Compare Excel Sheets Easily with Java Code

đź’ˇ Tip: To master this process, focus on understanding how data is organized and stored in Excel before diving into Java!
In the world of data analysis and management, comparing Excel sheets is a task that often presents significant challenges. Whether you’re looking to merge datasets, track changes, or synchronize information across different workbooks, manual comparison can be time-consuming and prone to human error. This is where leveraging the power of programming, specifically Java, can make a substantial difference. Java, with its robust libraries like Apache POI, provides a versatile solution for reading, manipulating, and comparing Excel files. Here’s a step-by-step guide on how to easily compare Excel sheets using Java:
Preparing Your Environment

To start, ensure your development environment is set up correctly:
- Install Java Development Kit (JDK): If not already installed, download and install the JDK from the official Oracle website.
- Setup Java IDE: Choose an Integrated Development Environment like Eclipse, IntelliJ IDEA, or NetBeans.
- Add Apache POI Library:
<dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>5.2.3</version> </dependency>
Use Maven or Gradle to include Apache POI in your project.
Basic Setup for Excel File Manipulation

Once your environment is set up, let’s begin with the basic steps to manipulate Excel files:
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelComparator {
public static void main(String[] args) {
try {
FileInputStream file1 = new FileInputStream("path_to_file1.xlsx");
FileInputStream file2 = new FileInputStream("path_to_file2.xlsx");
// Load both Excel workbooks
Workbook workbook1 = new XSSFWorkbook(file1);
Workbook workbook2 = new XSSFWorkbook(file2);
// Now we'll compare these workbooks
// Close streams
file1.close();
file2.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Here’s what each part does:
Workbook Loading:
XSSFWorkbook
is used to read.xlsx
files (Excel 2007 onwards). For older.xls
files, useHSSFWorkbook
.Stream Management: Always ensure to close your input streams after use to free up system resources.
📝 Note: Java uses try-catch for exception handling. Make sure to handle exceptions appropriately for production code.
Comparing Sheets and Their Content

Now let’s delve into comparing the content:
- Iterate Through Sheets: Ensure both workbooks have the same number of sheets.
int numSheets1 = workbook1.getNumberOfSheets();
int numSheets2 = workbook2.getNumberOfSheets();
if (numSheets1 != numSheets2) {
System.out.println("Number of sheets don't match!");
return;
}
- Compare Each Sheet:
for (int i = 0; i < numSheets1; i++) {
Sheet sheet1 = workbook1.getSheetAt(i);
Sheet sheet2 = workbook2.getSheetAt(i);
// Check sheet names for consistency
if (!sheet1.getSheetName().equals(sheet2.getSheetName())) {
System.out.println("Sheets at index " + i + " have different names");
}
// Here you would iterate through rows and cells to compare data
}
- Iterate Through Rows and Cells: This step involves comparing cell values, ensuring you’re looking at the same row and column indices:
for (int rowIndex = 0; rowIndex <= sheet1.getLastRowNum(); rowIndex++) {
Row row1 = sheet1.getRow(rowIndex);
Row row2 = sheet2.getRow(rowIndex);
if (row1 == null || row2 == null) {
System.out.println("Row mismatch at index " + rowIndex + " in sheet " + i);
continue;
}
// Iterate through cells in both rows
for (int cellIndex = 0; cellIndex < row1.getLastCellNum(); cellIndex++) {
Cell cell1 = row1.getCell(cellIndex);
Cell cell2 = row2.getCell(cellIndex);
if (cell1 == null && cell2 == null) {
continue; // Both cells are empty or non-existent
} else if (cell1 == null || cell2 == null) {
System.out.println("Cell mismatch at row " + rowIndex + ", cell " + cellIndex);
} else if (!cell1.getStringCellValue().equals(cell2.getStringCellValue())) {
System.out.println("Values differ at row " + rowIndex + ", cell " + cellIndex);
}
}
}
This comparison checks for cell values, but you might need to extend it for different cell types or formatting.
Handling Complex Comparisons

For advanced scenarios:
- Formulas and Comments: These might not be directly comparable as they change dynamically. Ensure to handle them separately.
- Data Types: Be mindful of how different data types (numbers, dates, booleans, etc.) are stored and compared in Excel.
- Conditional Formatting: This doesn’t affect data, but could be vital in some comparisons.
Integration with Reporting Tools

To enhance your comparison:
- Logging: Use Java logging frameworks like Log4j for detailed reporting.
- Report Generation: Consider using libraries like Apache PDFBox for creating PDF reports from comparison results.
Summary of Key Points

Automating Excel comparison with Java simplifies the process, making it less error-prone and more efficient:
- Preparation is key; setting up your environment correctly will save you time and headaches later on.
- Understand how data is stored in Excel and utilize Apache POI’s comprehensive API for interaction.
- Always ensure your code handles exceptions gracefully, especially when dealing with file operations.
- Use structured logging or report generation to make the output more useful and presentable.
This approach not only streamlines the comparison process but also opens up possibilities for more advanced data manipulation and automation tasks.
Why use Java for Excel sheet comparison?
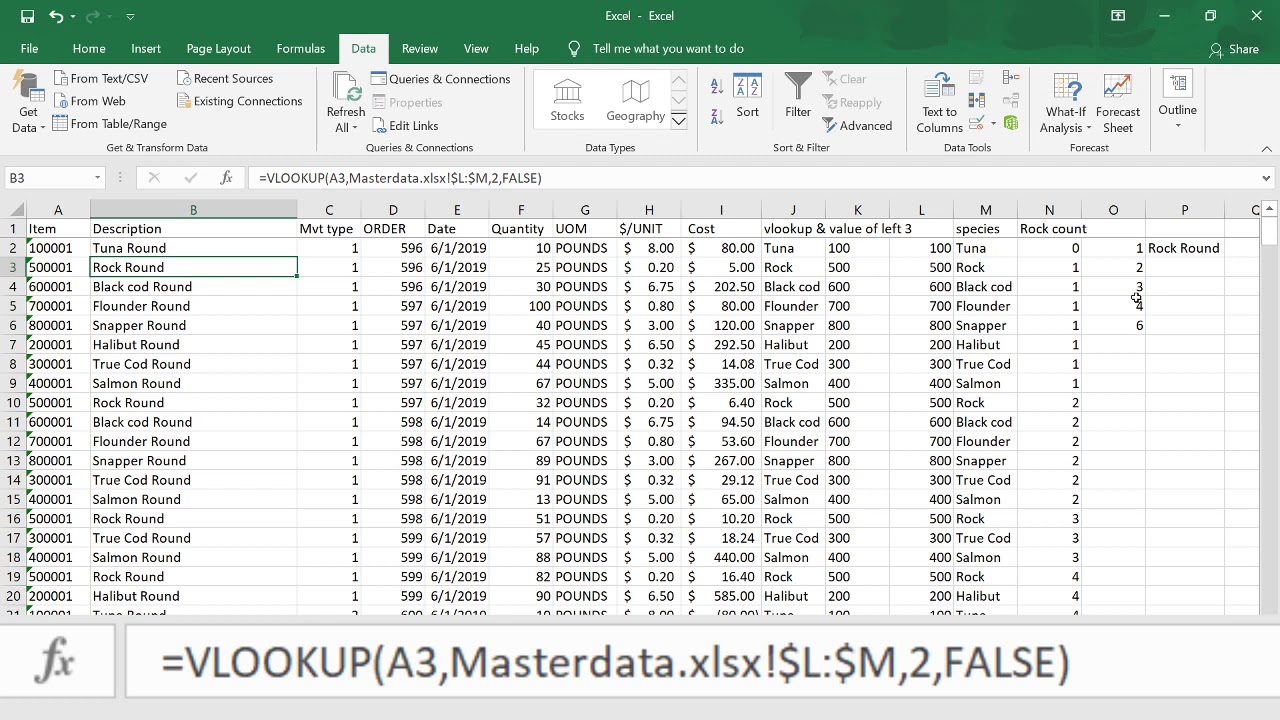
+
Java is platform-independent, has robust libraries like Apache POI for handling Excel files, and provides powerful capabilities for data manipulation, making it an ideal choice for automating complex Excel tasks.
Can I compare multiple sheets at once?

+
Yes, the example provided iterates through each sheet in the workbooks, comparing them one by one. Extend this to compare several sheets if needed.
How can I handle large Excel files?

+
For very large files, consider using SAX parsing with Apache POI’s event model to process Excel files in a more memory-efficient manner, avoiding loading the entire workbook into memory at once.