Python: Extract Data from Excel Sheets Easily
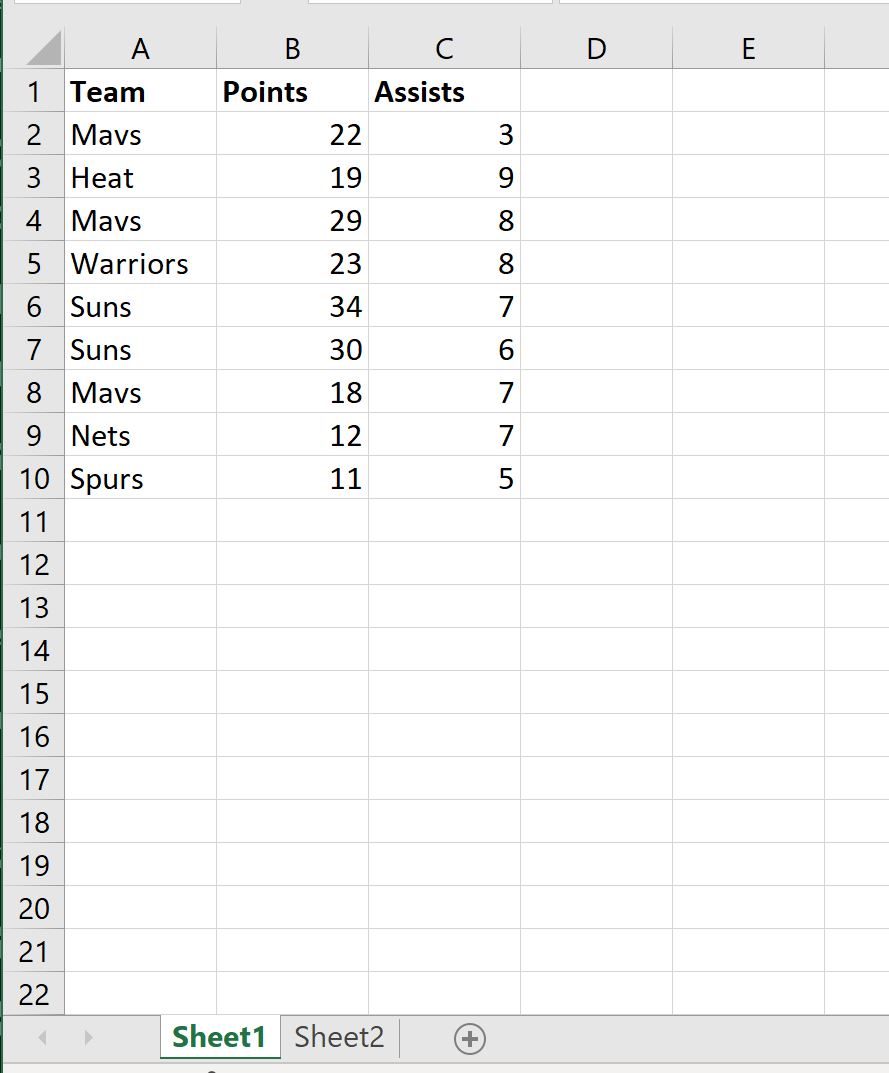
Excel is among the most popular tools for data management, and knowing how to extract data from Excel sheets programmatically can significantly enhance productivity and efficiency in data analysis. Python, with its versatile libraries like openpyxl and pandas, provides robust capabilities to interact with Excel files. In this long-form blog post, we will walk through the process of extracting data from Excel spreadsheets using Python, step-by-step.
Setting Up Your Python Environment

Before diving into data extraction, you need to ensure your Python environment is set up for this task:
- Install Python if you haven't already. Visit the Python website for download instructions.
- Install openpyxl and pandas. You can install these packages using pip:
pip install openpyxl pandas
Loading an Excel Workbook

Once you have the libraries installed, let's begin by loading an Excel workbook:
from openpyxl import load_workbook
# Load the workbook
workbook = load_workbook(filename='your_workbook.xlsx')
# Get the active sheet
sheet = workbook.active
⚠️ Note: Make sure the Excel file is closed to avoid potential errors due to lock issues.
Extracting Data with Openpyxl

Openpyxl lets you read cell values directly. Here's how you can extract data:
Reading Specific Cells

cell_value = sheet['A1'].value
print(cell_value)
Reading a Range of Cells

cell_range = sheet['A1:B10']
for row in cell_range:
for cell in row:
print(cell.value)
Reading Entire Columns

column_b = sheet['B']
for cell in column_b:
print(cell.value)
Advanced Data Extraction with Pandas

While openpyxl is great for basic tasks, pandas is a powerful tool for more complex data manipulation:
Reading an Excel File with Pandas

import pandas as pd
# Read the Excel file
df = pd.read_excel('your_workbook.xlsx', sheet_name='Sheet1')
# Display the DataFrame
print(df)
Filtering and Sorting Data

Pandas allows you to easily filter and sort your data:
# Filter for rows where 'ColumnA' is greater than 10
filtered_df = df[df['ColumnA'] > 10]
# Sort 'ColumnB' in descending order
sorted_df = df.sort_values(by='ColumnB', ascending=False)
# Display results
print(filtered_df)
print(sorted_df)
Data Manipulation and Analysis

After extracting your data, you might want to perform various operations:
Basic Operations

- Calculate the mean: `df['ColumnA'].mean()`
- Find the maximum value: `df['ColumnB'].max()`
- Sum values: `df['ColumnA'].sum()`
- Count non-null entries: `df['ColumnA'].count()`
Using Pandas for Complex Analysis

# Group by 'ColumnA' and calculate mean of 'ColumnB'
grouped = df.groupby('ColumnA')['ColumnB'].mean().reset_index(name='mean')
print(grouped)
Writing Back to Excel

Once you've manipulated the data, you might need to save it back to an Excel file:
# Save DataFrame to a new Excel file
df.to_excel('new_file.xlsx', index=False)
This ensures that any changes or analyses you've made are preserved in the Excel format.
Integrating with Other Data Sources

Python's extensive library ecosystem means you can integrate Excel data with various data sources or APIs:
- Database Integration: Use SQLAlchemy or psycopg2 to connect to databases like PostgreSQL.
- Web APIs: Use requests to fetch data from APIs.
- Combining Multiple Excel Files: Automate merging of data from several Excel sheets.
In the realm of data management, understanding how to extract, manipulate, and integrate data from Excel files can transform your workflow. Python’s libraries like openpyxl and pandas provide powerful tools to perform these tasks with ease. Here’s a recap of what we’ve covered:
- Setting up your Python environment with the necessary libraries.
- Loading Excel files using both openpyxl for basic manipulation and pandas for more advanced operations.
- Extracting data from specific cells, ranges, or entire columns, and performing analyses like filtering, sorting, and statistical calculations.
- Writing data back to Excel files to maintain your data’s usability in the familiar Excel format.
- Integrating with other data sources to broaden the scope of your data analysis.
Mastering these techniques opens up a multitude of opportunities for efficient data handling. Whether you’re merging data from multiple Excel sources, pulling in information from web APIs, or simply automating mundane data entry tasks, Python, with its simplicity and the right libraries, can handle it all effectively.
How do I handle errors when working with Excel files in Python?

+
Use try-except blocks to catch and handle exceptions like FileNotFoundError
or ValueError
. Additionally, ensure that the Excel file is not locked by another program, and that the file path is correct.
Can I modify the Excel file directly in Python?

+
Yes, using libraries like openpyxl, you can write data to specific cells or ranges, create new sheets, format cells, and save the changes back to the Excel file.
Is there a limit to the size of Excel files I can process with Python?
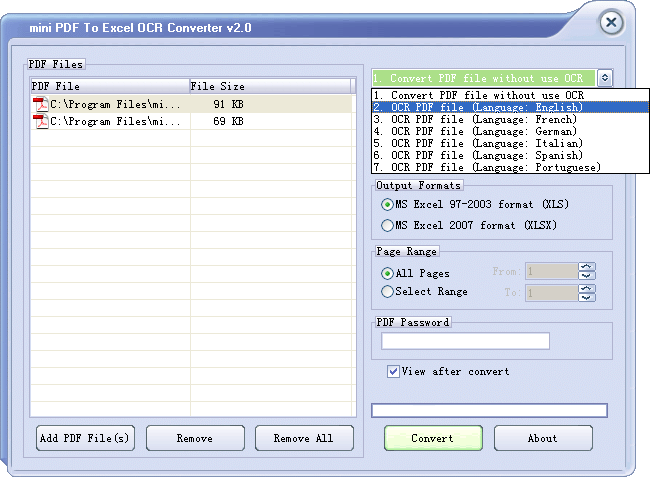
+
While there’s no strict limit, performance might degrade with very large files. For handling bigger datasets, consider reading the Excel file in chunks or using alternative data processing methods like SQL databases.