3 Ways to Open Excel Sheets with Python
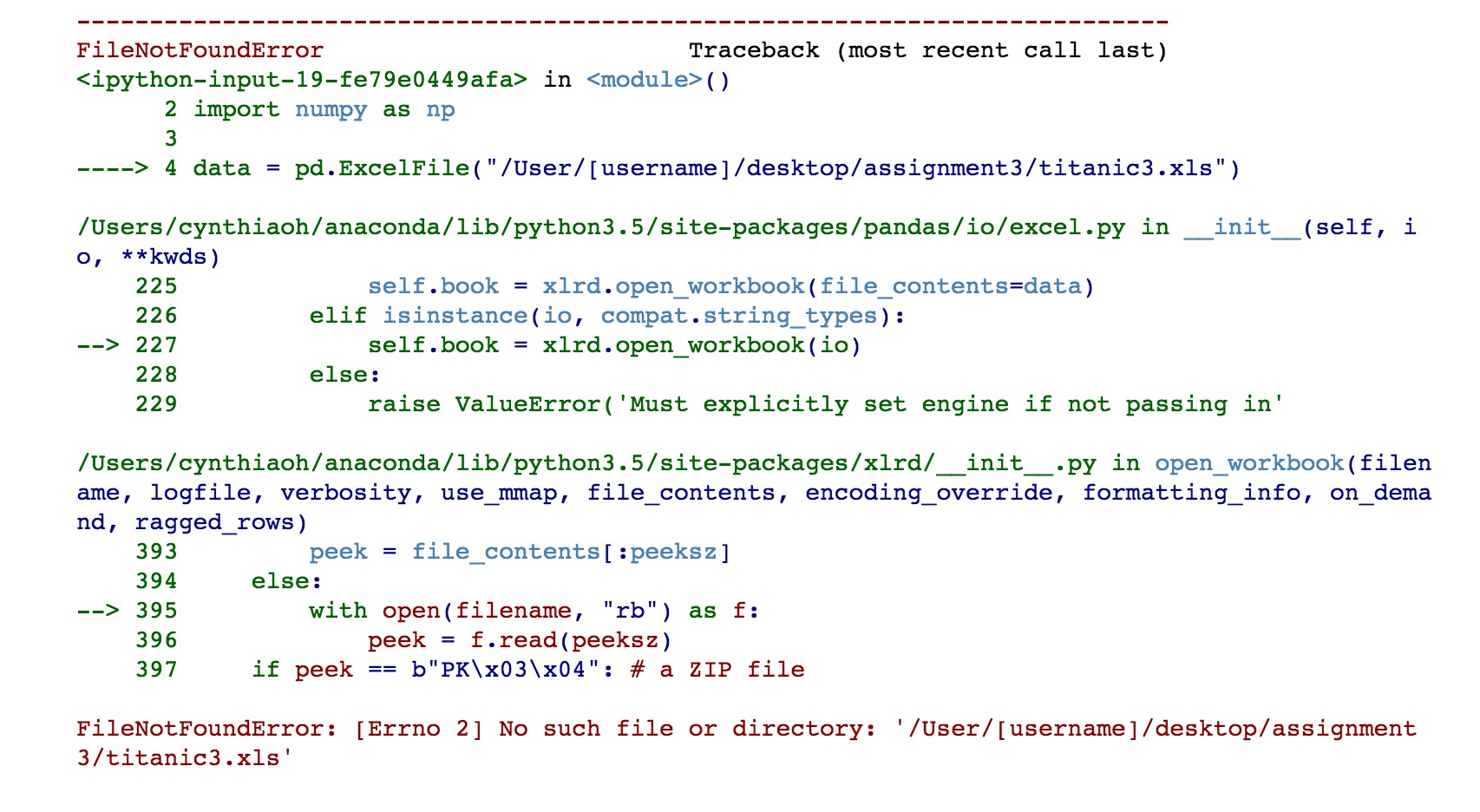
If you're looking to automate or enhance your Excel file interactions, Python offers robust libraries and methods to open, read, and manipulate Excel sheets. Let's explore three primary ways to achieve this, focusing on different libraries and their usage scenarios.
1. Using openpyxl
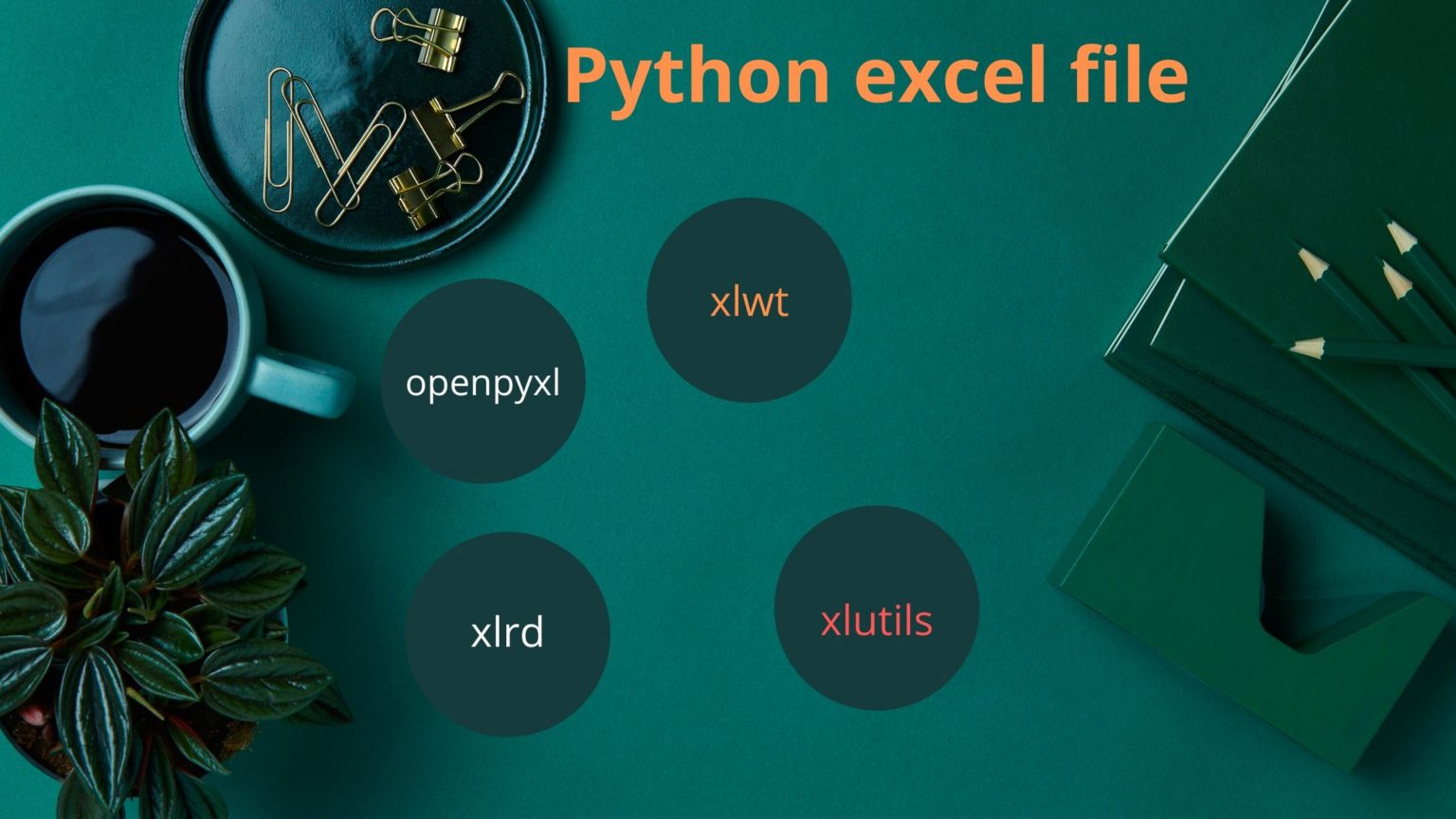
openpyxl is a popular Python library specifically designed to work with Excel 2010 xlsx/xlsm/xltx/xltm files. It allows for reading, writing, and modifying spreadsheets without the need to have Microsoft Excel installed.
- Installation: Install openpyxl via pip:
pip install openpyxl
from openpyxl import load_workbook
# Load workbook
wb = load_workbook('example.xlsx')
# Get sheet names
print(wb.sheetnames)
# Select the active sheet or any specific sheet
sheet = wb.active # or wb['Sheet1']
2. Using xlrd
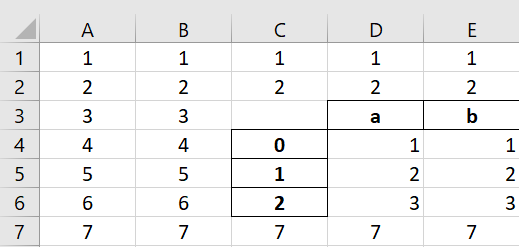
xlrd is another Python library that's effective for reading data from older Excel formats (like .xls) but also supports newer xlsx formats.
- Installation: Install xlrd through pip:
pip install xlrd
import xlrd
# Open the workbook
wb = xlrd.open_workbook('example.xls')
# Print sheet names
print(wb.sheet_names())
# Select sheet by index or name
sheet = wb.sheet_by_index(0) # or wb.sheet_by_name('Sheet1')
3. Using pandas
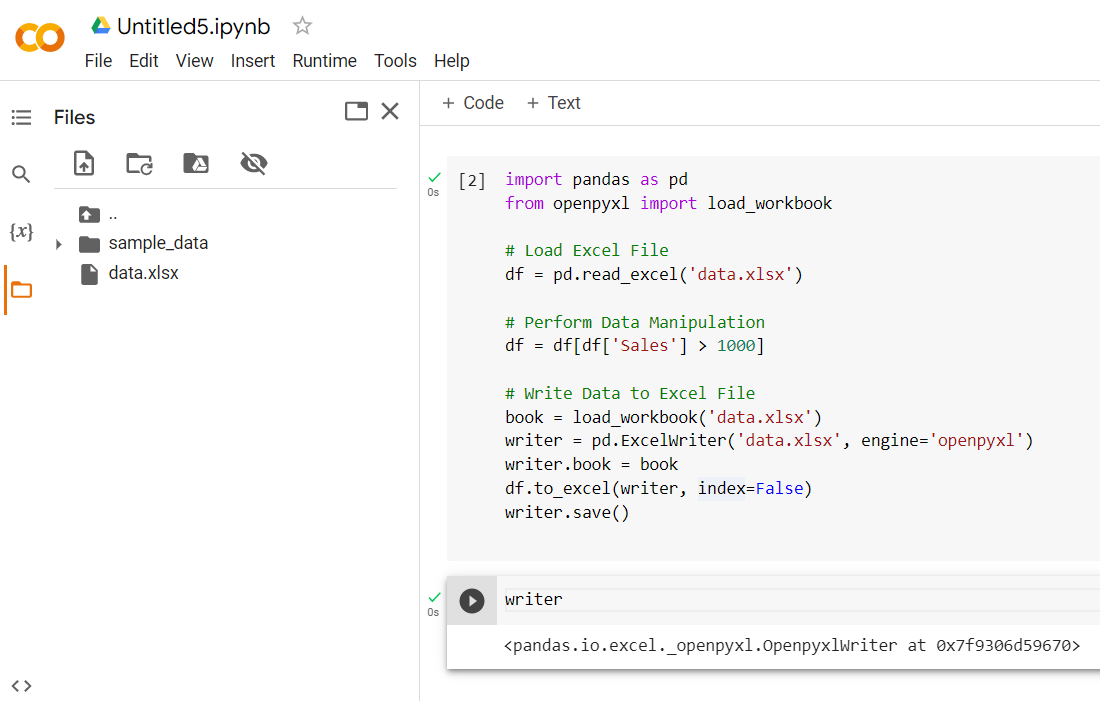
Pandas is not only for data manipulation but also excels in reading Excel files, offering simplicity and power for data analysts and scientists.
- Installation: Pandas can be installed with:
pip install pandas
import pandas as pd
# Read the Excel file
df = pd.read_excel('example.xlsx', sheet_name='Sheet1')
# View the data frame
print(df.head())
Each of these methods has its strengths and is best suited for different tasks:
- openpyxl - Best for creating or modifying spreadsheets, particularly when you need to preserve styling.
- xlrd - Efficient for reading large amounts of data from older Excel formats.
- pandas - Ideal for data analysis where Excel files serve as data sources.
📝 Note: If you're dealing with complex Excel features like pivot tables or charts, consider using additional libraries or looking into third-party solutions.
Whether you're automating report generation, data extraction, or simply opening and reading spreadsheets, Python provides versatile tools tailored for different needs. By understanding these libraries and their specific use cases, you can choose the best method for your project, ensuring efficiency and accuracy in your work with Excel files.
Which library should I use if I’m working with Excel files for data analysis?
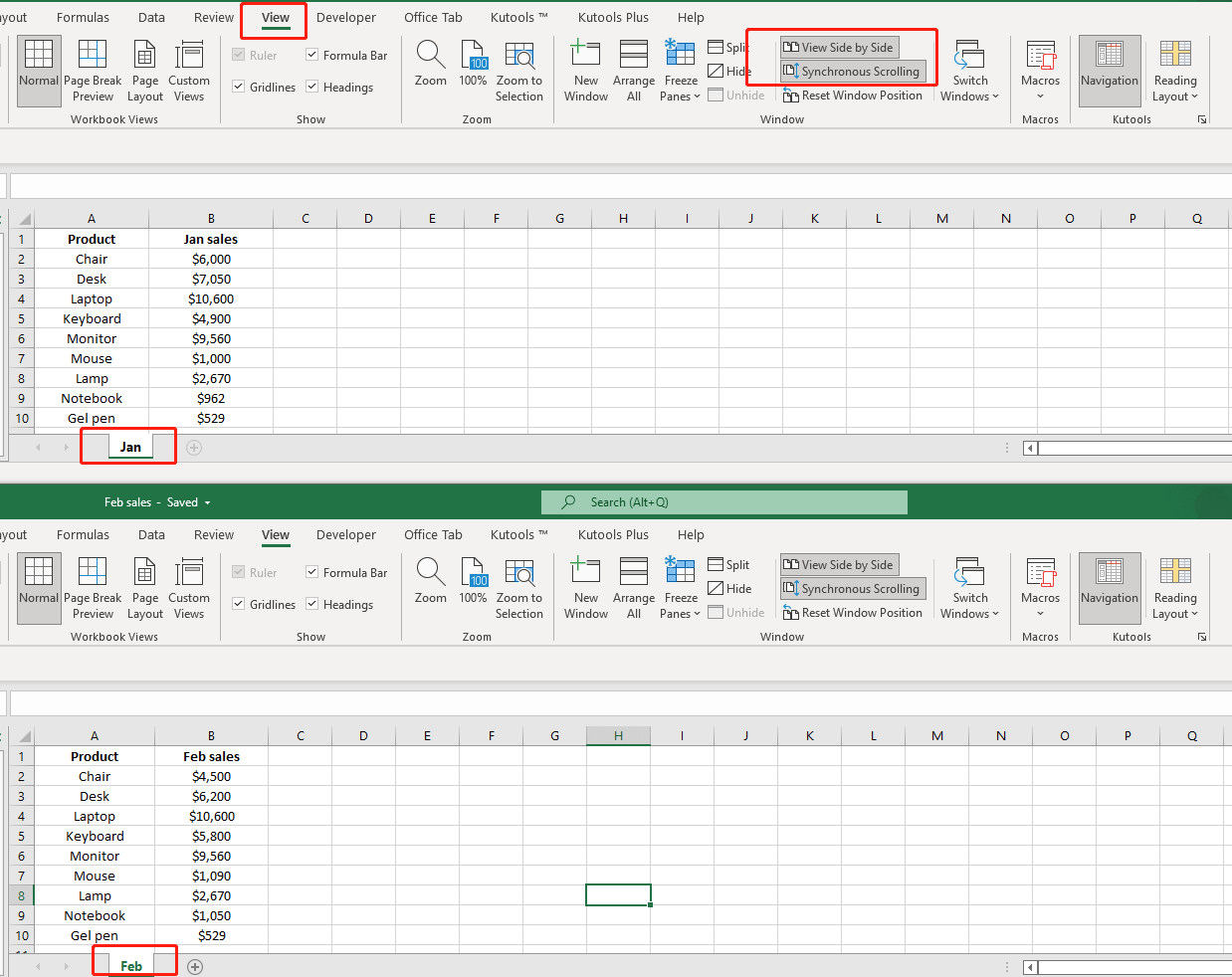
+
For data analysis, pandas is highly recommended due to its robust data manipulation capabilities. It can easily import Excel sheets into DataFrames, which are perfect for further analysis and transformation.
Can I use these libraries to modify Excel files?
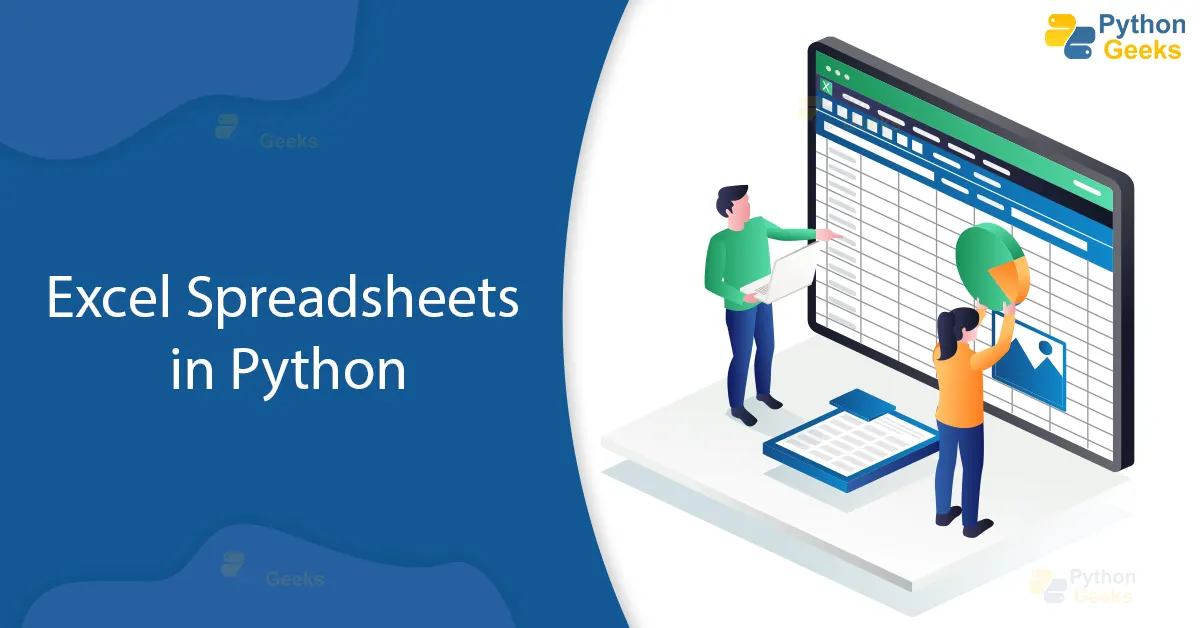
+
Yes, both openpyxl and pandas can write and modify Excel files. openpyxl provides more options for cell-specific modifications, whereas pandas focuses on bulk data operations.
Are there any limitations to these libraries when working with Excel?
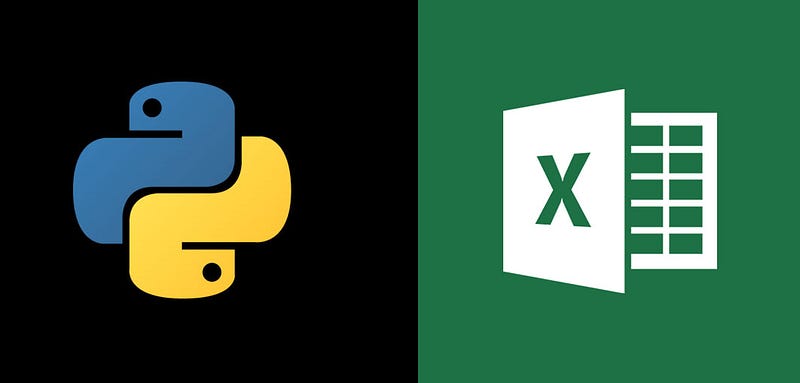
+
Yes, limitations include:
- Limited support for macros, especially complex ones.
- Potential issues with compatibility when dealing with Microsoft Excel features not replicated in the libraries.
- xlrd lacks support for newer Excel formats by default, requiring an additional library like openpyxl for xlsx files.