5 Ways to Keep Your Excel Sheet from Moving Unexpectedly

Automating Mouse Interactions with Python
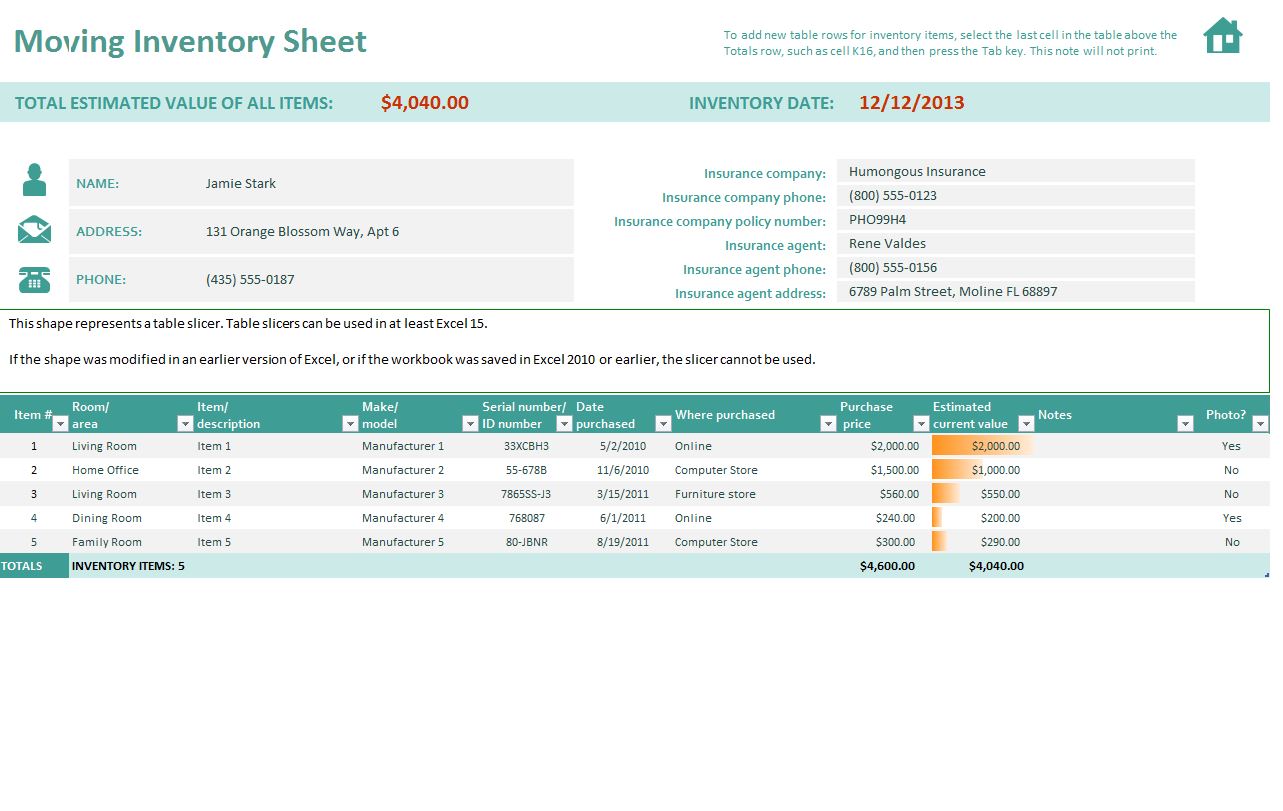
If you often find yourself trying to keep an Excel sheet from moving unexpectedly, there might be a solution in automating interactions with your mouse. Python, with libraries like pyautogui, can help automate repetitive tasks such as moving your mouse or clicking. Here are five ways you can prevent your Excel sheet from unexpected movements using Python:
1. Disable Window Focus

Windows applications can sometimes regain focus unexpectedly, causing an Excel sheet to move. By automating the process to disable window focus, you can keep your sheet stable:
- Install the required library: First, install pygetwindow with pip:
pip install pygetwindow
. - Run this Python script to lock focus on the Excel window:
import pyautogui import pygetwindow as gw
def disable_focus(): # Get the Excel window excel = gw.getWindowsWithTitle(“Excel”)[0] # Bring Excel to focus excel.activate()
disable_focus()
⚠️ Note: The window's title must match exactly; if your Excel sheet has a custom title, adjust it in the script.
2. Set Up Virtual Mouse Locks

Sometimes, you might inadvertently move the mouse, and your Excel sheet follows. Here’s how to set up virtual mouse locks:
- Import pyautogui: Make sure you have it installed.
- Write a script to restrict mouse movement:
import pyautogui
def lock_mouse(): x, y = pyautogui.position() pyautogui.moveTo(x, y, duration=0.2)
while True: lock_mouse()
3. Pause Screen Refresh

Excel sheets sometimes move due to automatic refreshing. Python can help by pausing this refresh:
- Create a function to pause screen refresh:
import win32com.client import time
def pause_refresh(excel): excel = win32com.client.Dispatch(“Excel.Application”) time.sleep(5) # Adjust as needed excel.ScreenUpdating = False
🕓 Note: Be careful not to stop screen updates indefinitely as this might impact Excel's performance.
4. Control Window Size and Placement

Enabling precise control over window size and placement can keep your sheet from moving:
- Set up window management:
import win32gui
def set_window_size(window_title, width, height, x, y): hwnd = win32gui.FindWindow(None, window_title) win32gui.SetWindowPos(hwnd, 0, x, y, width, height, 0)
5. Automatic Scroll Lock

Using Python to scroll to a specific cell can lock your sheet’s view:
- Activate Scroll Lock:
import pyautogui import win32com.client
def scroll_to(excel, sheet, cell): excel = win32com.client.Dispatch(“Excel.Application”) sheet = excel.Sheets(sheet) sheet.Activate() sheet.Range(cell).Activate()
scroll_to(“Excel”, “Sheet1”, ‘A1’)
By implementing these Python strategies, you can keep your Excel sheets from moving unexpectedly, enhancing your productivity and user experience. Automating these mouse interactions not only saves time but also ensures precision in your work, reducing frustration from unintended sheet movements.
Can these Python scripts run in the background?

+
Yes, by scheduling or running these scripts as background tasks, you can ensure they execute without requiring user interaction.
What if I need to use other applications during the task?

+
When working with other applications, ensure the scripts handle window focus gracefully or pause when necessary to avoid conflicts.
Are these methods safe?

+
These methods are generally safe, but always ensure that you test them in a non-critical environment first to understand their effects on your system.