3 Ways to Unprotect an Excel Sheet with VBA Macros

How to Use VBA Macros to Unprotect an Excel Sheet

Excel spreadsheets are commonly used for organizing, analyzing, and storing data, but occasionally, you might find yourself locked out of a workbook or sheet due to forgotten passwords or inherited files with unknown protections. VBA (Visual Basic for Applications) can be a handy tool to help you regain access to these sheets. Here, we’ll explore three VBA macro methods to unprotect an Excel sheet.
Method 1: Using a Blank Password

In some instances, Excel sheets might have been protected with a blank or no password at all. This method exploits this possibility:
- Open Excel and press ALT + F11 to open the VBA editor.
- Choose Insert > Module to create a new module.
- Copy and paste the following code into the module:
Sub UnprotectSheetWithBlankPassword()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.ProtectContents Then
ws.Unprotect ""
End If
Next ws
End Sub
This VBA macro iterates through all the worksheets in your workbook and attempts to unprotect them using a blank password. Here's what the code does:
- Loops through each worksheet in the workbook.
- Checks if the worksheet is protected.
- Attempts to unprotect the sheet using an empty password string.
🔐 Note: If the sheet has no password or if it was protected with a blank password, this method will work.
Method 2: Brute Force Method

If the sheet is protected with a simple password, a brute-force attack might be feasible:
- Again, press ALT + F11 to open VBA editor and insert a new module.
- Copy and paste the following code into your module:
Sub UnprotectSheetBruteForce()
Dim ws As Worksheet
Dim pwd As String
Dim i As Long
For Each ws In ThisWorkbook.Worksheets
If ws.ProtectContents Then
For i = 65 To 90 ' ASCII codes for A-Z
pwd = Chr(i)
On Error Resume Next
ws.Unprotect pwd
If Err.Number = 0 Then
MsgBox "Sheet '" & ws.Name & "' unprotected with password: " & pwd, vbInformation
Exit Sub
End If
On Error GoTo 0
Next i
End If
Next ws
End Sub
This macro attempts all possible combinations of single characters (A to Z):
- Loops through each protected worksheet.
- Tries each letter from A to Z as a password.
- If the sheet is unprotected, it will display a message box with the correct password.
🚨 Note: This method can be time-consuming and may trigger security warnings.
Method 3: Dictionary Attack

If you suspect that the password might be a common word or phrase, a dictionary attack could be effective:
- In the VBA editor, insert a new module and enter the following code:
Sub UnprotectSheetDictionary()
Dim ws As Worksheet
Dim pwd As Variant
Dim dict As Variant
dict = Array("password", "excel", "office", "letmein", "test", "123456", "admin", "hello", "welcome", "security")
For Each ws In ThisWorkbook.Worksheets
If ws.ProtectContents Then
For Each pwd In dict
On Error Resume Next
ws.Unprotect pwd
If Err.Number = 0 Then
MsgBox "Sheet '" & ws.Name & "' unprotected with password: " & pwd, vbInformation
Exit Sub
End If
On Error GoTo 0
Next pwd
End If
Next ws
End Sub
This macro utilizes a predefined list of common passwords:
- Iterates through each protected worksheet.
- Tries each password from the array 'dict'.
- Displays the correct password in a message box if successful.
🔍 Note: Ensure to include a variety of passwords in the array. Customization might increase success rates.
In summary, using VBA macros to unprotect Excel sheets can be an effective solution for regaining access to your data. Whether employing a blank password check, a brute-force method, or a dictionary attack, these approaches provide a way around Excel’s protection mechanisms. However, it’s important to remember that these methods should only be used ethically and responsibly, respecting the intellectual property and privacy rights of others. Understanding and responsibly using Excel’s built-in security features can help prevent unauthorized access to sensitive data in the future.
Here are some key takeaways:
- Blank Password Check: A straightforward approach for sheets protected with an empty password.
- Brute Force Attack: Works when the password is simple and not too long, but can be slow and potentially flagged by security software.
- Dictionary Attack: Best for when you suspect a common word or phrase has been used.
Remember, if these methods fail, consider seeking professional help or using specialized software designed for this purpose. Always ensure you have the legal and moral right to access the content of a protected sheet.
Is it legal to unprotect an Excel sheet with VBA?
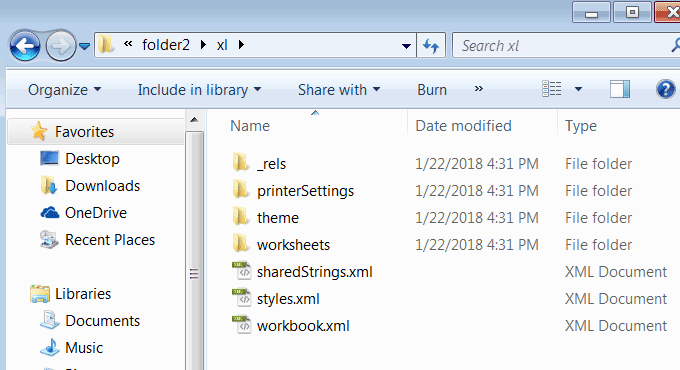
+
It’s legal if you have the rights to the data. Always consider ethical implications and ensure you have permission.
Can these methods bypass all types of Excel protection?

+
No, complex passwords, strong encryption, or password-protected workbooks might not be bypassed by these methods.
What precautions should I take before using VBA to unprotect sheets?

+
Backup your workbook to avoid data loss, and consider the security implications of altering Excel’s protection settings.