Creating Multiple Excel Sheets in C: A Simple Guide

In the realm of Excel programming, one of the most sought-after functionalities is the ability to manipulate and create multiple sheets within a single workbook. This is particularly useful for developers working on data analysis projects, report generation, or any task requiring the organization of diverse data sets. In this guide, we'll dive deep into how to create multiple sheets in an Excel workbook using C, providing you with the foundational knowledge and the steps to achieve this.
Why Use C for Excel Sheet Creation?

Although there are numerous tools and languages available for Excel manipulation, C offers a unique blend of performance and control. It allows for:
- High Performance: Direct manipulation of Excel files can be done at a binary level, providing speed advantages.
- Flexibility: Custom control over Excel’s internals, which might not be accessible through other APIs.
- Legacy Systems: Compatibility with systems where more modern languages might not be supported.
Requirements for C Programming in Excel

To start with, you will need:
- A C Compiler: Ensure you have GCC or another C compiler installed.
- Excel Interop Library: While not directly part of Excel, you can interact through libraries like libxl or Excel4J.
- Understanding of Excel File Structure: Knowing how Excel stores data can be beneficial when manipulating files at a lower level.
Creating Multiple Sheets in C

Let’s walk through the process of creating a workbook with multiple sheets using C:
1. Setting Up the Project

First, we need to set up our development environment:
#include
#include “libxl.h” int main() { BookHandle book; SheetHandle sheet;
// Initialize the workbook book = xlCreateBook(); if(book) { // Your code here to create and manipulate sheets xlReleaseBook(book); return 0; } else { printf("Failed to create workbook.\n"); return 1; }
}
📌 Note: Make sure you have the libxl library correctly installed and linked in your project for this code to work.
2. Creating Sheets

With the workbook initialized, you can now create multiple sheets:
// Adding three sheets xlBookAddSheet(book, “Sheet1”, NULL); xlBookAddSheet(book, “Sheet2”, NULL); xlBookAddSheet(book, “Sheet3”, NULL);
// If you need to select one for operations: sheet = xlBookSheetByName(book, "Sheet1");
📌 Note: Always check if the sheet creation was successful before proceeding with data manipulation.
3. Writing Data to Sheets

After creating the sheets, you can write data to them:
// Assuming you’re writing to ‘Sheet1’
int col = 0, row = 0;
xlSheetWriteStr(sheet, row, col, “Hello from Sheet1!”, NULL);
4. Saving the Workbook
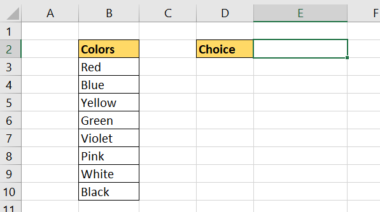
Finally, save the workbook to a file:
if(xlBookSave(book, “multiple_sheets.xlsx”) == 1) {
printf(“Workbook saved successfully.\n”);
} else {
printf(“Failed to save workbook.\n”);
}
Advanced Usage

For more complex scenarios, here are some additional considerations:
- Renaming Sheets: Sheets can be renamed after creation.
- Sheet Order: You can adjust the order in which sheets appear in the workbook.
- Formulas and Formatting: Apply Excel formulas, cell styles, and formatting to enhance data presentation.
Function | Description |
---|---|
xlSheetSetCol(sheet, 2, 10, 0, 0) | Set column width for column C to 10 characters |
xlSheetWriteFormula(sheet, 3, 0, "=A1+B1", NULL) | Write a formula at cell A4 |

In the final sections of this guide, we'll summarize the process and address some common questions developers have when manipulating Excel files with C.
This guide has explored the basics of creating multiple sheets within an Excel workbook using C. With the right tools and understanding of Excel's file structure, you can achieve efficient and tailored Excel file manipulation. The flexibility offered by working directly with binary files in C can significantly speed up data management tasks, and with the techniques outlined, you are now equipped to handle multiple sheets programmatically.
Can I open and modify existing Excel files with C?

+
Yes, using libraries like libxl, you can both read from and write to existing Excel files. However, ensure you have the necessary permissions to modify the files.
How can I handle errors when working with Excel files in C?

+
Most Excel manipulation libraries for C provide error handling functions or return codes that indicate success or failure. Always check these return values and implement proper error handling in your code.
Is there a performance benefit in using C for Excel operations compared to other languages?

+
Yes, C can be more performant, especially when dealing with large datasets, because it allows for direct binary file manipulation and does not incur the overhead of higher-level languages or runtimes.