5 Ways to Insert Images in Excel with C

If you are a developer or a data analyst working extensively with Microsoft Excel, integrating images into spreadsheets programmatically can significantly streamline workflows and enhance the presentation of data. Whether it's for generating reports, dashboards, or custom data visualizations, Excel offers various methods to embed images using C#, the programming language known for its versatility and robustness in .NET environments. In this comprehensive guide, we'll explore five distinct ways to insert images in Excel using C#.
1. Using the Excel Interop Library

The Microsoft Office Interop library allows you to automate Excel from C# by treating it as an extension of your application. This approach provides a direct, albeit somewhat outdated, method for working with Excel’s COM interface.
- Pros: Direct access to Excel features, full control over Excel’s object model.
- Cons: Requires Excel to be installed on the machine, can be slower, requires COM interop.
To insert an image:
using Excel = Microsoft.Office.Interop.Excel;
Excel.Application excelApp = new Excel.Application();
Excel.Workbook workbook = excelApp.Workbooks.Open(@"C:\YourPath\YourWorkbook.xlsx");
Excel.Worksheet sheet = workbook.Sheets[1];
Excel.Shapes shapes = sheet.Shapes;
shapes.AddPicture(@"C:\YourPath\YourImage.png", Microsoft.Office.Core.MsoTriState.msoFalse, Microsoft.Office.Core.MsoTriState.msoTrue, 100, 100, 200, 200);
workbook.SaveAs(@"C:\YourPath\ModifiedWorkbook.xlsx");
workbook.Close();
excelApp.Quit();
📝 Note: Ensure that your project has a reference to Microsoft.Office.Interop.Excel and that you handle the Excel process appropriately to avoid leaving instances running in the background.
2. Utilizing EPPlus Library

EPPlus is a .NET library for managing Excel spreadsheets. It’s widely used for its ease of use and rich features.
- Pros: Faster than Interop, no need for Excel installation, cross-platform compatibility.
- Cons: Some features of Excel might not be available or require extra work.
Here’s how you can add an image:
using OfficeOpenXml;
using System.IO;
using (ExcelPackage package = new ExcelPackage(new FileInfo(@"C:\YourPath\YourWorkbook.xlsx")))
{
ExcelWorksheet worksheet = package.Workbook.Worksheets.Add("Sheet1");
FileInfo imageInfo = new FileInfo(@"C:\YourPath\YourImage.png");
worksheet.Drawings.AddPicture("Image", imageInfo);
package.SaveAs(new FileInfo(@"C:\YourPath\ModifiedWorkbook.xlsx"));
}
📝 Note: EPPlus uses streams to manage the Excel file, which is efficient but can be memory-intensive for large files.
3. Inserting Images with OpenXML SDK
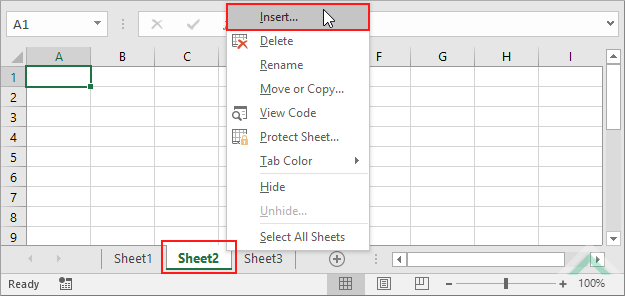
OpenXML SDK provides direct access to Excel’s file format, giving you fine-grained control over the XML that makes up the Excel file.
- Pros: No need for Excel installation, very lightweight, and efficient for file manipulation.
- Cons: Direct manipulation of XML can be complex and error-prone.
An example:
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Spreadsheet;
using System.IO;
using A = DocumentFormat.OpenXml.Drawing;
using Xdr = DocumentFormat.OpenXml.Drawing.Spreadsheet;
using (SpreadsheetDocument document = SpreadsheetDocument.Open(@"C:\YourPath\YourWorkbook.xlsx", true))
{
WorkbookPart workbookPart = document.WorkbookPart;
WorksheetPart worksheetPart = workbookPart.WorksheetParts.First();
DrawingsPart drawingsPart = worksheetPart.AddNewPart<DrawingsPart>();
worksheetPart.Worksheet.Append(new DocumentFormat.OpenXml.Spreadsheet.Drawing()
{
Id = drawingsPart.GetIdOfPart(drawingsPart),
Name = "Drawing1"
});
ImagePart imagePart = drawingsPart.AddImagePart(ImagePartType.Jpeg);
using (FileStream stream = new FileStream(@"C:\YourPath\YourImage.jpg", FileMode.Open))
{
imagePart.FeedData(stream);
}
Xdr.WorksheetDrawing worksheetDrawing = new Xdr.WorksheetDrawing();
Xdr.TwoCellAnchor twoCellAnchor = new Xdr.TwoCellAnchor();
Xdr.Picture picture = new Xdr.Picture();
worksheetDrawing.Append(twoCellAnchor);
twoCellAnchor.Append(picture);
picture.Append(new Xdr.NonVisualPictureProperties());
picture.Append(new Xdr.BlipFill()
{
Blip = new A.Blip()
{
Embed = drawingsPart.GetIdOfPart(imagePart)
}
});
picture.Append(new Xdr.ShapeProperties());
drawingsPart.WorksheetDrawing = worksheetDrawing;
worksheetPart.Worksheet.Save();
}
4. Using SpreadsheetGear

SpreadsheetGear is a commercial library for .NET that supports Excel file manipulation with high performance.
- Pros: Native .NET implementation, high performance, comprehensive Excel support.
- Cons: Commercial licensing costs.
Example:
using SpreadsheetGear;
using System.IO;
IWorkbook workbook = Factory.GetWorkbook(@"C:\YourPath\YourWorkbook.xlsx");
IWorksheet worksheet = workbook.Worksheets[0];
IImage image = worksheet.Cells["A1"].AddPicture(@"C:\YourPath\YourImage.png", PictureFormat.Png);
image.Placement = Placement.FreeFloating;
workbook.SaveAs(@"C:\YourPath\ModifiedWorkbook.xlsx", FileFormat.OpenXMLWorkbook);
5. Writing Custom Code with OleDb

If you want to avoid external libraries, you can use the System.Data.OleDb namespace to write images into Excel cells.
- Pros: No third-party libraries required, basic manipulation capabilities.
- Cons: Limited functionality, not suitable for complex tasks.
Here’s how:
using System.Data.OleDb;
using System.IO;
string connString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\\YourPath\\YourWorkbook.xlsx;Extended Properties=\"Excel 12.0;HDR=YES;IMEX=1;\"";
using (OleDbConnection conn = new OleDbConnection(connString))
{
conn.Open();
using (OleDbCommand cmd = new OleDbCommand("INSERT INTO [Sheet1$] (ImageColumn) VALUES (?)", conn))
{
byte[] imageBytes = File.ReadAllBytes(@"C:\YourPath\YourImage.jpg");
cmd.Parameters.AddWithValue("?", imageBytes);
cmd.ExecuteNonQuery();
}
}
To wrap up, this guide has covered five different approaches to inserting images into Excel spreadsheets using C#. Each method has its advantages, depending on your specific requirements:
- Interop: Offers full control but requires Excel to be installed.
- EPPlus: Efficient and cross-platform, with easy to use API.
- OpenXML SDK: Lightweight and powerful but requires XML knowledge.
- SpreadsheetGear: High performance with a commercial cost.
- OleDb: Simplistic but limited in functionality.
When deciding which approach to use, consider the project's complexity, the need for specific Excel features, licensing considerations, and performance requirements. Each method has its place, and understanding them can help you choose the best tool for your data management and presentation needs.
Can I resize or move images inserted using these methods?

+
Yes, with methods like EPPlus or Interop, you can modify the image properties to resize or reposition them within the spreadsheet.
Do these methods support all image formats?

+
Most methods support common formats like PNG, JPEG, GIF, but not all might support less common formats like TIFF or BMP. Always check the documentation or test the specific formats you need.
Is it possible to automate image insertion for multiple spreadsheets at once?

+
Absolutely. You can loop through multiple Excel files or worksheets using any of these methods to automate the process for bulk insertion.
What if I need to manipulate Excel files on a server without Excel installed?

+
Options like EPPlus, OpenXML SDK, or third-party libraries like SpreadsheetGear are ideal for server-side operations where Excel isn’t installed.
How does the performance compare among these methods?

+
Performance varies:
- EPPlus and SpreadsheetGear are generally faster than Interop.
- OpenXML SDK can be very efficient for manipulating file structure directly.
- OleDb method might be slower for large operations or complex spreadsheets.
In summary, this guide has explored five different methods to insert images into Excel using C#, each suited to different scenarios and requirements. Whether you're looking for a straightforward, no-install approach or need the power of Excel's full feature set, there's a method here for you. Understanding these methods will allow you to integrate images efficiently into your Excel-based applications or reports, enhancing data presentation and analysis.