5 Easy Steps to Create Excel Sheets in MVC

Introduction to Excel and MVC Integration
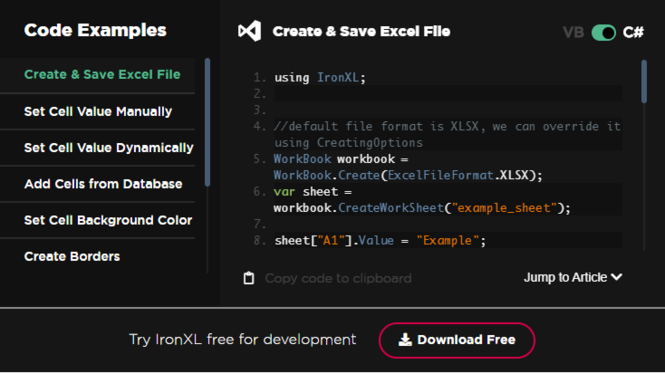
Excel is an incredibly powerful tool for managing and analyzing data, and integrating it with your MVC application can significantly enhance data manipulation capabilities. Whether you’re working on a web application that needs to generate reports, allow users to download data, or manage complex datasets, learning how to integrate Excel functionality into an MVC framework is invaluable. This guide will walk you through 5 easy steps to create Excel sheets in MVC, ensuring that you can leverage Excel’s capabilities within your web applications.
Step 1: Set Up Your Development Environment

Before diving into the actual integration, you must ensure that your development environment is ready. Here’s what you need:
- ASP.NET MVC framework installed: Ensure you are using a version that supports your intended backend language (C#, VB.NET).
- Visual Studio or another IDE: For coding, debugging, and deployment.
- EPPlus library: An open-source .NET library for reading and writing Excel files. Add it via NuGet Package Manager in Visual Studio:
Install-Package EPPlus
🔗 Note: Make sure your project has the necessary dependencies installed. EPPlus is the library of choice here due to its ease of use and functionality.
Step 2: Create a Controller for Excel Export

Controllers in MVC handle the flow of data between the View and the Model. For Excel integration:
- Create a new controller: Name it something like
ExcelExportController
. - Define actions: Include methods to trigger Excel export and handle data preparation.
public class ExcelExportController : Controller
{
[HttpGet]
public ActionResult ExportToExcel()
{
// Implementation will follow
return View();
}
}
Step 3: Prepare Data for Export
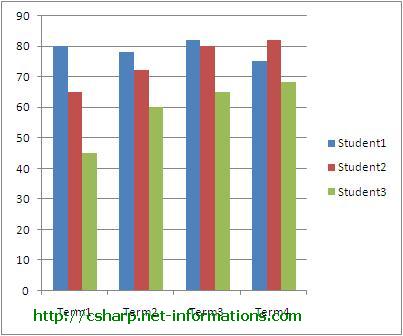
The next step involves preparing the data that you wish to export to Excel:
- Retrieve data: Fetch data from your database or any data source.
- Format data: Ensure the data is in a format that can be easily converted to an Excel sheet.
public ActionResult ExportToExcel()
{
var data = db.YourModel.ToList(); // Retrieve data from your model or any other data source
return View(data);
}
🔗 Note: Proper data formatting is crucial to ensure that Excel understands and displays your data correctly.
Step 4: Generate the Excel File Using EPPlus

With the data prepared, you’ll now generate the Excel file:
- Use EPPlus: Call methods to create, format, and save the Excel file.
- Stream the file: Send the Excel file as a response to the client for download.
public FileResult ExportToExcel() { var data = db.YourModel.ToList(); // Fetch your data
using (ExcelPackage pck = new ExcelPackage()) { // Add a new worksheet ExcelWorksheet ws = pck.Workbook.Worksheets.Add("Sheet1"); // Load data into the worksheet ws.Cells["A1"].LoadFromCollection(data, true); // Auto-fit columns ws.Cells[ws.Dimension.Address].AutoFitColumns(); // Save and return file for download byte[] fileContent = pck.GetAsByteArray(); return File(fileContent, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet", "export.xlsx"); }
}
🔗 Note: Remember to dispose of the ExcelPackage object properly to avoid memory leaks.
Step 5: Implement in the View or as an Action Link
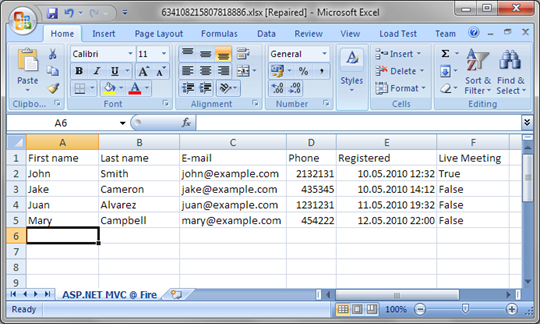
To allow users to trigger the export, you’ll need to provide an interface:
- Action Link: Add a link or button in your view to trigger the Excel export.
- Form Submit: Alternatively, use form submission to initiate the download.
@Html.ActionLink(“Export to Excel”, “ExportToExcel”, “ExcelExport”, null, new { @class = “btn btn-primary” })
In wrapping up our journey through integrating Excel with MVC, we've explored how to set up your development environment, create a controller for Excel exports, prepare your data, generate the Excel file using EPPlus, and implement the export functionality in your web application's user interface. This process not only streamlines data reporting and analysis but also empowers your web applications with dynamic data export capabilities.
By following these steps, you can efficiently export data from your MVC application to Excel, providing users with familiar tools to manipulate and analyze their data further. This integration not only enhances the functionality of your application but also makes data handling more intuitive and user-friendly, which in turn can lead to better data insights and improved productivity.
How does MVC improve the export to Excel?

+
MVC provides a structured way to organize your application, separating concerns between the Model (data), View (UI), and Controller (logic), making the process of generating Excel reports more manageable and modular. It allows for better maintainability and the potential to use different technologies for each layer.
Can I use libraries other than EPPlus for Excel generation in MVC?

+
Yes, there are several other libraries available like ClosedXML, NPOI, or even Office Interop for Excel. EPPlus was highlighted here for its simplicity and performance, but your choice might vary depending on your project’s specific requirements or dependencies.
Is it necessary to generate Excel files server-side, or can it be done client-side?

+
Generating Excel files server-side allows for better security, performance, and control over the data and file generation process. However, client-side options like JavaScript Excel libraries (e.g., XLSX.js) exist, which can be useful for simple tasks but might not offer the same level of functionality or performance.
Related Terms:
- C OfficeOpenXml create excel file
- Export excel Asp Net MVC
- MVC export to Excel
- C export to Excel
- Upload Excel asp net MVC