Excel VBA: How to Reference Sheets Easily

Mastering Microsoft Excel is a valuable skill, particularly when you delve into its programming language, VBA (Visual Basic for Applications). VBA allows users to automate tasks, create custom functions, and manage spreadsheets more efficiently. One of the fundamental skills in VBA programming involves working with sheet references. In this guide, we'll explore several methods to reference sheets in Excel VBA, ensuring you can navigate through your workbooks like a pro.
Direct Sheet Name Reference

Perhaps the most straightforward method to reference a sheet in VBA is by using its name directly:
Sheets(“Sheet1”).Activate
This command activates Sheet1. If you want to perform an action like selecting a range:
Sheets("Sheet1").Range("A1").Select
💡 Note: When using direct sheet names, ensure there are no spaces or special characters unless you use single quotes:
Sheets('Sheet 1').Activate
Sheet Index Number
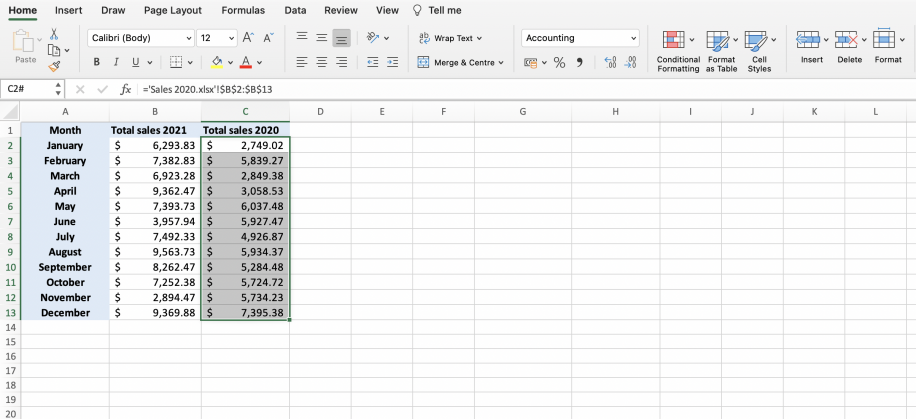
VBA also allows referencing sheets by their index number. In a workbook, sheets are indexed from 1 to the total number of sheets:
Sheets(1).Activate
This will activate the first sheet in the workbook. Here are some examples:
Sheets(2).Select
- Selects the second sheet.Sheets(Count).Select
- Selects the last sheet (useSheets.Count
to get the count).
📝 Note: Using index numbers can be risky as the order of sheets can change, potentially affecting your macro’s reliability.
Using CodeName

Each sheet in Excel has a CodeName property which doesn’t change even if you rename the sheet. You can view this in the VBA editor:
Sheet1.Activate
Here, 'Sheet1' refers to the CodeName of the sheet, not its visible name. This approach is especially useful when:
- The sheet's name might change but its purpose in your code remains the same.
- You want to avoid issues with sheet renaming or deleting.
However, accessing sheet properties or methods can be slightly different:
Debug.Print Sheet1.Name 'Prints the current visible name of the sheet
Workbook Sheets

If you're dealing with multiple workbooks, specifying the workbook can be necessary:
Workbooks("MyWorkbook.xlsx").Worksheets("Sheet1").Activate
This command will activate "Sheet1" in "MyWorkbook.xlsx". If your code is running in the context of the workbook where the sheet exists:
ThisWorkbook.Sheets("Sheet1").Activate
🔍 Note: Always verify if the workbook you're referencing is open, otherwise, your code will throw an error.
Dynamic Sheet References

For more versatile programming, you might need dynamic references. Here’s how you can:
- Use a variable for sheet names:
Dim sheetName as String
sheetName = “Sheet1”
Sheets(sheetName).Activate
Dim ws As Worksheet
For Each ws In Sheets
Debug.Print ws.Name ‘Prints each sheet name
Next ws
Dynamic references provide flexibility, particularly when dealing with an unknown number of sheets or sheets created on the fly:
Code Example | Description |
---|---|
Dim ws As Worksheet | Referencing a sheet in another open workbook. |
Dim ws As Worksheet | Activating all sheets in turn. |

🎯 Note: Dynamic references can help avoid hard-coding sheet names, improving code adaptability.
Conclusion

Referencing sheets in Excel VBA can be done through various methods, each suitable for different scenarios. Whether you’re using direct names, indices, code names, or workbook references, understanding these techniques can significantly enhance your VBA programming. These methods provide the versatility to manage sheets efficiently, automate tasks, and handle workbook complexity with ease. By incorporating these strategies into your VBA toolkit, you’ll be better equipped to manipulate Excel data programmatically, ensuring your macros are robust, readable, and easy to maintain.
What is the difference between “Sheets” and “Worksheets” in VBA?

+
“Sheets” refers to all types of sheets in the workbook, including chart sheets and other objects. “Worksheets” specifically refers to Excel spreadsheets.
Why should I use the CodeName property to reference sheets?

+
The CodeName property remains unchanged even if the sheet’s name is modified, providing a stable way to reference sheets in your code.
How can I check if a sheet exists before referencing it?

+
You can use the following code to check for the existence of a sheet:
On Error Resume Next
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets(“SheetName”)
If Not ws Is Nothing Then
‘Sheet exists
Else
‘Sheet does not exist
End If
On Error GoTo 0
Can I reference a sheet from another workbook?

+
Yes, by specifying the workbook name in your reference. For example:
Workbooks(“WorkbookName.xlsx”).Sheets(“SheetName”).Activate
How do I handle spaces or special characters in sheet names?

+
Use single quotes to enclose sheet names with spaces or special characters, like this:
Sheets(‘Sheet Name with Spaces’).Activate