Effortlessly Import Excel into JTable with Java

In the world of Java programming, working with Excel files and integrating them into a graphical user interface (GUI) application can be quite daunting for beginners. However, with the right tools and understanding, it can become a straightforward task. In this comprehensive guide, we will explore how to effortlessly import Excel data into a JTable using Java. We'll use the Apache POI library, which is widely recognized for manipulating Microsoft Office document formats, including Excel.
What You Need to Know Before Starting

- Java Development Kit (JDK): Ensure you have JDK installed on your machine.
- IDE: Use an Integrated Development Environment like Eclipse, IntelliJ IDEA, or NetBeans for coding convenience.
- Apache POI Library: Download or add this library through your build management tool (Maven or Gradle).
Setting Up Your Java Project

To begin, set up a new Java project in your preferred IDE:
- Create a new project.
- Add the Apache POI dependencies to your project if using Maven or Gradle:
org.apache.poi
poi
5.2.0
org.apache.poi
poi-ooxml
5.2.0
Step-by-Step Guide to Import Excel into JTable
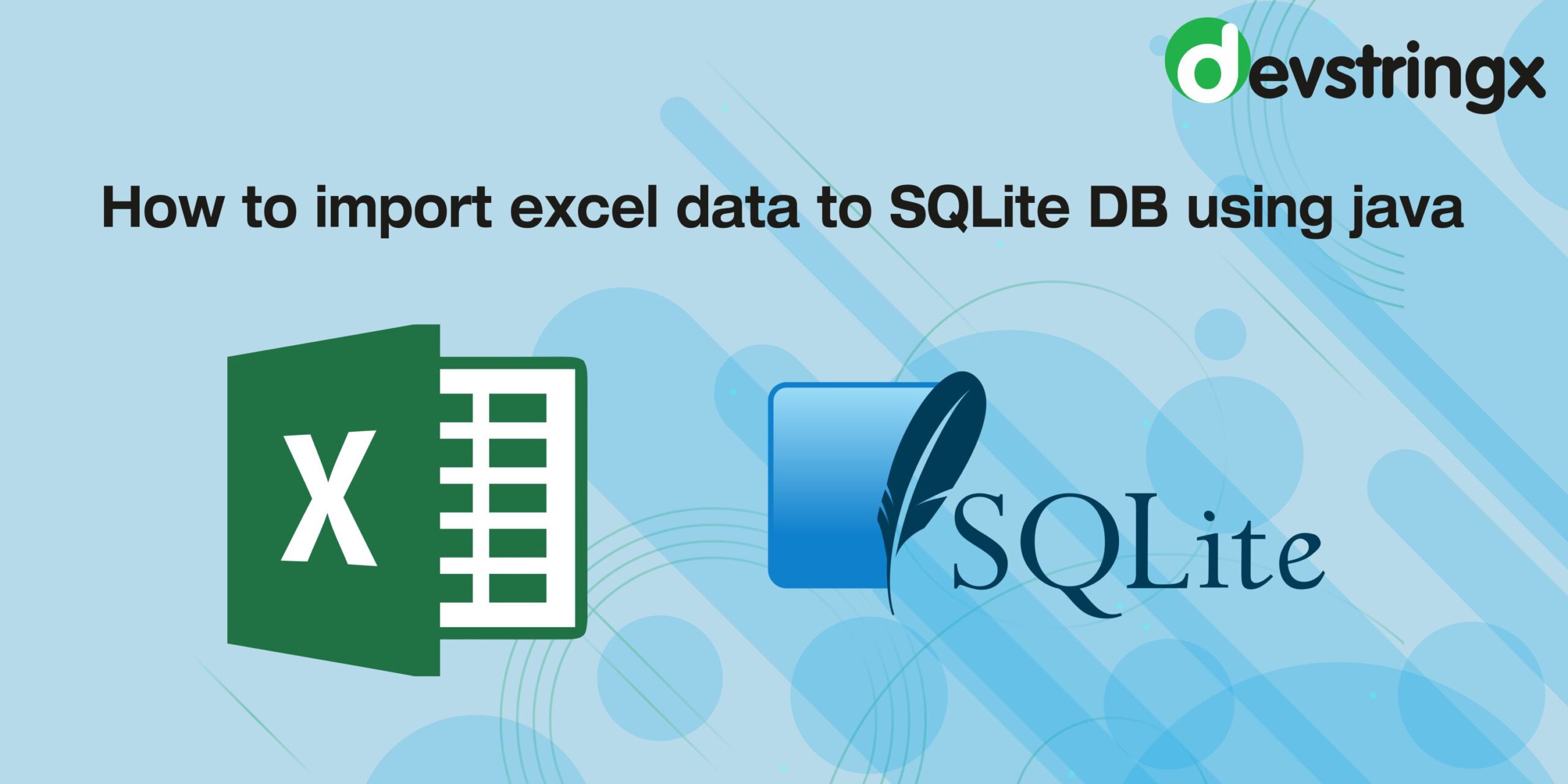
1. Import Necessary Libraries

Start by importing the required classes:
import javax.swing.;
import javax.swing.table.DefaultTableModel;
import org.apache.poi.ss.usermodel.;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
2. Reading Excel File

Here, we’ll read an Excel file named “data.xlsx”. Ensure this file is in your project directory or specify its path:
File file = new File(“data.xlsx”);
FileInputStream fis = new FileInputStream(file);
Workbook workbook = new XSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
3. Extracting Data into a JTable
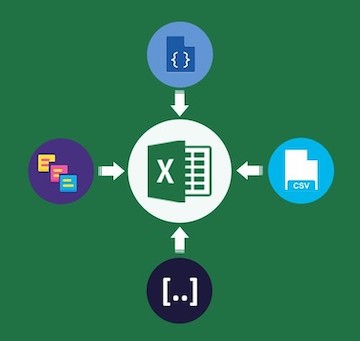
Now, let’s extract the data from the sheet into a JTable:
DefaultTableModel model = new DefaultTableModel();
for (Row row : sheet) {
String[] rowData = new String[sheet.getRow(0).getLastCellNum()];
for (int i = 0; i < sheet.getRow(0).getLastCellNum(); i++) {
Cell cell = row.getCell(i, Row.MissingCellPolicy.CREATE_NULL_AS_BLANK);
rowData[i] = cell.toString();
}
model.addRow(rowData);
}
JTable table = new JTable(model);
4. Displaying the JTable in a Frame

Finally, we’ll display the JTable in a JFrame to visualize the data:
JFrame frame = new JFrame(“Excel Data Imported”);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new JScrollPane(table));
frame.pack();
frame.setVisible(true);
🔔 Note: Make sure to handle exceptions properly, especially when dealing with file operations.
5. Closing Resources

It’s crucial to close the workbook and file input stream to prevent resource leaks:
workbook.close();
fis.close();
🔍 Note: When dealing with larger Excel files, consider using a streaming reader to manage memory usage effectively.
Enhancing Your Excel-JTable Integration

Here are some ways to improve the functionality:
- Auto-Adjust Column Width: Use
table.setAutoResizeMode(JTable.AUTO_RESIZE_OFF);
for more control over column widths. - Data Filtering: Add filters or search functionality to enhance user interaction.
- Dynamic Updates: Allow users to save changes back to the Excel file.
Throughout this guide, we've focused on importing Excel files into a JTable, providing a seamless experience for data visualization and manipulation within a Java Swing application. By following these steps, Java developers can effortlessly handle Excel data, making applications more versatile and user-friendly.
The process described here not only simplifies data import but also prepares your Java application for further customizations like data editing, sorting, and real-time updates. This level of integration enhances the user experience by bridging the gap between traditional file formats like Excel and the dynamic capabilities of Java GUIs.
How do I handle different Excel file formats?

+
Apache POI supports multiple Excel file formats like .xls (HSSFWorkbook) and .xlsx (XSSFWorkbook). You can use WorkbookFactory to create a workbook from any supported file type automatically.
Can I modify the Excel data once it’s in the JTable?

+
Yes, you can update the JTable model. To save changes back to Excel, you’ll need to write the data back using Apache POI’s write methods on the Workbook.
What if my Excel file has formulas or merged cells?

+
Formulas are often evaluated in Excel, so you might not get the formula itself but its current value. For merged cells, ensure you handle them correctly when reading cell values, as merged cells can confuse table data layout.