VBA Tip: Quickly Switch Excel Sheets Like a Pro

Why Master Sheet Switching in Excel?
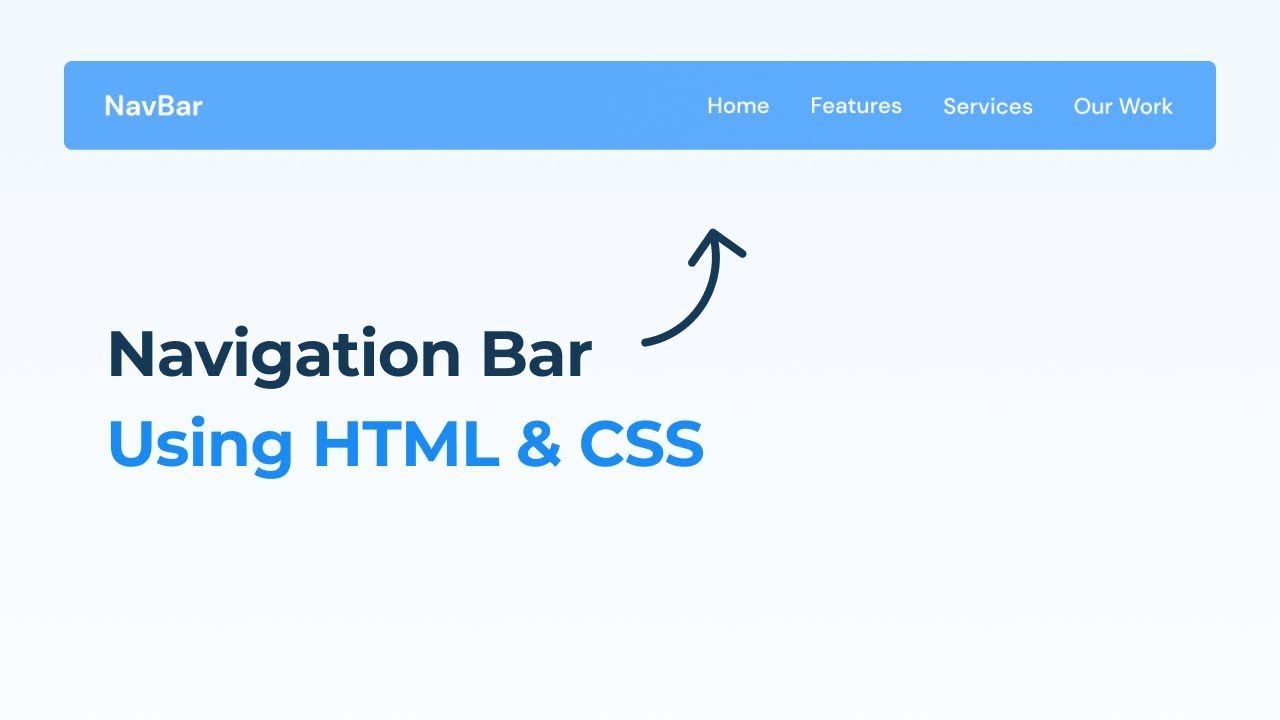
Switching between sheets in Excel can be a tedious task, especially if you’re dealing with numerous tabs or complex workbooks. By learning to swiftly navigate and manage sheets with VBA, you not only save time but also elevate your Excel skills to a professional level. Whether you’re an accountant, data analyst, or simply a frequent Excel user, mastering this skill can significantly streamline your workflow.
💡 Note: Remember, the goal is to enhance efficiency. Use VBA when manual switching is impractical or time-consuming.
Understanding the Workbook Object Model in VBA

The key to efficient sheet switching lies in understanding Excel’s object model in VBA:
- Workbook: This is the highest level object, representing an entire Excel file.
- Worksheet: An individual tab within the workbook.
- Range: A group of cells within a worksheet.
To interact with sheets, you first need to reference the workbook:
Dim wb as Workbook
Set wb = ThisWorkbook
Basic VBA Methods to Switch Sheets

Activating Sheets by Name

To switch to a specific sheet by its name:
wb.Sheets("SheetName").Activate
Activating Sheets by Index

For numerical indexing:
wb.Sheets(SheetIndexNumber).Activate
Keyboard Shortcuts

If you prefer keyboard shortcuts:
- Ctrl + PgUp: Move to the previous sheet
- Ctrl + PgDn: Move to the next sheet
Enhancing Sheet Navigation with VBA

To further streamline your Excel workflow, VBA can be used for:
- Looping through Sheets: Automate tasks across multiple sheets.
Dim sht As Worksheet
For Each sht In wb.Worksheets
'Code to be executed for each sheet
Next sht
- Custom User Forms: Create a form for easy navigation.
UserForm1.Show
🌐 Note: Creating custom user forms requires additional setup outside of these basic commands.
Advanced Techniques for Sheet Switching

Dynamic Sheet Navigation

Using variables or user input:
Dim SheetToActivate As String
SheetToActivate = InputBox("Enter sheet name to activate:")
On Error Resume Next
wb.Sheets(SheetToActivate).Activate
If Err.Number <> 0 Then
MsgBox "Sheet does not exist!"
Err.Clear
End If
Conditional Sheet Activation

To activate different sheets based on conditions:
If SomeCondition Then
wb.Sheets("Scenario A").Activate
Else
wb.Sheets("Scenario B").Activate
End If
Improving Efficiency

For large Excel files:
Application.ScreenUpdating = False
'Your code here
Application.ScreenUpdating = True
Integrating with Excel Functions

Combine VBA with Excel’s built-in functions:
- Index:
=INDEX(Sheet2!A1:A10, MATCH("Value", Sheet2!A1:A10, 0))
- Indirect:
=INDIRECT(SheetName!CellReference)
These functions enable dynamic referencing between sheets, enhancing VBA’s capabilities.
Keyboard Shortcuts for Power Users

While VBA provides automation, understanding keyboard shortcuts can further speed up your work:
- Ctrl + Home: Jumps to cell A1 of the active sheet.
- Alt + E, S, V: Quick paste special.
- Ctrl + Shift + 0: Toggle column outlines.
- F5: Opens the “Go To” dialog box.
Custom Shortcuts Using VBA

Create custom keyboard shortcuts:
Sub SetCustomShortcut()
Application.OnKey "^Shift+Tab", "MyCustomMacro"
End Sub
Sub MyCustomMacro()
'Your custom macro code here
End Sub
In summary, mastering the art of switching sheets in Excel through VBA not only enhances your productivity but also opens up a realm of possibilities for automation and personalization. Whether you’re creating financial models, managing data, or performing repetitive tasks, these techniques ensure you can navigate your Excel workbook with ease and efficiency.
Can VBA be used to switch sheets based on user input?

+
Yes, VBA allows you to create interactive elements where users can input sheet names, and VBA will navigate to the appropriate sheet using variables or input boxes.
What are the limitations of sheet switching in VBA?

+
VBA’s performance can slow down with very large workbooks, especially if the code involves looping through many sheets or using heavy Excel calculations. Additionally, activating sheets with VBA resets the active cell, which could disrupt user actions if not handled properly.
How can I make my VBA code more efficient?
+To improve VBA efficiency, consider turning off screen updating with Application.ScreenUpdating = False
, minimizing the use of Select
or Activate
commands, and structuring your code to minimize repeated actions or checks.