCol As Long
Dim c As Range
Set ws = ThisWorkbook.Sheets("Sheet1")
lRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
lCol = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column
Set filterRange = ws.Range("A1").CurrentRegion
For Each c In filterRange.Rows(1).Cells
filterRange.AutoFilter Field:=c.Column, Criteria1:=c.Value
If Not filterRange.SpecialCells(xlCellTypeVisible).Address = filterRange.Address Then
ws.Copy After:=Sheets(Sheets.Count)
Set newWs = ActiveSheet
newWs.Name = Left(c.Value, 31)
filterRange.SpecialCells(xlCellTypeVisible).Copy Destination:=newWs.Range("A1")
Application.DisplayAlerts = False
newWs.Cells.Delete Shift:=xlUp
Application.DisplayAlerts = True
Application.CutCopyMode = False
End If
ws.AutoFilterMode = False
Next c
End Sub
⚠️ Note: Make sure to adjust the worksheet name, column letters, and row numbers according to your dataset.
2. Using Advanced Filter

Advanced Filter allows for more complex data splitting:
Sub SplitData_AdvancedFilter()
Dim ws As Worksheet
Dim listWs As Worksheet
Dim rng As Range
Dim critWs As Worksheet
Dim lastRow As Long
Dim keyCol As String
Set ws = ThisWorkbook.Sheets("Sheet1")
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Set rng = ws.Range("A1").CurrentRegion
keyCol = "B" 'Change to the column letter for your unique key.
'Create a new worksheet for unique keys
Set critWs = ThisWorkbook.Worksheets.Add(After:=ws)
critWs.Name = "Criteria"
rng.AdvancedFilter Action:=xlFilterCopy, CriteriaRange:=_, CopyToRange:=critWs.Range("A1"), Unique:=True
For Each row In critWs.UsedRange.Rows
If row.Row > 1 Then
'Create a new sheet for each unique key
Set listWs = ThisWorkbook.Sheets.Add(After:=Sheets(Sheets.Count))
listWs.Name = row.Cells(1).Value
'Filter data and copy to the new sheet
rng.AdvancedFilter Action:=xlFilterInPlace, CriteriaRange:=critWs.Range("A1:A2"), _
CopyToRange:=listWs.Range("A1"), Unique:=False
End If
Next row
Application.DisplayAlerts = False
critWs.Delete
ws.AutoFilterMode = False
Application.DisplayAlerts = True
End Sub
This macro works by first identifying unique keys, then filtering and copying the relevant data to new sheets.
3. Splitting Data Using Pivot Table

Pivot Tables can also be used for data segmentation:
Sub SplitData_PivotTable()
Dim ws As Worksheet, ptWs As Worksheet
Dim pc As PivotCache, pt As PivotTable
Dim fld As PivotField
Set ws = ThisWorkbook.Sheets("Sheet1")
Set ptWs = ThisWorkbook.Worksheets.Add(After:=ws)
'Set up the Pivot Cache
Set pc = ThisWorkbook.PivotCaches.Create(SourceType:=xlDatabase, SourceData:=ws.Range("A1").CurrentRegion)
'Create the Pivot Table
Set pt = pc.CreatePivotTable(TableDestination:=ptWs.Range("A3"), TableName:="SplitDataPivot")
With pt
.ManualUpdate = True
.SmallGrid = False
'Add Column Fields
.AddFields RowFields:="Category"
.ColumnGrand = True
.RowGrand = True
'Hide the Pivot Table to work in background
ptWs.Rows("2:2").Hidden = True
ptWs.Rows("1:1").EntireRow.Hidden = True
'Generate separate sheets
For Each fld In pt.RowFields
For Each pi In fld.PivotItems
pi.Visible = True
ws.Range("A1").CurrentRegion.Copy
With Worksheets.Add(After:=ThisWorkbook.Sheets(ThisWorkbook.Sheets.Count))
.Name = Left(pi.Value, 31)
.Range("A1").PasteSpecial Paste:=xlPasteValuesAndNumberFormats
End With
Next pi
Next fld
End With
End Sub
This method uses a Pivot Table to create sheets based on categories or other pivot fields.
4. Customizing Sheet Names

When splitting data, having meaningful sheet names can make navigation much easier:
Function CustomSheetName(rng As Range) As String
Dim cell As Range
Dim name As String
For Each cell In rng
If Len(Trim(cell.Value)) > 0 Then
If InStr(1, name, cell.Value, vbTextCompare) = 0 Then
If Len(name) + Len(cell.Value) > 31 Then Exit For
name = name & " " & cell.Value
End If
End If
Next cell
CustomSheetName = Replace(Trim(name), "/", "-")
End Function
This function allows you to concatenate cell values into a sheet name, ensuring it remains within Excel's 31-character limit.
5. Handling Complex Data Structures

For datasets with more intricate structures, VBA can still come to the rescue:
Sub ComplexSplitData()
Dim ws As Worksheet, newWs As Worksheet
Dim lastRow As Long, lastCol As Long, i As Long, j As Long
Dim keyCol As Long, header As Range
Set ws = ThisWorkbook.Sheets("Sheet1")
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
lastCol = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column
Set header = ws.Range("A1").Resize(1, lastCol)
'Cycle through rows
For i = 2 To lastRow
With ws
If .Cells(i, keyCol).Value <> .Cells(i - 1, keyCol).Value Then
.Copy After:=Sheets(Sheets.Count)
Set newWs = ActiveSheet
newWs.Name = .Cells(i, keyCol).Value
End If
'Copy and paste data
header.Copy Destination:=newWs.Range("A1")
.Rows(i).EntireRow.Copy Destination:=newWs.Range("A2")
End With
Next i
End Sub
This script accounts for different data structures by checking for unique values in the key column and creating new sheets accordingly.
In summary, these VBA tricks provide a powerful toolkit for splitting data in Excel. Whether you are dealing with simple or complex datasets, VBA can enhance your data management skills significantly. Each method has its unique advantages, allowing you to choose based on your specific needs, from the simplicity of AutoFilter to the sophisticated handling of multiple pivot fields. By implementing these techniques, you'll not only streamline your work process but also make your data more accessible and easier to analyze.
What is the benefit of splitting data into multiple Excel sheets?

+
Splitting data allows for easier analysis, reduces file sizes for better performance, and helps in organizing information based on categories or specific attributes.
Can these VBA methods be used for any dataset size?

+
Yes, these methods can handle any dataset size, though very large datasets might require optimization for speed and memory usage.
Do I need to have programming experience to use these VBA tricks?

+
While basic programming knowledge helps, the provided VBA code can be used with little to no modification, making it accessible for those new to VBA as well.
What should I do if the script runs too slow on large datasets?

+
Consider disabling screen updating with Application.ScreenUpdating = False
at the beginning of your macro and turning it back on at the end, or using techniques like loops instead of copying and pasting large blocks of data.