5 Easy Steps to Read Excel Data with MATLAB

Reading Excel files with MATLAB is a skill that can significantly boost productivity for data analysts, engineers, and researchers. Whether you're dealing with financial data, scientific experiments, or any form of tabular information, MATLAB's ability to interface seamlessly with Excel provides a powerful toolset for data manipulation, analysis, and visualization. Here, we'll walk through 5 easy steps to read Excel data using MATLAB, ensuring you get the most out of this versatile programming environment.
Step 1: Preparing Your Environment

- Install MATLAB: Ensure that you have MATLAB installed. You can download the latest version from the official MATLAB website if you haven’t already.
- Verify Excel Support: Check if the version of MATLAB you are using supports Excel file reading. MATLAB’s Excel support is typically included in versions released after R2016b.
- Set Up Excel Files: Have your Excel file ready. Ensure it’s in a known location, and remember the full path to the file, or place it in MATLAB’s working directory.
💡 Note: If you’re using a network drive or have not properly set the file path, MATLAB might not locate the file, causing errors.
Step 2: Importing the Data

There are multiple ways to import data from Excel into MATLAB, each suited for different use cases:
Using UI Import Tool

- Navigate to the Home tab in MATLAB.
- Click on ‘Import Data’ and select your Excel file. This method is great for quick data import with a visual interface.
- Choose the worksheet and the range of cells you want to import. You can also set data types and rename variables for clarity.
Using MATLAB Code

filename = ‘full_path_to_your_excel_file.xlsx’;
sheet = 1;
xlRange = ‘A1:F100’;
[num, txt, raw] = xlsread(filename, sheet, xlRange);
- filename: The path to your Excel file.
- sheet: The number of the worksheet or its name.
- xlRange: The cell range to read, for example, ‘A1:F100’.
📘 Note: For large datasets or dynamic ranges, you might need to adjust xlRange or use xlsfinfo() to determine the last row or column with data.
Step 3: Data Processing and Cleaning

Once you’ve imported your data into MATLAB, it often requires cleaning and processing:
- Remove Missing Values: Use the
ismissing()
orrmmissing()
functions to handle or remove missing data. - Convert Data Types: Sometimes, the data imported might not be in the correct type. Use
str2double()
,str2num()
, or other conversion functions. - Naming Variables: Rename variables for easier reference.
Step 4: Data Analysis

Now, your data is ready for analysis. Here are some basic analytical functions you might use:
Function | Purpose |
---|---|
`mean(data)` | Calculate the mean of the dataset |
`std(data)` | Calculate the standard deviation |
`max(data)` | Find the maximum value |
`histogram(data)` | Create a histogram for distribution analysis |

Step 5: Exporting Your Results
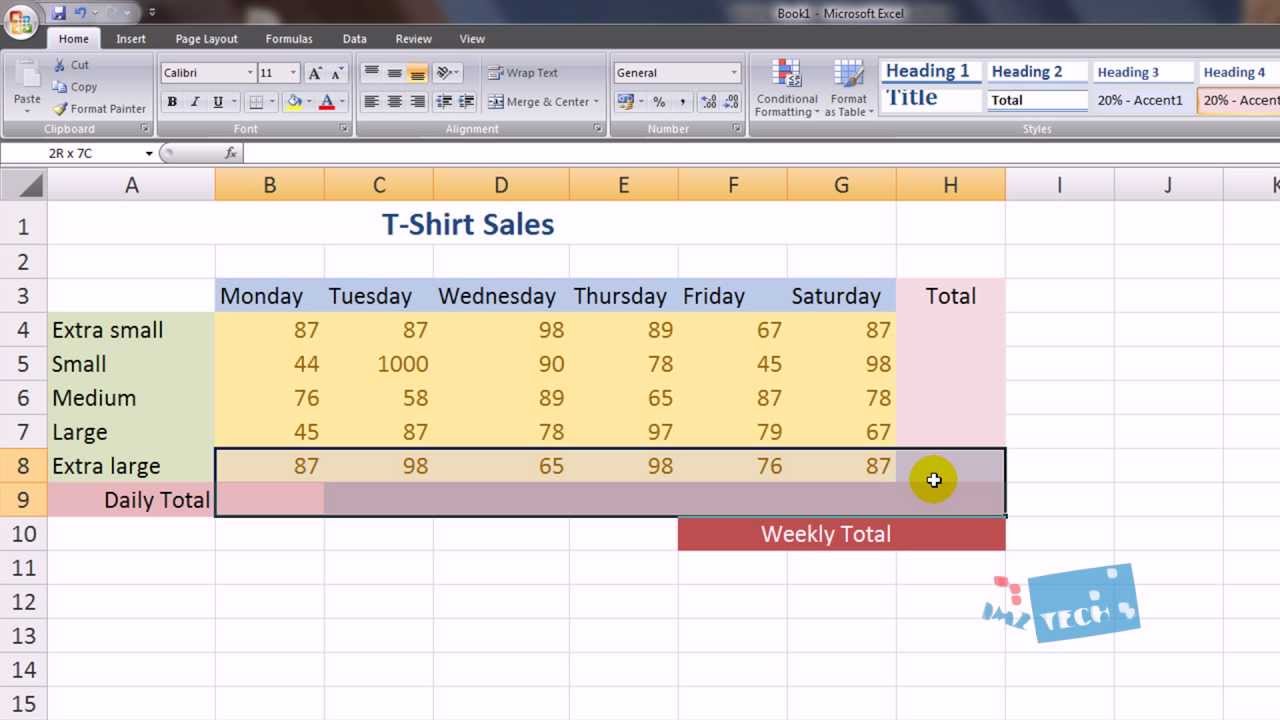
After analyzing your data, you might want to:
- Save Results: Use
save()
to save data for later use. - Write to Excel: Use
xlswrite()
to write results back into Excel for reporting or sharing.
new_data = your_analysis_results;
filename = ‘path_to_new_excel_file.xlsx’;
xlswrite(filename, new_data);
Throughout this journey, you've learned not just to read data from Excel but how to manipulate, analyze, and export it with MATLAB. These steps are foundational, but with practice, you can explore more complex operations like machine learning, signal processing, or advanced data visualization with MATLAB's extensive libraries.
Can MATLAB handle large Excel files efficiently?

+
Yes, MATLAB can handle large files, but performance may vary based on the dataset size and the computer’s specifications. For very large datasets, consider using memory-efficient techniques or segmenting the data.
What if my Excel data has errors or inconsistencies?

+
MATLAB offers various functions to clean data, like removing missing values or converting data types. You can manually clean the Excel file before importing or use MATLAB’s data manipulation capabilities.
Do I need Excel installed to use MATLAB with Excel files?

+
No, MATLAB has built-in capabilities to read and write Excel files without needing Excel installed, using the COM interface or by using third-party libraries like libxls or libxlsxwriter.