5 Ways to Use HashMap for Reading Excel Data

The use of HashMap in Java for handling data from Excel files can greatly improve efficiency, readability, and maintainability of your applications. Here are five innovative ways to harness HashMap when dealing with Excel data:
Mapping Excel Columns to HashMap

One of the primary uses of HashMap with Excel files is to create a mapping from column headers to their respective data:
- Extract column names from the first row of the Excel sheet.
- Use these column names as keys in your HashMap, with each key’s value being an ArrayList to hold the column’s data.
This approach allows for:
- Easier access to specific columns for data manipulation.
- Dynamic handling of columns when they change position or are added/removed in the spreadsheet.
- Reduced chance of errors when referencing column data.
Here’s how it might look:
Column Name | Data List |
---|---|
ID | [1, 2, 3, …] |
Name | [John, Jane, Doe, …] |
Age | [25, 30, 35, …] |

Grouping Data by Unique Identifiers

You can use HashMap to group data from an Excel sheet:
- Choose an identifier like a student ID, employee ID, or any unique value.
- Use this identifier as the key to store related data in a HashMap.
This grouping can be utilized to:
- Summarize or aggregate data based on these identifiers.
- Perform quick lookups for related records.
- Generate reports or dashboards where data is grouped by specific criteria.
⚠️ Note: Ensure the identifiers are unique to avoid data collision.
Using HashMap for Data Lookup

By loading an Excel file into a HashMap, you can achieve:
- Rapid access to records by creating a lookup table.
- Optimize for scenarios where you need to access data points frequently or perform searches.
Transposing Excel Data

Transposing rows to columns or vice versa can be challenging, but with HashMap, it becomes straightforward:
- Create a nested HashMap where the outer key is the original column, and the inner key is the row number, with the corresponding cell value as the value.
- Then, by swapping keys, you can transpose the data effectively.
Creating Custom Excel Data Structures
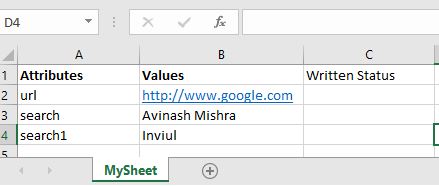
Develop custom data structures:
- Create complex key-value pairs to represent different elements of your data model.
- Store and manipulate nested Excel data in a more flexible and intuitive manner.
Overall, HashMap's versatility allows you to manage Excel data with greater efficiency, reducing the complexity of handling large datasets. By adopting these methods, you can streamline your data processing tasks, making your code cleaner, more reusable, and easier to maintain.
How do I load Excel data into a HashMap?

+
First, read the Excel file using libraries like Apache POI or JExcelAPI. Iterate through each row, capturing column headers for keys and row data for values. Store these in a HashMap where the key is the column header, and the value is an ArrayList of cell values.
What should I consider when choosing keys for my HashMap?

+
Ensure keys are unique and representative of the data. Avoid using keys that might not be unique or that are subject to change frequently.
Can I use HashMap to edit Excel data?

+
Yes, you can update values or add new entries to a HashMap, which can then be written back to an Excel file after processing.
How can HashMap improve performance with Excel data?

+
HashMap provides constant-time complexity for basic operations like adding, removing, or looking up entries, making data retrieval faster compared to iterating through an entire list or array.