5 Simple Ways to Check if an Excel Sheet Exists in Python

In the realm of data analysis, Python and Excel are a powerful duo, facilitating seamless data manipulation, analysis, and visualization. When working with Python, it's not uncommon to need to check whether a particular Excel sheet exists before performing operations like reading from, writing to, or modifying the sheet. This article explores five straightforward methods to verify if a specific worksheet exists in an Excel file using Python. These methods cater to different scenarios and Python libraries, ensuring that you have multiple options at your disposal.
Method 1: Using Openpyxl

Openpyxl is one of the most popular libraries for working with Excel files in Python. Here’s how you can check if a sheet exists with Openpyxl:
from openpyxl import load_workbook
# Load the workbook
workbook = load_workbook(filename='your_workbook.xlsx')
# Check if a sheet named "Sheet1" exists
sheet_name = "Sheet1"
if sheet_name in workbook.sheetnames:
print(f"The sheet '{sheet_name}' exists.")
else:
print(f"The sheet '{sheet_name}' does not exist.")
By loading the workbook and checking its sheetnames
attribute, you can easily verify the existence of a specific sheet.
Notes:

💡 Note: Openpyxl supports .xlsx, .xlsm, .xltx, and .xltm files. If you’re working with older Excel formats, consider converting them or using other libraries.
Method 2: Using Pandas

Pandas, while primarily known for data manipulation, can also interact with Excel files effectively. Here’s how you can check for sheet existence:
import pandas as pd
# Load the Excel file into a Pandas ExcelFile object
excel_file = pd.ExcelFile('your_workbook.xlsx')
# Check if a sheet named "Sheet1" exists
sheet_name = "Sheet1"
if sheet_name in excel_file.sheet_names:
print(f"The sheet '{sheet_name}' exists.")
else:
print(f"The sheet '{sheet_name}' does not exist.")
Pandas reads the Excel file into an ExcelFile
object, allowing you to inspect its sheet names without loading the entire workbook into memory.
Method 3: Using Pyexcel
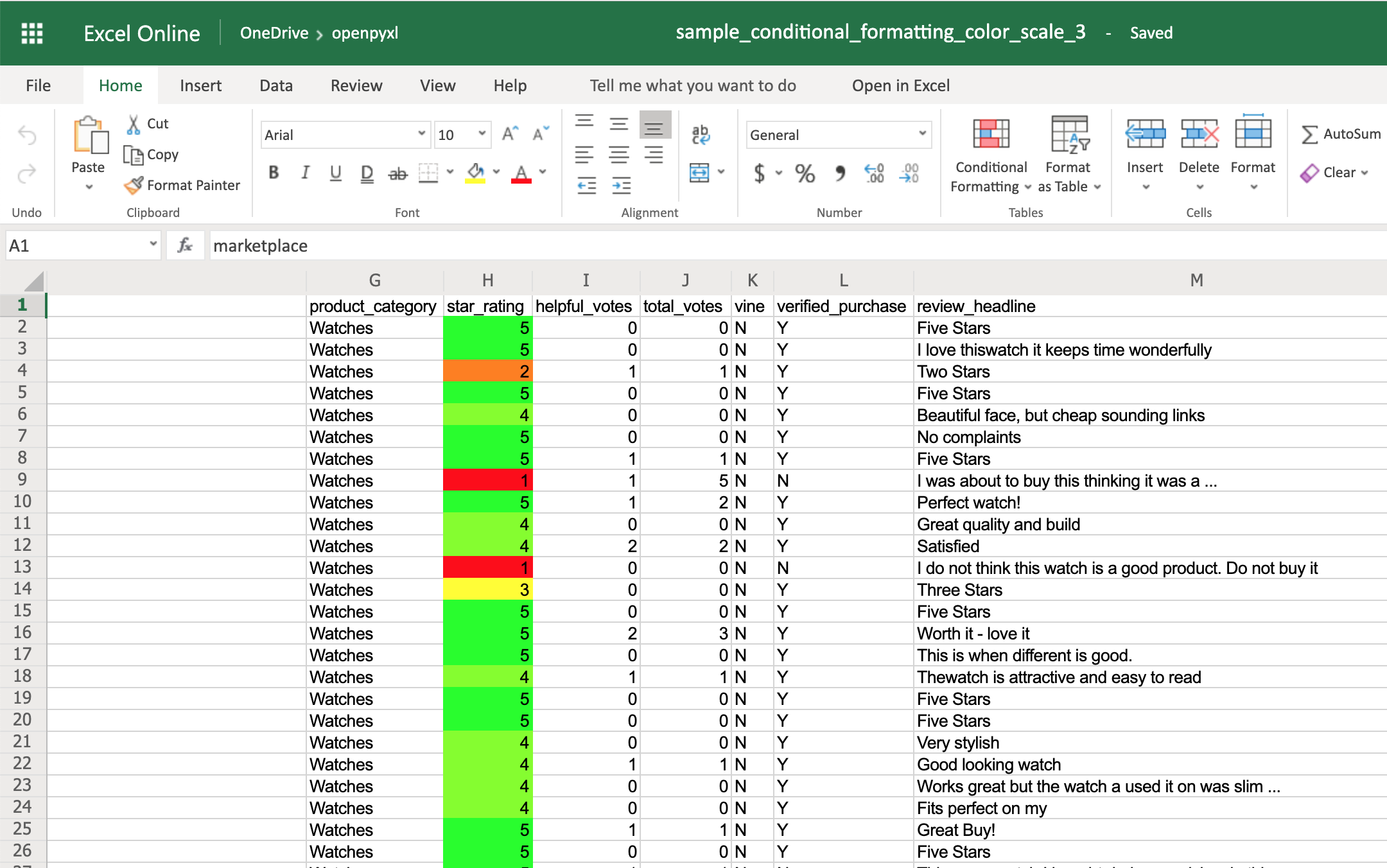
Pyexcel is another useful library for handling Excel files, offering a high-level API:
from pyexcel import get_book
# Load the workbook
workbook = get_book(file_name="your_workbook.xlsx")
# Check if a sheet named "Sheet1" exists
sheet_name = "Sheet1"
if sheet_name in workbook.sheet_names():
print(f"The sheet '{sheet_name}' exists.")
else:
print(f"The sheet '{sheet_name}' does not exist.")
This method uses Pyexcel's ability to work with multiple file formats, making it versatile for mixed environments.
Method 4: Using XLWing

XLWings provides a unique interface to Excel via Python, allowing for both automation and data manipulation:
import xlwings as xw
# Ensure Excel isn't open or this might fail
try:
wb = xw.Book('your_workbook.xlsx')
# Check if a sheet named "Sheet1" exists
sheet_name = "Sheet1"
if sheet_name in wb.sheets:
print(f"The sheet '{sheet_name}' exists.")
else:
print(f"The sheet '{sheet_name}' does not exist.")
finally:
xw.apps.active.quit()
Remember, using XLWings involves opening Excel, so ensure no interactive sessions are running or use error handling to manage exceptions gracefully.
Method 5: Direct File Reading

Sometimes, you might need a lightweight, library-independent solution:
import zipfile
# Excel files are essentially zip files
with zipfile.ZipFile("your_workbook.xlsx", "r") as z:
sheets = [name for name in z.namelist() if name.startswith('xl/worksheets/sheet')]
# Check if a sheet named "Sheet1" exists by its file name in the zip
sheet_name = "Sheet1"
if any(name.split('/')[-1] == f"sheet{sheet_name.replace(' ', '_')}.xml" for name in sheets):
print(f"The sheet '{sheet_name}' exists.")
else:
print(f"The sheet '{sheet_name}' does not exist.")
This method bypasses Excel-specific libraries and uses Python's built-in zipfile
module, focusing on the underlying structure of an Excel file.
Notes:

📝 Note: This method assumes standard naming conventions for sheets within the .xlsx file structure.
Each method above provides a different approach to checking if an Excel sheet exists, tailored to different needs and preferences. Whether you're looking for integration with a specific library, need to handle different file formats, or prefer a lightweight, library-agnostic method, there's an option for you. These methods not only help in data validation but also streamline your workflow by ensuring that subsequent operations on the Excel file will proceed without errors due to missing sheets.
The ability to verify the presence of a sheet in an Excel workbook is a fundamental skill when automating data tasks with Python. It not only enhances the robustness of your scripts but also allows for better error handling and user feedback. Python's rich ecosystem of libraries provides numerous ways to approach this problem, each with its strengths, making your data analysis journey more efficient and less prone to errors.
Can I use these methods to check for sheets in other spreadsheet formats?

+
Most of these methods are designed for .xlsx files. However, some libraries like Pyexcel or Openpyxl also support other formats like .ods (OpenDocument Spreadsheet) or .xls with some limitations or extra configurations.
Which method is best for real-time updates on an Excel file?

+
If you’re looking for real-time capabilities, XLWings would be your best choice as it can interact with Excel live. However, for file-based checks, Pandas or Openpyxl might be more efficient due to their quick file-reading capabilities.
How do I handle password-protected Excel files?
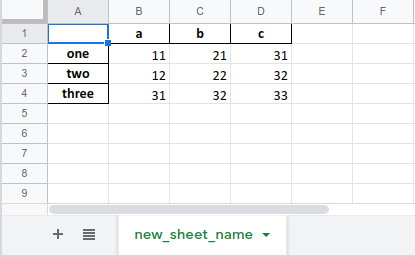
+
Not all libraries support password-protected files out of the box. For Openpyxl, you need to use additional libraries like msoffcrypto-tool
to decrypt the file before accessing it. For other libraries, similar third-party tools might be needed.