Automatically Name Excel Sheets: Simple Guide

Excel spreadsheets often manage extensive datasets, requiring a structured approach to organization. One effective strategy to streamline data management is automatically naming Excel sheets. This guide will explore why and how to automate sheet naming, enhancing productivity and efficiency.
Why Automate Sheet Naming?

- Efficiency: Saves time by eliminating the need for manual input of sheet names.
- Consistency: Reduces errors by maintaining uniform naming conventions across multiple sheets.
- Scalability: Easily manage larger datasets or workbooks with numerous sheets.
- Accessibility: Enhances navigation through a well-organized workbook.
How to Automate Sheet Naming with VBA

Visual Basic for Applications (VBA) is a powerful tool within Excel that enables automation. Here’s how to set up automatic sheet naming:
Enable Developer Tab

Before diving into VBA, ensure the Developer tab is visible in your Excel:
- Go to File > Options.
- Click on Customize Ribbon.
- In the list of Main Tabs, check the Developer box.
- Click OK to display the Developer tab.
Create VBA Script

Now, let’s write the VBA code:
Press Alt + F11 to open the VBA Editor. In the Project Explorer, double-click on your workbook name, then insert a new module.
Sub AutoSheetName()
Dim ws As Worksheet
Dim i As Integer
i = 1
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> "Sheet1" Then ' Prevent changing the first sheet
ws.Name = "Sheet" & i
i = i + 1
End If
Next ws
End Sub
This script will rename every sheet in the workbook, starting with "Sheet1", sequentially as "Sheet2", "Sheet3", etc., except for the first sheet (if you wish to preserve it).
Run the Script

You can automate this script to run when you open your workbook:
- In the VBA Editor, double-click on “ThisWorkbook” in the Project Explorer.
- Paste the following code:
Private Sub Workbook_Open()
Call AutoSheetName
End Sub
This event will ensure the sheets are automatically named upon opening the workbook.
Important Considerations for VBA Automation
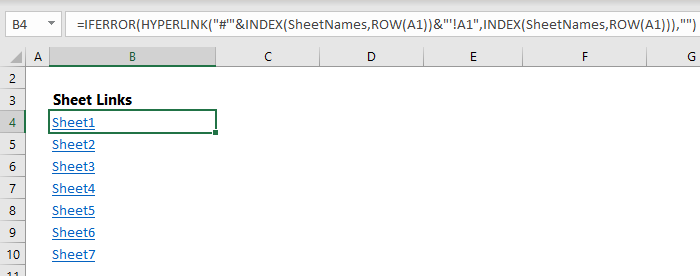
📌 Note: Ensure your VBA project is saved with a Macro-Enabled file format (like .xlsm or .xlsb) to maintain macro functionality.
Dealing with Existing Sheet Names

If your workbook already has named sheets, you might want to:
- Prompt the user before renaming.
- Only rename sheets that match a specific criteria (like those with “Sheet” in their names).
Automation Limitations

Be aware of:
- The maximum length for a sheet name is 31 characters.
- Avoid naming conflicts, which can disrupt Excel’s functionality.
After implementing these steps, your Excel sheets will have organized names, making navigation and data management more straightforward.
Automating Sheet Naming Beyond Basic VBA
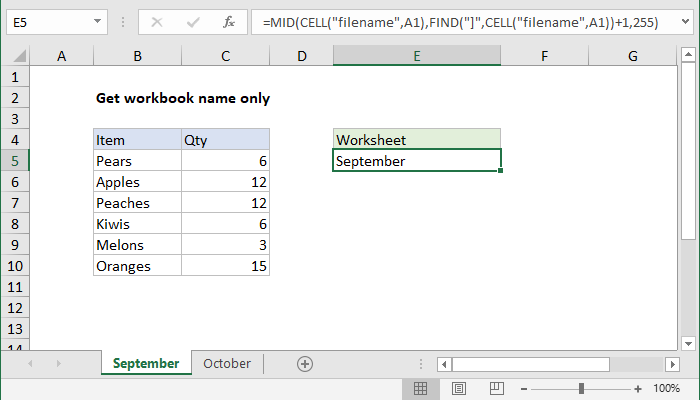
There are advanced techniques to further automate sheet naming:
Dynamic Naming Based on Cell Value

You can use cell values to dynamically name sheets:
Sub DynamicSheetName()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If Not IsEmpty(ws.Range("A1").Value) Then ' Check if cell A1 is not empty
ws.Name = Left(ws.Range("A1").Value, 31)
End If
Next ws
End Sub
This script looks at cell A1 of each sheet for its name. If A1 has a value, the sheet is named accordingly. If the name is longer than 31 characters, it truncates it to fit the limit.
Event-Driven Naming

Use event-driven procedures to rename sheets when specific actions occur:
Private Sub Workbook_SheetChange(ByVal Sh As Object, ByVal Target As Range)
If Target.Address = "$A$1" Then
If Not IsEmpty(Target.Value) Then
Sh.Name = Left(Target.Value, 31)
End If
End If
End Sub
This code renames the sheet when the value in cell A1 changes.
Automation in Excel through VBA not only simplifies organization but also enhances the efficiency and consistency of your data management. By following these steps, you'll be able to ensure your workbook is always neatly structured, promoting better teamwork and easier data access.
Can I revert Excel sheet names back to default?

+
Yes, you can write a VBA script to revert sheet names to their default, such as “Sheet1”, “Sheet2”, etc. However, ensure your backup or document your current sheet names before doing so.
What happens if I try to name sheets the same?

+
Excel won’t allow duplicate sheet names. The script should handle this by appending a number or using a different naming convention to make names unique.
Can I automate naming sheets based on external data?

+
Yes, you can use functions like VLOOKUP or external data connections to pull in data and dynamically name sheets based on that information.
How do I ensure sheet names are user-friendly?
+Use short, descriptive names. Avoid using special characters or spaces, and follow a naming convention that your team can easily understand and work with.
Is VBA the only way to automate sheet naming in Excel?
+While VBA is the most common method, Excel’s newer versions introduce features like Power Query and Power Automate, which can also manipulate sheet names under specific conditions.