Excel VBA: List All Sheet Names Effortlessly

If you work with Microsoft Excel, particularly large workbooks with multiple sheets, managing them efficiently can become a challenge. Whether you're an accountant, data analyst, or even a casual user looking to organize large datasets, knowing how to list all sheet names using VBA can streamline your work and save time. Here's a comprehensive guide on how to achieve this effortlessly.
Understanding VBA in Excel

Visual Basic for Applications (VBA) is Excel’s programming language that allows automation of repetitive tasks, interaction with Excel’s user interface, and manipulation of worksheet data. Before diving into how to list sheet names, let’s briefly understand what VBA can do:
- Automate Tasks: Run macros to automate repetitive actions like data entry or formatting.
- Data Manipulation: Easily process and manipulate data across multiple sheets or workbooks.
- Custom Functions: Create custom functions that can perform operations not available with built-in Excel formulas.
- User Forms: Develop user interfaces for easier data input and interaction.
Why List Sheet Names?
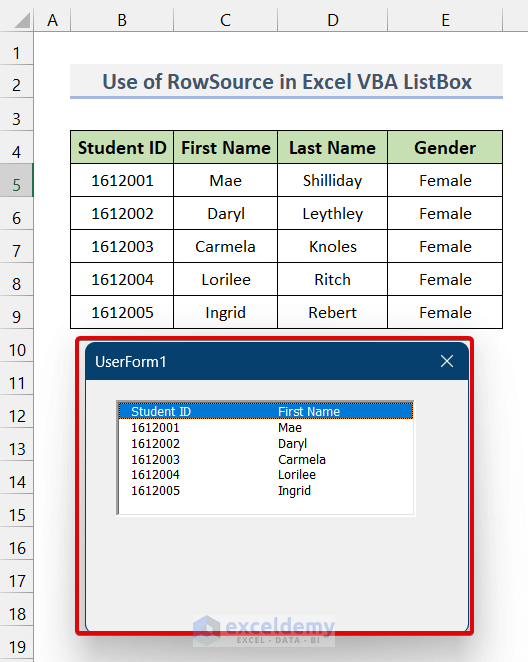
- Documentation: Keeps track of workbook structure for documentation purposes.
- Report Generation: Helps in generating reports that refer to multiple sheets.
- Data Integrity: Ensures no sheet is overlooked during data analysis or review.
Creating the VBA Macro to List Sheet Names

To list all sheet names in Excel using VBA:
- Open your Excel workbook.
- Press Alt + F11 to open the VBA editor.
- Right-click on any of the objects in the 'Project Explorer' on the left.
- Choose Insert then Module to create a new module.
- Paste the following VBA code into the module:
Sub ListAllSheetNames()
Dim ws As Worksheet
Dim sheetNames As String
' Loop through each sheet in the workbook
For Each ws In ThisWorkbook.Worksheets
sheetNames = sheetNames & ws.Name & vbCrLf
Next ws
' Insert the list of sheet names in cell A1 of the active sheet
ActiveSheet.Range("A1").Value = sheetNames
End Sub
Let's break down this code:
Dim ws As Worksheet
: Declares a variable to hold each worksheet object.Dim sheetNames As String
: Variable to store the concatenated names of sheets.For Each ws In ThisWorkbook.Worksheets
: Loop through every sheet in the active workbook.sheetNames = sheetNames & ws.Name & vbCrLf
: Concatenate each sheet name to the string, adding a line break.ActiveSheet.Range("A1").Value = sheetNames
: Assign the collected names to cell A1 of the active sheet.
⚠️ Note: This script will overwrite any data in cell A1. Always ensure you have backed up your data before running VBA macros.
Enhancing the Macro

If you need additional functionality:
- List Sheets in a Specific Workbook: You might want to list sheets from a workbook other than the active one. Change
ThisWorkbook
toWorkbooks("WorkbookName").Worksheets
. - Sort Sheet Names: By adding VBA code to sort the array of sheet names before inserting into the sheet.
- Include Hidden Sheets: Modify the loop to include hidden sheets by testing for visibility.
Sub EnhancedListAllSheetNames()
Dim ws As Worksheet
Dim sheetNames() As String
Dim i As Long, j As Long
' Resize the array to the number of sheets
ReDim sheetNames(1 To ThisWorkbook.Worksheets.Count)
' Loop through each sheet in the workbook
For i = 1 To ThisWorkbook.Worksheets.Count
Set ws = ThisWorkbook.Worksheets(i)
sheetNames(i) = ws.Name
Next i
' Sort the sheet names
For i = LBound(sheetNames) To UBound(sheetNames)
For j = i + 1 To UBound(sheetNames)
If sheetNames(i) > sheetNames(j) Then
Dim temp As String
temp = sheetNames(i)
sheetNames(i) = sheetNames(j)
sheetNames(j) = temp
End If
Next j
Next i
' Insert the sorted list of sheet names in cell A1 of the active sheet
ActiveSheet.Range("A1").Resize(UBound(sheetNames)) = Application.Transpose(sheetNames)
End Sub
This enhanced macro sorts sheet names alphabetically and places them in cells from A1 downwards, avoiding overwriting existing data beyond A1.
Final Thoughts

By leveraging VBA, listing all the sheet names in an Excel workbook becomes a task that can be executed with a few clicks. This not only saves time but also reduces the risk of errors when manually documenting sheet names. Remember that while VBA offers tremendous control and flexibility, it also comes with the responsibility to ensure data integrity and security. Always backup your work before executing new macros, and consider learning more about VBA to unlock further automation possibilities in Excel.
How can I run the VBA macro to list sheet names?

+
Open the VBA editor with Alt + F11, go to the module where you’ve added the macro code, and click the Run button or press F5 to execute the macro.
Can I list sheet names from closed workbooks?

+
Yes, with more complex VBA code. However, this often requires opening the workbook programmatically, extracting the sheet names, and then closing it. The process is more involved and beyond the scope of this introductory tutorial.
Is there a way to list sheet names without VBA?

+
Yes, though it’s less efficient. You can manually type out the names or use Excel’s built-in Name Manager to reference and list sheet names, but this would be time-consuming and prone to errors for large workbooks.