5 Ways to Retrieve Excel Sheet Names in C

Excel is a powerful tool for organizing data, but managing multiple sheets within one workbook can become cumbersome if you're not well-versed in its features or in programming with languages like C#. Here, we delve into 5 Ways to Retrieve Excel Sheet Names in C#, providing you with different techniques to interact with Excel spreadsheets programmatically. This knowledge is essential for developers aiming to automate Excel-related tasks, enhance productivity, or integrate Excel functionality into larger software solutions.
Method 1: Using COM Automation

COM (Component Object Model) Automation allows .NET applications to interact with Excel. Here’s how you can retrieve sheet names:
- Ensure Microsoft Excel is installed on your machine.
- Add a reference to "Microsoft.Office.Interop.Excel.dll" in your project.
- Use the following code to access the sheets:
using Excel = Microsoft.Office.Interop.Excel;
...
Excel.Application excelApp = new Excel.Application();
Excel.Workbook workbook = excelApp.Workbooks.Open(@"C:\path\to\your\file.xlsx");
foreach (Excel.Worksheet sheet in workbook.Worksheets)
{
Console.WriteLine(sheet.Name);
}
💡 Note: While COM automation provides direct access, it requires Excel to be installed on the server, which might not be the case in production environments. Additionally, it can be resource-intensive.
Method 2: OleDb Connection

You can retrieve sheet names by connecting to the Excel file through OleDb:
- Use this connection string format: "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=YourFilePath;Extended Properties=Excel 12.0"
- Execute a schema query to get sheet names:
string connectionString = @"Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\path\to\your\file.xlsx;Extended Properties='Excel 12.0;IMEX=1'";
using (OleDbConnection conn = new OleDbConnection(connectionString))
{
conn.Open();
DataTable dt = conn.GetSchema("Tables");
foreach (DataRow row in dt.Rows)
{
string sheetName = row["TABLE_NAME"].ToString();
Console.WriteLine(sheetName);
}
}
Method 3: Open XML SDK
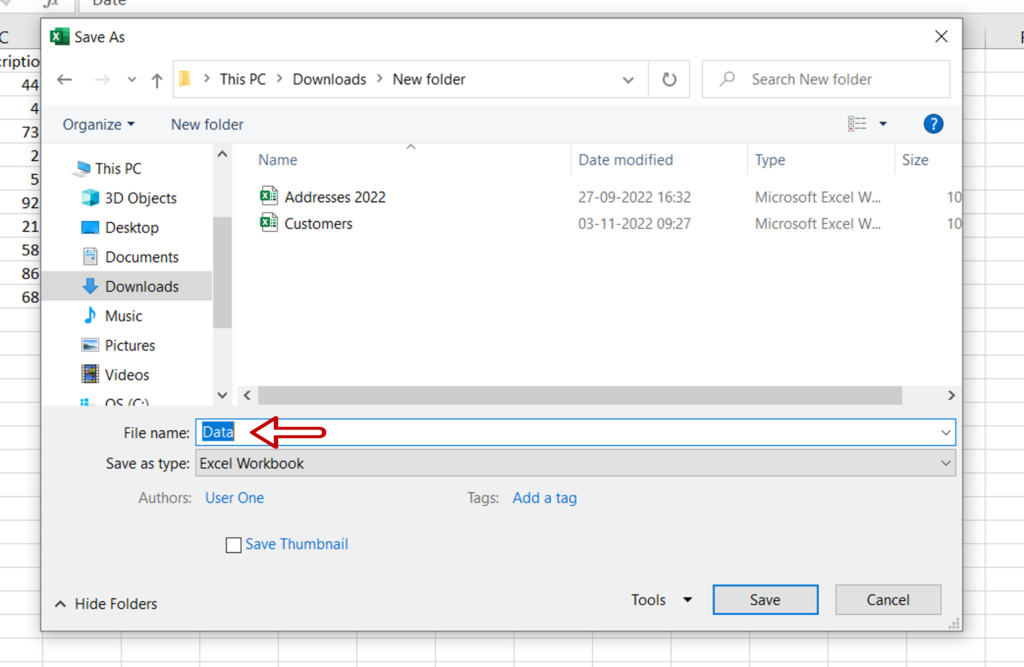
The Open XML SDK allows manipulation of Excel files without requiring Excel itself. Here’s how:
- Install the Open XML SDK package via NuGet.
- Parse the workbook.xml file within the Excel package:
using DocumentFormat.OpenXml.Packaging;
using DocumentFormat.OpenXml.Spreadsheet;
...
using (SpreadsheetDocument document = SpreadsheetDocument.Open(@"C:\path\to\your\file.xlsx", false))
{
WorkbookPart workbookPart = document.WorkbookPart;
foreach (Sheet sheet in workbookPart.Workbook.Sheets)
{
Console.WriteLine(sheet.Name);
}
}
🌟 Note: This method is useful for web applications or any environment where Excel might not be installed, but remember that Open XML does not support all Excel features.
Method 4: Using EPPlus

EPPlus is another library that simplifies Excel file manipulation without COM automation:
- Add EPPlus via NuGet.
- Use the following code to retrieve sheet names:
using OfficeOpenXml;
...
using (var package = new ExcelPackage(new FileInfo(@"C:\path\to\your\file.xlsx")))
{
foreach (var worksheet in package.Workbook.Worksheets)
{
Console.WriteLine(worksheet.Name);
}
}
Method 5: Using ExcelDataReader

For those looking for a fast, lightweight option:
- Include ExcelDataReader through NuGet.
- Access sheet names like this:
using ExcelDataReader;
...
using (var stream = File.Open(@"C:\path\to\your\file.xlsx", FileMode.Open, FileAccess.Read))
{
using (var reader = ExcelReaderFactory.CreateReader(stream))
{
var result = reader.AsDataSet();
foreach (DataTable table in result.Tables)
{
Console.WriteLine(table.TableName);
}
}
}
By exploring these methods, developers can choose the one that best suits their project requirements, whether it be for performance, compatibility, or functionality. Each method has its own advantages:
- COM Automation is straightforward but resource-heavy.
- OleDb can be used in environments where Excel isn't installed.
- Open XML SDK and EPPlus provide better performance in web applications.
- ExcelDataReader is lightweight and works well for simple data extraction.
These techniques not only help in retrieving sheet names but can also be expanded for further manipulation and analysis of Excel data. Whether you're building a tool to process Excel files or integrating Excel functionalities into a larger application, knowing how to interact with spreadsheets programmatically is crucial in today's data-driven environment.
It's also worth considering the maintenance aspect; methods that don't require Excel to be installed might offer better long-term support as Microsoft continues to evolve Excel's internal architecture. Additionally, while these methods focus on retrieving sheet names, they can be adapted for reading, writing, or modifying data, making your applications more versatile and powerful.
By understanding these approaches, you're equipped to handle Excel files in various scenarios, enhancing your software's capabilities and your productivity as a developer.
Can I use these methods in a production environment?

+
Yes, most of these methods are suitable for production, but some considerations apply: COM Automation requires Excel to be installed, which might not be ideal for server environments. Methods like Open XML SDK or EPPlus are better for web applications since they don’t require Excel.
Do I need Excel installed for these methods?

+
No, except for COM Automation. Methods like OleDb, Open XML SDK, EPPlus, and ExcelDataReader can work without Excel installed, making them suitable for environments where Excel isn’t available or feasible.
Which method is best for performance?

+
For performance, Open XML SDK or EPPlus are usually the best due to their lightweight nature and direct XML manipulation. However, performance can also depend on the specifics of your application and the size of the Excel files being processed.
Are there any licensing concerns with these libraries?

+
Most of the libraries mentioned here are either open-source or Microsoft’s own tools. EPPlus, for instance, was open-source but now requires a commercial license for some uses. Always check the licensing terms for the specific version you’re using.
Can these methods handle password-protected Excel files?

+
Not all methods support password-protected files out of the box. For instance, Open XML SDK can open encrypted files if you provide the correct password, but COM Automation can handle this natively as it interacts directly with Excel.