5 Ways to Merge Excel Sheets with VBA Code

Mastering Excel is a vital skill for many professionals, and one common task is merging data from multiple sheets. While you might use manual methods or basic formulas, VBA (Visual Basic for Applications) provides a more efficient and automated approach. This blog post will explore five effective ways to merge Excel sheets using VBA code, each tailored for different scenarios.
1. Basic Merge Using Union Method
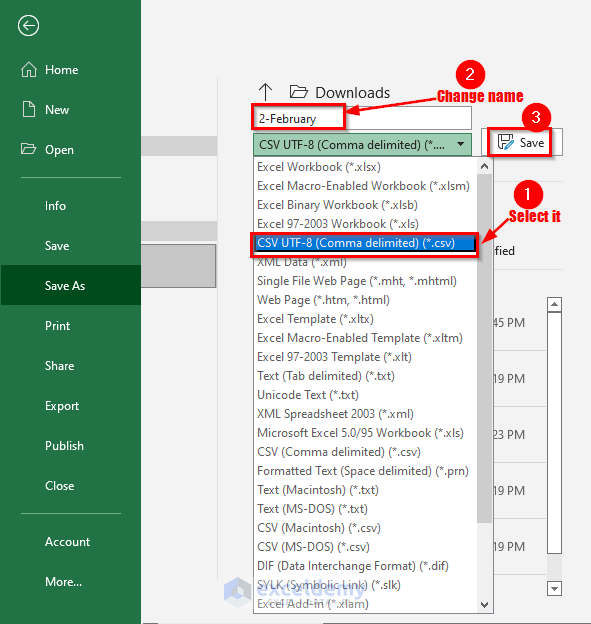
The simplest way to merge data from multiple sheets into one is through the Union method. Here's how you can do it:
- Open the Visual Basic Editor (Alt + F11).
- Insert a new module (Insert > Module).
- Copy and paste the following code:
```vba
Sub MergeSheets()
Dim ws As Worksheet
Dim baseWs As Worksheet
Dim lRow As Long
Dim lCol As Long
Set baseWs = ThisWorkbook.Worksheets("Sheet1") 'Define your destination sheet
'Start looping through the sheets
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> baseWs.Name Then 'Do not include the destination sheet
lRow = baseWs.Cells(baseWs.Rows.Count, "A").End(xlUp).Row + 1
ws.UsedRange.Copy baseWs.Cells(lRow, 1)
End If
Next ws
End Sub
```
Let's break down what this VBA code does:
- Define the Destination Sheet:
Set baseWs = ThisWorkbook.Worksheets("Sheet1")
assigns the worksheet where data will be merged. - Loop through Each Sheet: The
For Each
loop iterates over all sheets, copying the used range from each sheet to the bottom of the destination sheet.
⚠️ Note: This method might not handle sheets with different structures well, as it directly copies and pastes data.
2. Merging with Specific Criteria
If you need to merge data based on specific criteria, this more sophisticated approach might be needed:
- Insert a new module in VBA as before.
- Paste the following code:
```vba
Sub MergeWithCriteria()
Dim ws As Worksheet
Dim DestWs As Worksheet
Dim LastRow As Long
Dim lastCol As Long
Dim CriteriaColumn As Long
Set DestWs = ThisWorkbook.Worksheets("Destination")
CriteriaColumn = 5 'Column E for criteria check
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> DestWs.Name Then
For i = 1 To ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
If ws.Cells(i, CriteriaColumn).Value = "Specific Criteria" Then
LastRow = DestWs.Cells(DestWs.Rows.Count, "A").End(xlUp).Row + 1
ws.Rows(i).Copy Destination:=DestWs.Rows(LastRow)
End If
Next i
End If
Next ws
End Sub
```
Here's how this method works:
- Define Criteria: The criteria is set in
CriteriaColumn
where only rows matching "Specific Criteria" are copied. - Row by Row Check: Each row of every sheet is checked against the criteria, and if it matches, that row is copied to the destination sheet.
3. Append Data Horizontally
If your data needs to be combined side-by-side, use this method:
- Insert a new module in VBA.
- Copy and paste the following code:
```vba
Sub MergeSheetsHorizontal()
Dim ws As Worksheet
Dim DestWs As Worksheet
Dim StartCol As Long
Set DestWs = ThisWorkbook.Worksheets("Sheet1")
StartCol = 1 'Start from first column in destination sheet
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> DestWs.Name Then
DestWs.Cells(1, StartCol).EntireColumn.Insert
ws.UsedRange.Copy Destination:=DestWs.Cells(1, StartCol)
StartCol = DestWs.Cells(1, DestWs.Columns.Count).End(xlToLeft).Column + 1
End If
Next ws
End Sub
```
This VBA script will:
- Insert new columns for each sheet being merged.
- Copy the data from each sheet to the newly inserted columns.
4. Dynamic Merge with Error Handling
For a more robust solution where sheets might have different formats or errors:
- Insert a new module in VBA.
- Here's the code:
```vba
Sub MergeWithErrorHandling()
Dim ws As Worksheet
Dim DestWs As Worksheet
Dim LRow As Long, LCol As Long
Dim Source As Range, Dest As Range
On Error GoTo ErrorHandler
Set DestWs = ThisWorkbook.Worksheets("Sheet1")
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> DestWs.Name Then
Set Source = ws.UsedRange
LRow = DestWs.Cells(DestWs.Rows.Count, "A").End(xlUp).Row + 1
Set Dest = DestWs.Range(DestWs.Cells(LRow, 1), DestWs.Cells(LRow + Source.Rows.Count - 1, Source.Columns.Count))
Source.Copy Dest
End If
Next ws
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
```
This script:
- Uses error handling to manage potential issues during merging.
- Provides a cleaner exit if errors occur.
5. Merge Using Array Processing
For large datasets or where performance is critical, processing data in arrays can significantly speed up operations:
- Insert a new module in VBA.
- Use this code:
```vba
Sub FastMerge()
Dim ws As Worksheet
Dim DestWs As Worksheet
Dim destData() As Variant
Dim sourceData() As Variant
Dim i As Long, j As Long, nRow As Long
Set DestWs = ThisWorkbook.Worksheets("Sheet1")
For Each ws In ThisWorkbook.Worksheets
If ws.Name <> DestWs.Name Then
With ws
sourceData = .UsedRange.Value2
nRow = UBound(sourceData, 1)
For i = LBound(sourceData, 1) To nRow
nRow = DestWs.Cells(DestWs.Rows.Count, "A").End(xlUp).Row + 1
DestWs.Cells(nRow, 1).Resize(, UBound(sourceData, 2)).Value = Application.Index(sourceData, i, 0)
Next i
End With
End If
Next ws
End Sub
```
Key aspects:
- Data is read into an array for faster processing.
- Uses
Application.Index
to copy data from the source sheet to the destination sheet row by row.
After exploring these five ways to merge Excel sheets with VBA, you now have a variety of options at your disposal, each suited for different needs:
- Basic Union Merge for straightforward data consolidation.
- Merging with Criteria for selective merging.
- Horizontal Append for side-by-side data comparison.
- Error Handling Merge for error-prone environments.
- Array Processing for large datasets requiring high performance.
Each method enhances your ability to manage data across multiple sheets, increasing productivity and reducing the likelihood of errors. Keep in mind that VBA offers immense flexibility, allowing you to customize these solutions or create entirely new ones to fit your specific workflows.
Can I use these VBA scripts on any version of Excel?
+Yes, these VBA scripts should work in all modern versions of Excel that support VBA (from 2010 onwards), though some minor adjustments might be required for compatibility with older versions like Excel 2007 or below.
Is there a limit to the number of sheets I can merge?
+Excel’s limitation generally depends on system memory and worksheet size limits. Typically, you can handle merging numerous sheets if your computer has sufficient resources.
What should I do if my VBA script runs into errors?
+Implement error handling like in the “Merge with Error Handling” example. This will allow the script to continue running or inform you of issues, which you can then investigate and fix.
- Define Criteria: The criteria is set in
- Define the Destination Sheet: