Mastering Excel: How to Use VBA to Enter Formulas Easily

When it comes to maximizing the capabilities of Excel, few tools offer as much power and flexibility as VBA (Visual Basic for Applications). Excel VBA allows users to automate repetitive tasks, manipulate large datasets, and significantly enhance productivity. One of the most useful applications of VBA in Excel is simplifying the process of entering formulas into spreadsheets. This comprehensive guide will delve into how you can use VBA to enter formulas easily, streamline your workflow, and save considerable time.
The Power of VBA in Excel

Before we delve into the specifics of entering formulas with VBA, let’s briefly discuss why VBA is a game changer for Excel users:
- Automation: VBA can automate any sequence of commands, saving you from manual labor.
- Custom Functions: Create user-defined functions (UDFs) for unique calculations not available in standard Excel functions.
- Interactive and Dynamic Worksheets: VBA allows for dynamic and interactive worksheets that respond to user input in real-time.
- Error Handling: Robust error handling to manage unexpected scenarios during automation.
Basics of VBA in Excel

VBA can be accessed through Excel’s Developer tab, which might need to be enabled:
- Go to File > Options > Customize Ribbon, check Developer, and click OK.
- Once enabled, click on the Developer tab, then select Visual Basic or press Alt + F11.
Entering Formulas with VBA

Entering formulas into Excel using VBA involves understanding how to write a macro that can dynamically input formulas. Here are the steps to follow:
1. Launch the VBA Editor

Open the VBA editor as described above or use the shortcut Alt + F11.
2. Insert a Module

In the VBA Editor, right-click on any of your workbook’s VBAProject in the Project Explorer, select Insert, and then Module. This is where you’ll write your code.
3. Writing the VBA Code for Formula Entry

Here’s an example of how to use VBA to enter a simple sum formula into cell A1:
Sub EnterSumFormula()
‘Set the formula for cell A1
Range(“A1”).Formula = “=SUM(B1:B10)”
End Sub
✏️ Note: This code assumes your data for summing is in cells B1 to B10. Adjust the range as needed.
4. Expanding Your Knowledge

VBA can be used to enter more complex formulas:
Sub EnterComplexFormula()
‘Set a complex formula for cell A2
Range(“A2”).Formula = “=IF(A1>100,A1*0.9,A1*0.95)”
End Sub
Advanced Techniques

As you become more comfortable with VBA, here are some advanced techniques you can explore:
Dynamic Formulas with Variables
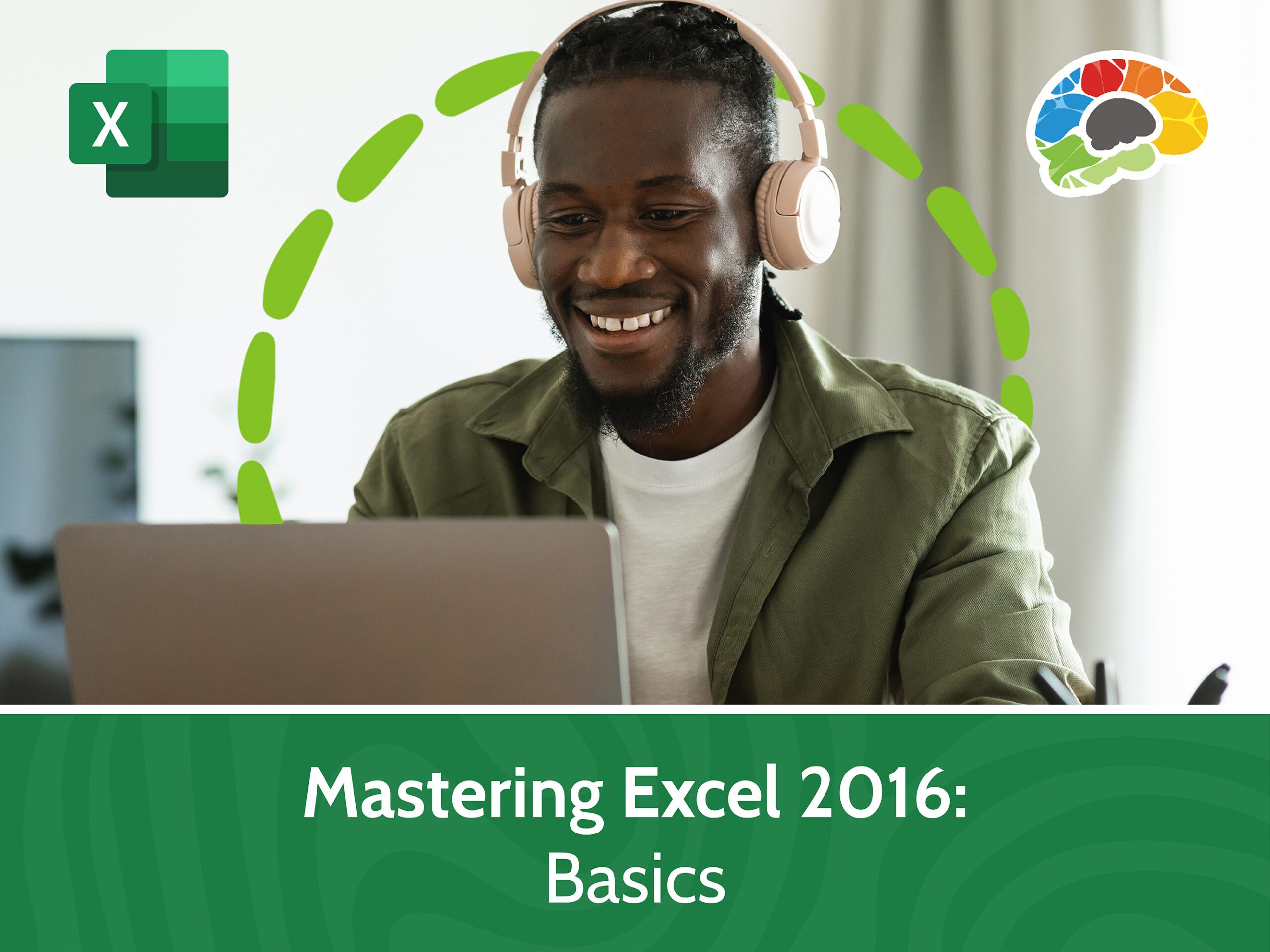
By using variables in your VBA code, you can dynamically change formulas:
Sub DynamicFormula()
Dim endRange As Integer
endRange = InputBox(“Enter the end row for the sum formula:”)
Cells(1, 1).Formula = “=SUM(B1:B” & endRange & “)”
End Sub
Using Arrays for Formula Entry

Entering formulas across a range of cells efficiently:
Sub ArrayFormulaEntry()
Dim formulas() As Variant
formulas = Array(“=A1+1”, “=A2+1”, “=A3+1”)
For i = LBound(formulas) To UBound(formulas)
Cells(i + 1, 1).Formula = formulas(i)
Next i
End Sub
Interacting with Excel Objects

Using VBA to interact with Excel objects like charts, pivot tables, and so on, can further extend your automation capabilities:
- Create charts from data dynamically.
- Update pivot tables with new data ranges or change their settings.
- Interact with other Excel features like filtering or data validation rules.
Handling Errors

Here’s how you can handle errors when entering formulas:
Sub ErrorHandlingFormula()
On Error GoTo ErrorHandler
Range(“A1”).Formula = “=SUM(B1:B10)”
Exit Sub
ErrorHandler:
MsgBox “An error occurred: ” & Err.Description
End Sub
Best Practices

When using VBA for formula entry, consider these best practices:
- Use meaningful variable names: This makes your code more readable.
- Comment your code: Comments should explain why something is done, not what is done.
- Test in small batches: Start with small sections of your workbook to ensure your formulas are correct.
- Backup your workbook: VBA changes can be detrimental if they go wrong, so always have backups.
As we wrap up our guide to using VBA for formula entry in Excel, remember that practice is key. Start by automating simple tasks, then gradually incorporate more complex formulas and functionality. By mastering VBA, you're not just improving your Excel skills; you're opening up a world of automation and efficiency that can save you hours of manual work. The knowledge you've gained here will empower you to tackle more intricate data management challenges with confidence, making your Excel experience significantly more productive and satisfying.
Can VBA change formulas dynamically?

+
Yes, VBA can be programmed to dynamically change formulas based on user input or specific conditions. For example, you can write a VBA script that adjusts the range of a formula depending on the number of rows of data available.
How do I ensure my VBA formulas are not overwritten by other users?

+
To protect your VBA formulas from being accidentally altered, you can lock the cells containing the VBA-generated formulas. Go to the Protection tab in Excel’s Format Cells dialog to enable this feature.
What are the limitations of using VBA for formula entry?
+
VBA is powerful but has limitations. It can slow down Excel for very complex operations, there’s a learning curve, and complex scripts can become hard to maintain over time. Also, some Excel features might not be fully compatible with VBA.