Change Active Excel Sheet with Macros Easily

When working with Excel, efficiency is key. One way to streamline your workflow and manage data effectively is by using macros to change active sheets within your workbook. Whether you're a seasoned Excel user or just getting started, understanding how to manipulate workbook sheets through VBA (Visual Basic for Applications) can save you a tremendous amount of time. This blog post will delve into how you can use macros for changing the active Excel sheet, provide step-by-step tutorials, and offer insights to enhance your productivity.
Why Use Macros to Change Active Sheets?

Macros are a powerful feature in Excel that allow for automation of repetitive tasks. Here are some reasons why you might want to use macros to manage active sheets:
- Automation: Automate the process of switching between sheets, reducing manual effort.
- Speed: Execute tasks faster than manual clicking.
- Consistency: Ensure uniform operations across different workbooks or sheets.
- Integration: Combine this action with other tasks to create complex workflows.
Step-by-Step Guide to Creating a Macro for Sheet Navigation

Here’s how you can create a simple macro to change the active sheet in Excel:
1. Open the Visual Basic Editor

- Press Alt + F11 on your keyboard to open the VBA editor.
2. Insert a New Module

- In the VBA editor, go to Insert > Module to add a new module where you’ll write your macro.
3. Write Your Macro

Now, you can write a simple macro. Here’s an example to change to Sheet2:
Sub ChangeToSheet2()
Worksheets(“Sheet2”).Activate
End Sub
4. Save and Run the Macro

- Save your macro with a name like ChangeToSheet2.
- Go back to Excel by pressing Alt + Q or clicking the “View Microsoft Excel” button in the editor.
- Run your macro by pressing Alt + F8, selecting your macro from the list, and clicking “Run”.
📝 Note: Make sure the sheet name you specify in your macro actually exists in the workbook. If it doesn't, Excel will throw an error.
Customizing the Macro

You might want to expand on this basic concept. Here are some ways to customize the macro:
1. Dynamic Sheet Selection

Instead of hardcoding the sheet name, allow the user to input it:
Sub DynamicSheetChange()
Dim sheetName As String
sheetName = InputBox(“Enter the sheet name to activate:”)
On Error Resume Next
Worksheets(sheetName).Activate
If Err.Number <> 0 Then MsgBox “Sheet not found!”
End Sub
⚠️ Note: The above macro uses an InputBox to get user input. It's crucial to handle potential errors, as shown with error handling.
2. Using Sheet Index

Sometimes, referencing sheets by index can be useful if their positions are known:
Sub ChangeSheetByIndex()
Sheets(2).Activate
End Sub
3. Cycling Through Sheets

For workflows where you need to cycle through sheets:
Sub CycleSheets()
With ThisWorkbook
If ActiveSheet.Index = .Sheets.Count Then
.Sheets(1).Activate
Else
.Sheets(ActiveSheet.Index + 1).Activate
End If
End With
End Sub
Integrating with Other Excel Features

Macros to change active sheets can be integrated with other Excel features like:
- Buttons: Assign a macro to a button on your sheet for easy access.
- Event Procedures: Trigger sheet changes based on events, like opening the workbook or on cell change.
- Form Controls: Use form controls like dropdowns or combo boxes to choose and navigate to different sheets.
🔎 Note: When integrating macros, be mindful of the security settings in Excel. Macros are disabled by default for workbooks from the internet unless you explicitly trust the publisher or reduce the security settings.
By now, you should have a good understanding of how to effectively use macros in Excel to manage and switch between active sheets. This skill not only helps in navigating large workbooks but also in automating data management tasks, creating custom user interfaces, and enhancing overall productivity.
Wrapping up, changing active sheets with macros in Excel offers numerous benefits like automation, speed, consistency, and integration with other features. By following the tutorials provided, you can start implementing these techniques in your daily work. Remember to keep security in mind, handle errors gracefully, and consider the specific needs of your workbook when designing your macros.
Can I use macros to change sheets in a protected workbook?
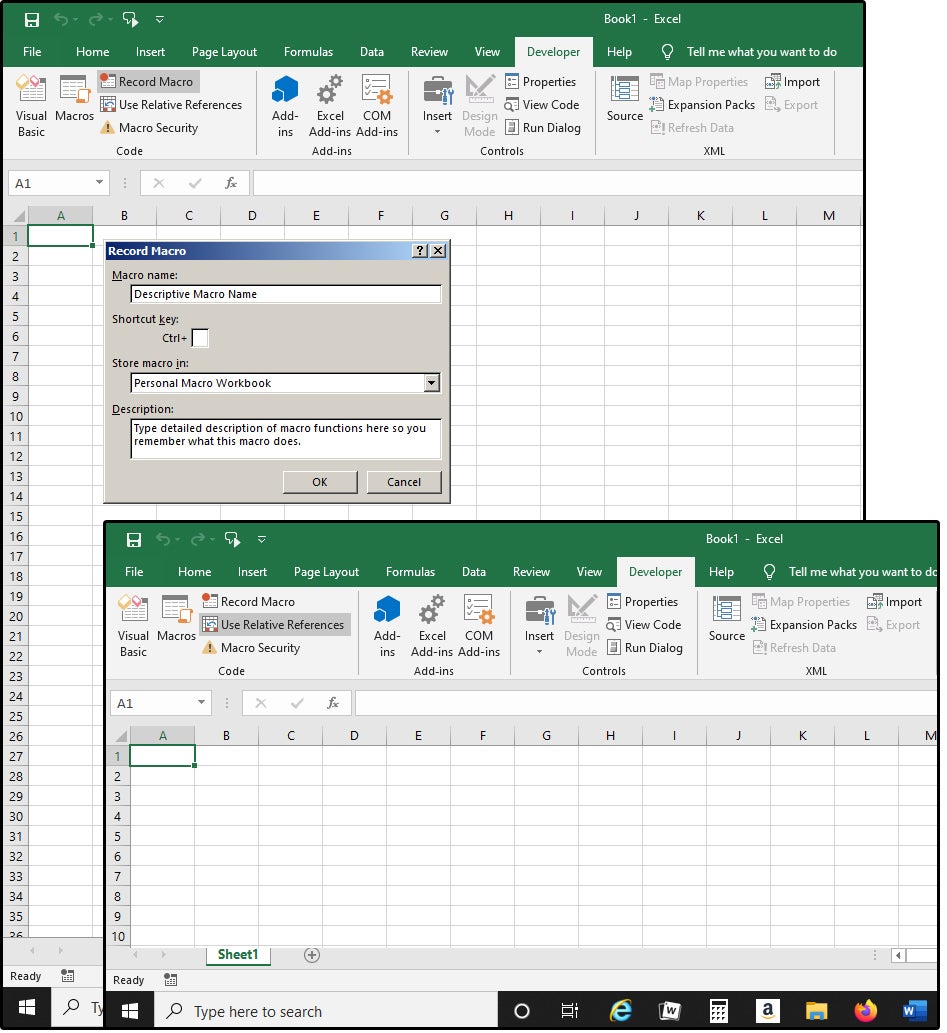
+
Yes, macros can change active sheets even in a protected workbook, provided the macro itself is not restricted by workbook protection settings. However, you must ensure that the macro has the necessary permissions to bypass protections or that the workbook is temporarily unprotected before running the macro.
Is it possible to navigate to a specific cell on a different sheet with a macro?

+
Absolutely. You can extend the macro to not only switch sheets but also select or focus on a particular cell:
Sub ChangeToSheetAndSelectCell()
Worksheets(“SheetName”).Activate
Range(“A1”).Select
End Sub
What if I want to run a macro based on cell value changes?
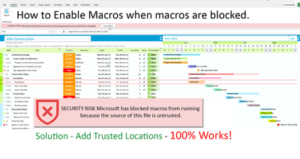
+
You can utilize the Worksheet_Change event in VBA to trigger macros when a cell value changes. Here’s how:
Private Sub Worksheet_Change(ByVal Target As Range)
If Not Intersect(Target, Me.Range(“A1”)) Is Nothing Then
‘ Your macro to change active sheet goes here
End If
End Sub