Extract Excel Date in Java: Step-by-Step Guide

Working with dates in Excel can sometimes be a tedious task, especially when you need to process or analyze that data programmatically. One common scenario in data handling is the need to extract and work with date values from Excel files using Java. This guide will walk you through the process of extracting dates from an Excel file using Java, ensuring you can automate and streamline your data management tasks efficiently.
Setting Up Your Java Environment

To begin, ensure you have the Java Development Kit (JDK) installed on your machine. Here are the steps:
- Download the latest JDK from the Oracle website if you haven’t already.
- Install and set up your PATH to include the bin directory of your JDK installation.
- Use an Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or even just a simple text editor paired with the command line.
Using Apache POI for Excel Reading

Apache POI is a powerful library for reading and writing Excel files in Java. Here’s how to get started:
- Add Apache POI Dependencies: If you’re using Maven, add these dependencies to your
pom.xml
:
org.apache.poi
poi
5.2.3
org.apache.poi
poi-ooxml
5.2.3
Reading the Excel File
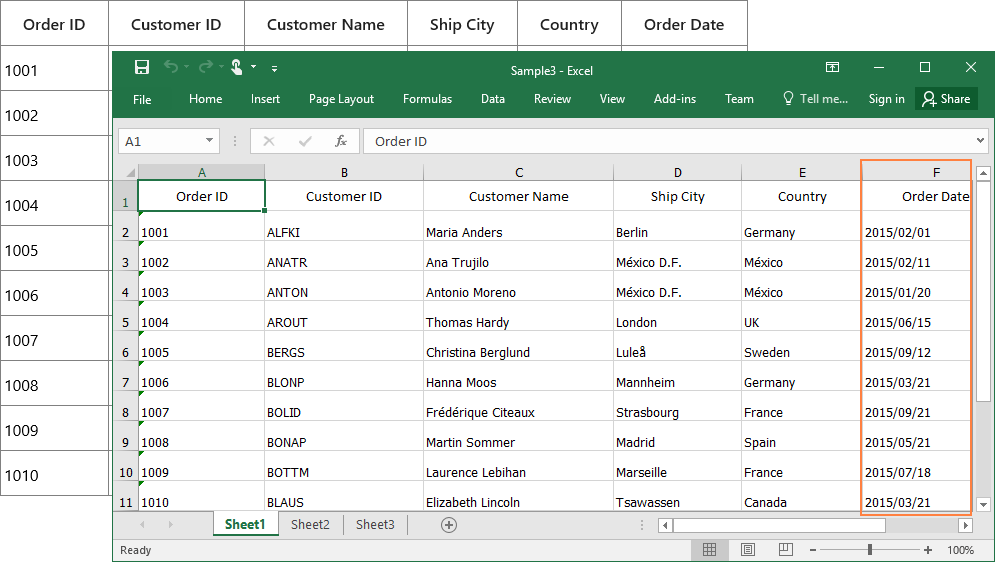
Let’s go through the steps to read an Excel file:
- Import Necessary Libraries
- Open the Excel File
- Iterate Through the Sheets, Rows, and Cells
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
FileInputStream excelFile = new FileInputStream(new File(“yourfile.xlsx”));
Workbook workbook = new XSSFWorkbook(excelFile);
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
for (Cell cell : row) {
if (cell.getCellType() == CellType.NUMERIC) {
if (DateUtil.isCellDateFormatted(cell)) {
Date date = cell.getDateCellValue();
System.out.println(“Date Value: ” + date);
} else {
System.out.println(“This cell is numeric but not a date.”);
}
}
}
}
💡 Note: Ensure the dates in your Excel file are actually formatted as dates. If they appear as text or other formats, you might need to preprocess or convert them within Excel itself or apply custom parsing logic in Java.
Handling Different Excel Formats

Excel files come in different formats like .xls and .xlsx:
- .xls (Excel 97-2003) uses HSSFWorkbook.
- .xlsx (Excel 2007 and later) uses XSSFWorkbook.
// For .xls files:
Workbook workbook = new HSSFWorkbook(excelFile);
// For .xlsx files:
Workbook workbook = new XSSFWorkbook(excelFile);
💡 Note: Apache POI provides universal Workbook interface, so after initializing with the correct workbook, your code for date extraction remains the same for both formats.
Formatting and Manipulating Date Values

Once you have extracted the date, you might want to format or manipulate it:
- Formatting the Date - Use Java’s
SimpleDateFormat
to format dates:
SimpleDateFormat sdf = new SimpleDateFormat(“MM-dd-yyyy”);
String formattedDate = sdf.format(cell.getDateCellValue());
java.time
package:
LocalDate localDate = cell.getDateCellValue().toInstant().atZone(ZoneId.systemDefault()).toLocalDate();
Conclusion

Extracting dates from an Excel file in Java can significantly enhance your data processing workflows. This guide covered the setup of your environment, the use of Apache POI to read Excel files, how to handle different Excel formats, and formatting extracted dates. By mastering these techniques, you can automate and streamline date extraction tasks, making data analysis and reporting much more efficient.
What if my dates are stored as text in Excel?

+
If dates are stored as text, you need to parse them manually or convert them into date format within Excel before processing with Java.
Can I extract date data from password-protected Excel files?

+
Yes, with Apache POI, you can use the POIFSFileSystem class to unlock the file before reading.
How do I handle time zones when extracting dates?

+
Java provides the ZoneId
class to handle time zones. Convert the date to a local date time with the appropriate zone if necessary.
What if the Excel file has multiple sheets with dates?

+
You can iterate over each sheet using a loop or read specific sheets by their index or name with workbook.getSheetAt(index)
or workbook.getSheet(name)
.