Comparing Excel Sheets with JavaScript: A Simple Guide

Why Compare Excel Sheets?

In today's data-driven world, Excel remains a cornerstone for businesses, researchers, and individuals to manage, analyze, and visualize data. But as data grows, so does the need for effective comparison techniques. Comparing Excel sheets can help in identifying discrepancies, syncing data, or merging changes made by multiple users or across different times. JavaScript, with its ubiquity and power in the browser, offers an accessible way to compare Excel files without specialized software.
Understanding JavaScript's Role

JavaScript's ability to manipulate DOM elements makes it perfect for creating dynamic web applications, including tools for Excel sheet comparison. By leveraging libraries such as Papa Parse or SheetJS, JavaScript can handle Excel file formats like .xls or .xlsx. This functionality extends to:
- Parsing Excel files into JavaScript objects
- Comparing and manipulating these objects
- Providing a visual representation of differences
Step-by-Step Guide to Compare Excel Sheets with JavaScript

1. Setting Up Your Environment

To get started, you'll need:
- A text editor or an Integrated Development Environment (IDE)
- A modern web browser with JavaScript support
- A library for Excel file parsing like Papa Parse or SheetJS
🔍 Note: You can add these libraries by including a script tag in your HTML or by using a module bundler.
2. Parsing Excel Files

We'll use the SheetJS library for this example:
const XLSX = require('xlsx');
// To parse an Excel file
const workbook = XLSX.readFile('yourfile.xlsx');
// Get the first worksheet
const worksheet = workbook.Sheets[workbook.SheetNames[0]];
// Convert worksheet to JSON
const jsonData = XLSX.utils.sheet_to_json(worksheet);
3. Comparing Sheets

After parsing the Excel sheets, compare them:
- Ensure both sheets have the same number of rows and columns
- Compare cell values one by one or use a function like:
function compareSheets(sheet1, sheet2) {
let differences = [];
sheet1.forEach((row1, index) => {
const row2 = sheet2[index];
for (let key in row1) {
if (row2[key] !== row1[key]) {
differences.push(`Row ${index + 1}, Column ${key}: ${row1[key]} != ${row2[key]}`);
}
}
});
return differences;
}
4. Displaying Differences

After identifying differences, you might want to display them:
const differences = compareSheets(jsonDataSheet1, jsonDataSheet2); // Add this HTML to your page dynamically or use DOM manipulation document.getElementById('differences').innerHTML = differences.map(d => `
${d}
`).join('');Best Practices for Comparison
- Normalize data: Ensure data types, formats, and casing are consistent
- Handle missing cells: Decide how to manage rows or columns with different lengths
- Optimize for performance: Large datasets require efficient algorithms
In wrapping up, comparing Excel sheets with JavaScript allows for flexible data management without relying on external tools. This approach not only leverages the power of JavaScript in data manipulation but also makes the process more accessible for users familiar with web technologies. Remember, the key is in the preparation of data and the careful handling of differences to provide a clear, actionable comparison result.
What libraries can I use to parse Excel files in JavaScript?
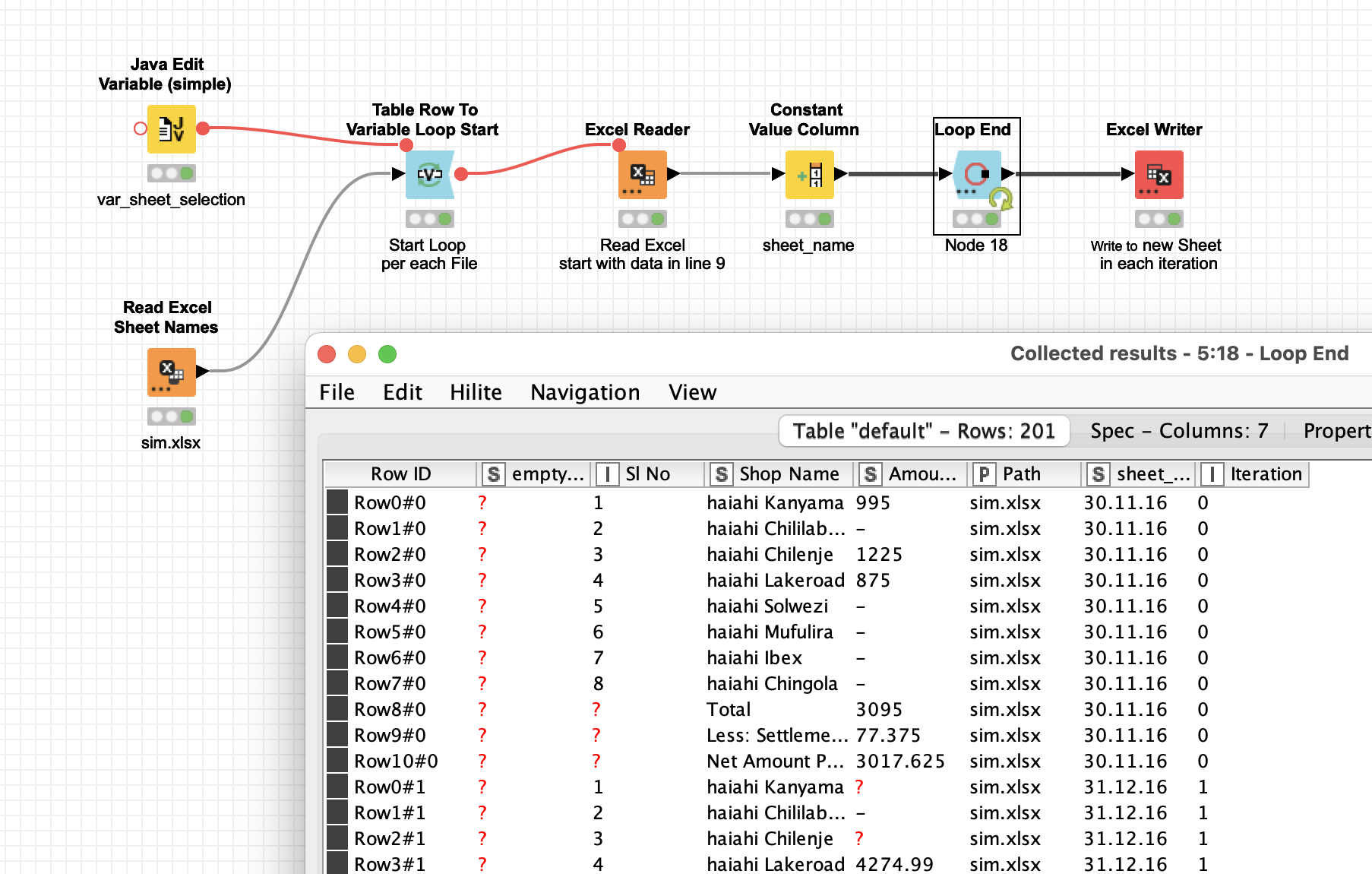
+
Popular libraries include Papa Parse, SheetJS, and XLSX for parsing Excel files in JavaScript.
How can JavaScript handle large Excel files for comparison?

+
To handle large files, consider using server-side processing, streaming data, or implementing efficient algorithms to manage the comparison process without loading the entire file into memory at once.
Can I automate Excel sheet comparison with JavaScript?

+
Yes, JavaScript can automate Excel comparisons by running scripts on a scheduled basis or as part of a workflow tool, making it efficient for regular data syncing or verification tasks.