5 Ways to Rename Sheets in Excel VBA Easily

Mastering Excel VBA can significantly enhance your productivity when working with complex spreadsheets. A frequent task that many users look to automate is renaming sheets. In this post, we'll explore five straightforward and efficient ways to rename sheets in Excel VBA. By automating this process, you not only save time but also reduce the risk of errors that can occur with manual renames.
Why Rename Sheets Using VBA?

VBA (Visual Basic for Applications) is the programming language of Excel and other Microsoft Office applications. Here are some reasons why using VBA for renaming sheets is beneficial:
- Automation: Automate repetitive tasks to save time.
- Consistency: Ensures consistent naming conventions across your workbook.
- Accuracy: Reduces the likelihood of human error.
- Efficiency: Speed up operations, especially in large workbooks.
Method 1: Simple Rename

The simplest method involves using a single line of code:
ActiveWorkbook.Sheets(“Sheet1”).Name = “NewName”
This line of code will rename “Sheet1” to “NewName”. However, there are a few caveats:
- Sheet1 must already exist.
- NewName cannot already exist within the workbook.
⚠️ Note: If NewName already exists, VBA will throw an error. You might want to include error handling for a more robust solution.
Method 2: Rename by Sheet Index

If you prefer to work with sheet indexes instead of names:
ActiveSheet.Index = 3
ActiveSheet.Name = “NewName”
- This code first selects the third sheet in the workbook and then renames it.
- This method is useful if the sheet order is known but the names are not.
Method 3: Loop Through Sheets

To rename multiple sheets at once, you could use a loop:
Dim ws As Worksheet
For Each ws In ActiveWorkbook.Worksheets
If ws.Name = “OldName” Then ws.Name = “NewName”
Next ws
This method looks for sheets with the name “OldName” and renames them to “NewName”.
Method 4: Rename Using Input Box
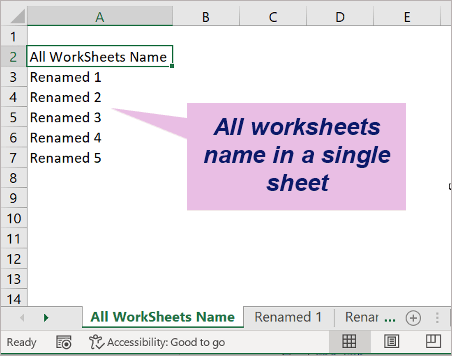
For interactive renaming where the user provides the new name:
Dim ws As Worksheet
Set ws = ActiveSheet
ws.Name = InputBox(“Enter new name for ” & ws.Name)
This code prompts the user to enter a new name for the currently active sheet.
Method 5: Rename Using a Range Value

If you have the new name listed somewhere in your workbook:
Dim ws As Worksheet
Dim cell As Range
Set ws = ActiveSheet
Set cell = ThisWorkbook.Sheets(“Names”).Range(“A1”)
ws.Name = cell.Value
- This method allows you to dynamically rename sheets based on cell values from another sheet.
These methods showcase the flexibility of VBA in handling different scenarios for renaming sheets. Each method has its use, depending on your specific requirements and how your workbook is structured.
In summary, renaming sheets in Excel using VBA is straightforward yet powerful. Whether you need to rename a single sheet or multiple sheets based on certain conditions or user inputs, VBA provides the tools you need to automate this process efficiently. Remember, the choice of method depends on your workflow, the complexity of your task, and how you prefer to interact with your spreadsheets. Automation through VBA not only reduces time spent on manual tasks but also brings consistency and reduces the potential for errors.
Can I use these VBA methods in any version of Excel?

+
Yes, these methods work in all modern versions of Excel, starting from Excel 2007 onwards.
What happens if I try to rename a sheet to a name that already exists?

+
Excel will throw an error if you attempt to rename a sheet to a name already in use. You’ll need to handle this error in VBA or ensure the name is unique before attempting the rename.
How do I ensure sheet names are unique when renaming dynamically?

+
Use a function to check if the name already exists. If it does, you can append a number or modify the name slightly to ensure it’s unique before renaming.
Can I automate sheet renaming with conditions like date or data within the sheet?

+
Absolutely, VBA can incorporate conditions or data-driven logic for renaming. You can write scripts to read values from cells, analyze dates, or check other criteria before renaming.