5 Ways to Unprotect Excel Sheets in C Without Password

Protecting your Excel sheets can be crucial for maintaining the security and privacy of your data. However, there might come a time when you need to bypass these protections for legitimate reasons. This guide outlines five effective methods to unprotect Excel sheets in C without requiring the original password, using the versatile C language.
Method 1: Reading Excel File Structure

Excel files are essentially ZIP archives containing XML files, which can be manipulated to remove protection:
- Open the Excel file as a ZIP archive.
- Navigate to the ‘xl/worksheets’ folder where each sheet is represented as an XML file.
- Find the XML tags that indicate sheet protection.
- Modify or delete these tags to remove protection.
To achieve this in C, you’ll need a library to handle ZIP files. Here’s a simple example using zlib:
#include <stdio.h>
#include <zlib.h>
void unprotectExcel(const char* filename) {
// Code to uncompress Excel file and modify sheet protection
}
🔍 Note: This method assumes the file is not password-protected at the file level. If it is, you'll need to handle decryption or find another method.
Method 2: Using Excel’s XML Security Features

Excel uses XML for its structure, and protection is often stored within:
- Analyze the XML for protection elements.
- Edit or remove the relevant XML tags.
- Re-save the file without protection.
#include <libxml/parser.h>
#include <libxml/tree.h>
void removeProtection(const xmlDoc *doc) {
// Code to remove protection elements from XML
}
Method 3: Brute-Force Password Cracking

Although not recommended due to ethical considerations, here’s how to implement it:
- Use cryptographic libraries to attempt different passwords.
- Check if the sheet can be accessed without exception.
- Iterate through common or guessed passwords.
#include <openssl/md5.h>
#include <string.h>
int crackExcelPassword(const char* filename, char* passwords) {
// Brute-force code here
}
⚠️ Note: Password cracking is ethically sensitive; use it only if you have legal permission or own the document.
Method 4: Exploiting Excel File Encryption
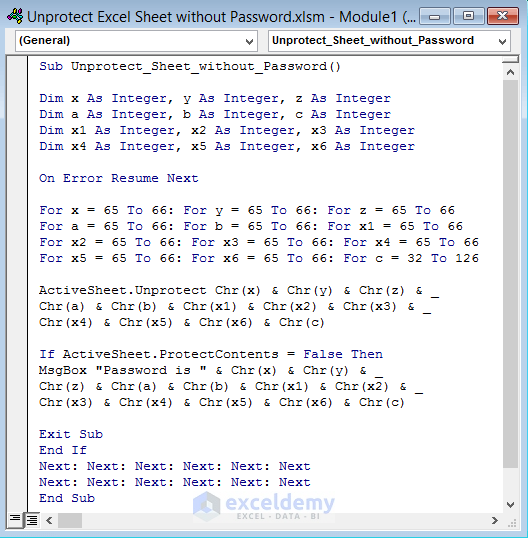
If Excel files are encrypted, here’s how you can approach:
- Analyze the encryption algorithm used by Excel.
- Use cryptographic libraries to understand or bypass the encryption.
- Manipulate the encrypted data to unprotect sheets.
#include <gcrypt.h>
void unprotectEncryptedExcel(const char* filename) {
// Code to decrypt and manipulate file
}
Method 5: Third-Party Libraries

Utilizing pre-built tools can simplify the process:
- Find libraries designed to interact with Excel files.
- Use functions provided to unprotect sheets or sheets specifically.
#include "some_third_party_excel_library.h"
void unprotectSheets(const char* filename) {
// Code using third-party library
}
Each method has its merits, depending on your scenario. Here's a summarized comparison in a table:
Method | Complexity | Ethical Considerations |
---|---|---|
Reading File Structure | Moderate | Generally Safe |
XML Manipulation | Moderate | Safe |
Brute-Force | High | Questionable |
File Encryption Exploit | High | Highly Questionable |
Third-Party Libraries | Low | Safe if Used Legally |

When considering these methods, remember the following:
- Only use these techniques if you have the right to unprotect the file.
- Respect data privacy and ownership laws.
- Secure your data by not over-relying on the default Excel protection mechanisms.
Exploring Excel sheet protection in C offers both educational insights and practical solutions for data security. Whether through XML manipulation, brute-force password cracking, or exploiting file encryption, these methods provide a glimpse into how Excel's security mechanisms work and can be bypassed under specific circumstances. While some methods raise ethical questions, understanding them is crucial for anyone involved in data security or software development.
Is it legal to unprotect an Excel sheet without a password?

+
Unprotecting an Excel sheet without a password can be legal if you have the authority to do so, for example, if you are the owner of the document or if you have explicit permission from the owner. However, unauthorized access to someone else’s protected data is often illegal and unethical.
Can these methods corrupt the Excel file?

+
There’s always a risk of file corruption when manipulating its internal structure or content. Always make a backup before attempting any of these methods to avoid data loss.
What are the alternatives to unprotecting sheets for data security?

+
For enhanced data security, consider using stronger file-level encryption, maintaining control over data access, or using enterprise-level security tools that manage permissions and provide auditing capabilities.