VBA: Quickly Rename Multiple Excel Sheets at Once

In today's data-driven world, Microsoft Excel remains a cornerstone tool for businesses, analysts, and individuals looking to manage and analyze data effectively. One common task that users often encounter involves managing multiple sheets within an Excel workbook, specifically renaming these sheets to reflect updated content or to organize them systematically. This post delves into how to leverage VBA (Visual Basic for Applications) to quickly rename multiple Excel sheets at once, enhancing your productivity by automating what can otherwise be a repetitive and time-consuming task.
Why Rename Excel Sheets?

- Organization: Renaming sheets can improve the clarity and organization of your workbook, making navigation easier.
- Updates: When your workbook’s content changes or evolves, updating sheet names can keep it current.
- Collaboration: Clearly named sheets facilitate collaboration, ensuring team members understand what each sheet contains without needing to open them.
Understanding VBA for Excel Sheet Renaming

VBA is Excel’s scripting language, allowing for automation of tasks through coding. Here’s how you can start:
- Open Excel: Navigate to Excel and open the workbook you wish to modify.
- Access the VBA Editor: Press
ALT + F11
to open the Visual Basic Editor. - Insert a Module: In the VBA Editor, right-click on any of the objects in the Project Explorer, go to “Insert”, then select “Module”.
Writing VBA Code to Rename Sheets

Let’s explore how to write VBA code to rename multiple Excel sheets:
Sub RenameMultipleSheets()
Dim ws As Worksheet
Dim newName As String
Dim i As Integer
i = 1
For Each ws In ThisWorkbook.Worksheets
newName = "Sheet" & i
ws.Name = newName
i = i + 1
Next ws
End Sub
💡 Note: The above code will rename all sheets sequentially starting from "Sheet1". You can customize the naming convention as per your requirement.
Customizing Sheet Names

If you want to rename sheets based on their current names or with specific prefixes/suffixes, you can adjust the VBA code:
Sub CustomRenameSheets()
Dim ws As Worksheet
Dim newName As String
Dim i As Integer
i = 1
For Each ws In ThisWorkbook.Worksheets
newName = "Data_" & i & "_" & Left(ws.Name, 5)
If Len(newName) > 31 Then
newName = Left(newName, 31)
End If
ws.Name = newName
i = i + 1
Next ws
End Sub
Renaming Sheets Based on Content

Sometimes, you might want to rename sheets based on specific content within the sheet itself, like:
Sub RenameByContent()
Dim ws As Worksheet
Dim cell As Range
Dim newName As String
For Each ws In ThisWorkbook.Worksheets
Set cell = ws.Range("A1")
If Not IsEmpty(cell) Then
newName = Replace(cell.Value, " ", "_")
If Len(newName) > 31 Then
newName = Left(newName, 31)
End If
ws.Name = newName
End If
Next ws
End Sub
💡 Note: Ensure the content in cell A1 of each sheet is what you want to use as the basis for the new sheet name. Adjust the cell reference or logic as needed.
Running Your VBA Script
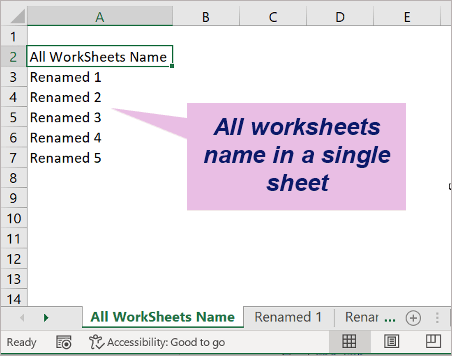
After writing your VBA script:
- Save the Workbook: Use “.xlsm” extension to enable macros.
- Run the Macro: Press
ALT + F8
to bring up the macro dialog, select your script, and click “Run”.
Things to Watch Out For
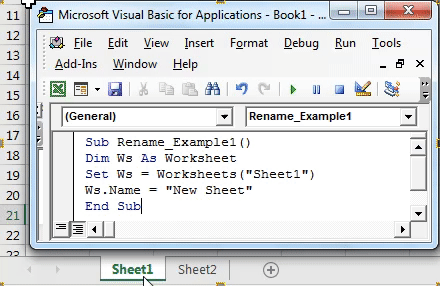
- Sheet name length is limited to 31 characters.
- Sheet names must be unique; VBA will throw an error if you try to duplicate names.
- Reserved characters like “:” or “/” cannot be used in sheet names.
🚨 Note: Be cautious when renaming sheets as VBA doesn't always give explicit feedback on failures, so make sure your naming logic is robust.
Summary of VBA Sheet Renaming

Automating the process of renaming multiple Excel sheets using VBA can significantly boost your workflow efficiency. By scripting your renaming tasks, you not only save time but also ensure consistency in sheet naming across your workbooks. Whether you’re renaming sheets for organization, updates, or collaboration, VBA provides a flexible, powerful tool to customize your Excel experience.
Can I undo changes made by VBA?

+
Yes, you can undo changes by closing the workbook without saving and reopening it, provided you haven’t saved since running the VBA script.
Can VBA rename sheets in different workbooks at the same time?

+
VBA can rename sheets within one workbook at a time. To rename sheets across workbooks, you would need to loop through and open each workbook to perform the task.
Is there a way to skip renaming a specific sheet?

+
Yes, you can add conditional checks in your VBA script to skip renaming sheets based on certain criteria, like checking the sheet’s name or its contents.