Creating Excel Sheets in Java with JXL: Simple Guide
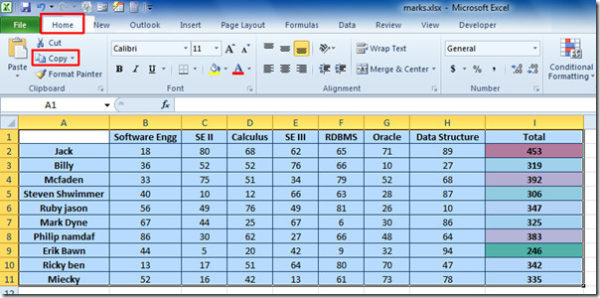
The JXL (Java Excel API) library is an incredibly versatile tool for anyone looking to create, modify, or read Excel files through Java programming. Whether you're dealing with report generation, data analysis, or just need to automate certain tasks in Excel, JXL provides a robust framework to accomplish these tasks efficiently.
Setting Up Your Environment
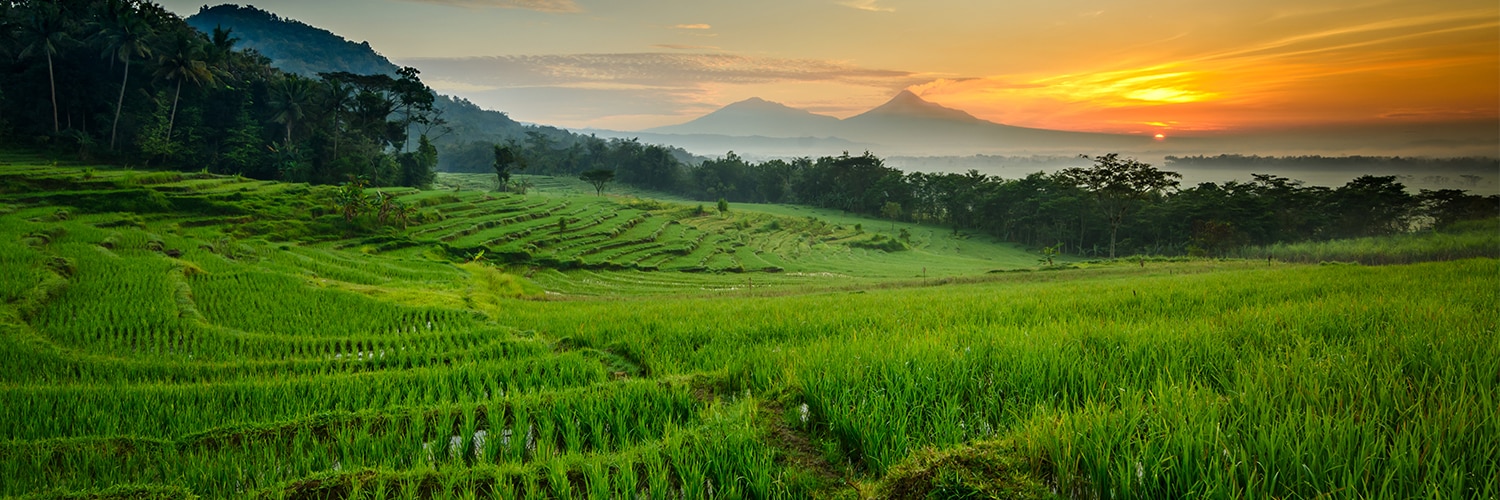
Before diving into coding with JXL, setting up your development environment is crucial:
- Download JXL: Visit the JXL library’s official site or use Maven/Gradle to include JXL in your project.
- Install Java Development Kit (JDK): Ensure you have at least JDK 1.5 or above for compatibility with JXL.
- Setup Your IDE: Use any popular IDE like Eclipse, IntelliJ IDEA, or NetBeans for your Java development.
Creating a New Excel Workbook
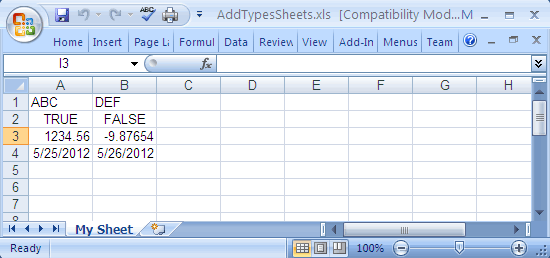
Creating an Excel workbook using JXL involves several key steps:
import jxl.; import jxl.write.; import java.io.File;
public class CreateExcelSheet { public static void main(String[] args) { try { WritableWorkbook workbook = Workbook.createWorkbook(new File(“example.xls”)); WritableSheet sheet = workbook.createSheet(“First Sheet”, 0);
// Add label to the sheet Label label = new Label(0, 0, "My First Excel File"); sheet.addCell(label); // Writing to the Excel file workbook.write(); workbook.close(); } catch (Exception e) { System.out.println(e); } }
}
Adding Data to Your Sheet

After creating the workbook, you might want to add more data:
- Labels: For text entries.
- Numbers: For numerical data.
- Formulas: To perform calculations within Excel.
Here’s how you can add different types of data:
// Add more labels Label label1 = new Label(1, 0, “Year”); sheet.addCell(label1);
Label label2 = new Label(2, 0, “Sales”); sheet.addCell(label2);
// Add numbers Number number = new Number(1, 1, 2023); sheet.addCell(number);
// Add formulas Formula formula = new Formula(2, 1, “B2 * 100”); sheet.addCell(formula);
📝 Note: Remember to handle exceptions when dealing with file I/O operations to ensure your program can gracefully handle errors.
Formatting Cells

Enhance the visual appeal of your Excel sheet by applying formatting:
WritableCellFormat cellFormat = new WritableCellFormat(); cellFormat.setBackground(Colour.GREY_25); cellFormat.setAlignment(Alignment.CENTRE); cellFormat.setVerticalAlignment(VerticalAlignment.CENTRE); cellFormat.setBorder(Border.ALL, BorderLineStyle.DOUBLE);
Label title = new Label(0, 0, “Sales Data”, cellFormat); sheet.addCell(title);
Working with Multiple Sheets
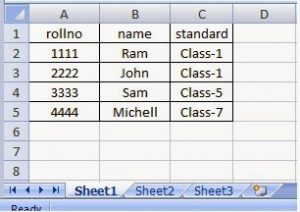
Adding multiple sheets to your workbook is straightforward:
WritableSheet sheet1 = workbook.createSheet(“Sheet1”, 0);
WritableSheet sheet2 = workbook.createSheet(“Sheet2”, 1);
Adding Images to Excel

Inserting images into Excel cells can provide a visual touch to your report:
try {
WritableImage image = new WritableImage(0, 2, 2, 2, new File(“path_to_image.jpg”));
sheet.addImage(image);
} catch (Exception e) {
e.printStackTrace();
}
🖼️ Note: Image paths should be absolute or relative depending on where your application is running from.
As we wrap up our journey through creating Excel sheets with JXL in Java, it’s clear that this library simplifies complex tasks related to Excel file manipulation. From basic data entry to advanced formatting and image insertion, JXL provides the tools needed to automate and enhance Excel workflows. This guide has explored setting up your environment, creating and populating workbooks, applying formatting, and working with multiple sheets and images. By understanding these functionalities, you can significantly improve your ability to work with Excel files programmatically, making data manipulation and report generation much more efficient.
What are the main advantages of using JXL over POI?
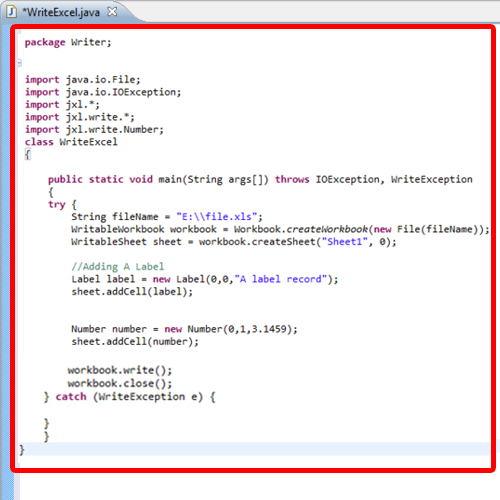
+
JXL is known for its simplicity and ease of use, especially for straightforward Excel operations. It’s lightweight, which makes it faster in some scenarios, particularly when dealing with Excel files that don’t require complex operations like charts or pivot tables.
Can JXL handle Excel 2007 and later file formats?
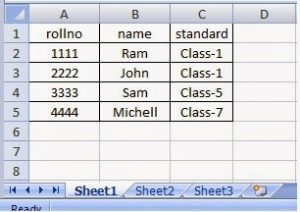
+
No, JXL primarily supports .xls files from Excel 97 to Excel 2003. For newer .xlsx files, you would need to use Apache POI or other libraries compatible with Office Open XML formats.
How can I insert charts into my Excel sheet using JXL?
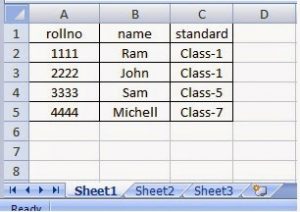
+
JXL does not support direct chart creation. However, you can create charts externally and then insert them as images. For dynamic chart creation, you might need to look at Apache POI or other alternatives.
How do I add hyperlinks or comments to cells with JXL?
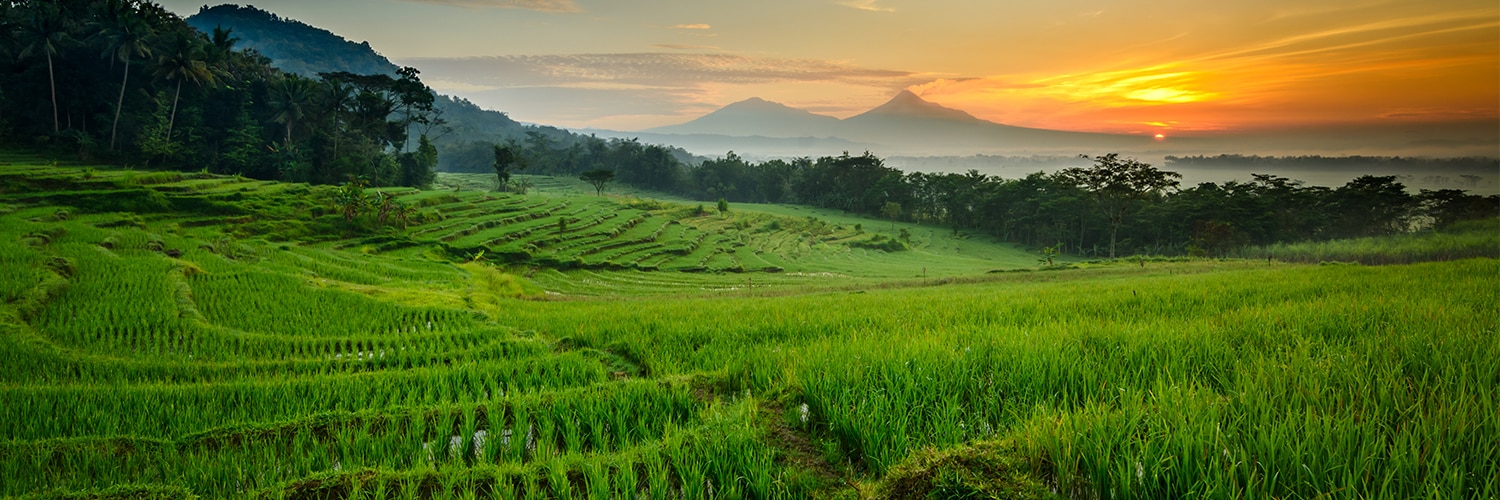
+
JXL supports adding hyperlinks with the Hyperlink
class, allowing you to link to URLs, documents, or within the same workbook. Comments can be added using the WritableCellComment
class.