Excel VBA: Create Hyperlinks Between Sheets Easily

Introduction to Hyperlinking in Excel VBA

Excel, with its powerful computation and data analysis capabilities, often serves as a cornerstone for businesses and individual users alike. One of the lesser-known but incredibly useful features of Excel is the ability to create hyperlinks between sheets using VBA (Visual Basic for Applications). This article delves into how you can automate hyperlink creation, enhancing navigation and usability in complex spreadsheets.
💡 Note: This guide assumes you have basic knowledge of Excel and a willingness to learn VBA coding.
Understanding Hyperlinks in Excel

Before diving into VBA, let’s understand what hyperlinks are in the context of Excel:
- Hyperlinks allow you to connect cells or text to websites, documents, emails, or different locations within the same Excel workbook.
- They make navigation easier by providing clickable links, which is particularly useful when dealing with large workbooks spread across multiple sheets.
The Basics of Excel VBA for Hyperlinks

VBA is the programming language behind Excel macros, enabling automation of repetitive tasks. Here’s how you can get started with VBA for hyperlinks:
Setting Up VBA

- Open Excel VBA Editor: Press
ALT + F11
to access the VBA editor. - Insert a New Module: Right-click on any of the objects in the left pane, select ‘Insert’, and then ‘Module’. This will open a new window where you can write your code.
Sub AddHyperlink()
Dim wsSource As Worksheet
Dim wsDest As Worksheet
Dim cell As Range
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsDest = ThisWorkbook.Sheets("Sheet2")
For Each cell In wsSource.Range("A1:A10")
If Not IsEmpty(cell) Then
cell.Hyperlinks.Add Anchor:=cell, _
Address:="", _
SubAddress:=wsDest.Name & "!" & cell.Offset(0, 1).Address, _
ScreenTip:="Go to " & wsDest.Name, _
TextToDisplay:=cell.Value
End If
Next cell
End Sub
💡 Note: Replace `"Sheet1"`, `"Sheet2"`, and the range `"A1:A10"` with your actual sheet names and cell ranges.
Automating Hyperlink Creation

Let’s walk through the process of creating hyperlinks automatically using VBA:
Step-by-Step Guide

Access the VBA Editor: As described earlier, use
ALT + F11
.Write the VBA Code:
- Create a new subroutine with
Sub AddHyperlink()
- Use
Dim
statements to declare variables for source and destination worksheets, and the range to work on. - Loop through cells in the source range, checking for non-empty cells.
- Create a new subroutine with
Sub AddHyperlink()
Dim wsSource As Worksheet
Dim wsDest As Worksheet
Dim cell As Range
Dim rngHyperlinks As Range
Set wsSource = ThisWorkbook.Sheets("Sheet1")
Set wsDest = ThisWorkbook.Sheets("Sheet2")
Set rngHyperlinks = wsSource.Range("A1:A10")
For Each cell In rngHyperlinks
If Not IsEmpty(cell) Then
cell.Hyperlinks.Add Anchor:=cell, _
Address:="", _
SubAddress:=wsDest.Name & "!" & cell.Offset(0, 1).Address, _
ScreenTip:="Go to " & wsDest.Name, _
TextToDisplay:=cell.Value
End If
Next cell
End Sub
- Run the Macro: Execute the macro by pressing
F5
or running it from the Excel ribbon.
💡 Note: Ensure you save your work as a Macro-Enabled Workbook (.xlsm) to run VBA code.
Enhancing Hyperlink Functionality

- Dynamic Hyperlinks: Instead of hardcoding sheet names or cell references, use variables to make your code flexible.
- Error Handling: Incorporate error handling to manage scenarios like missing sheets or cells.
Sub AddDynamicHyperlinks()
Dim wsSource As Worksheet, wsDest As Worksheet
Dim rngSource As Range, cell As Range
Dim linkName As String, linkDest As String
On Error GoTo ErrorHandler
linkName = "Sheet1"
linkDest = "Sheet2"
Set wsSource = ThisWorkbook.Sheets(linkName)
Set wsDest = ThisWorkbook.Sheets(linkDest)
Set rngSource = wsSource.Range("A1:A10")
For Each cell In rngSource
If Not IsEmpty(cell) Then
cell.Hyperlinks.Add Anchor:=cell, _
Address:="", _
SubAddress:=linkDest & "!" & cell.Offset(0, 1).Address, _
ScreenTip:="Go to " & linkDest, _
TextToDisplay:=cell.Value
End If
Next cell
Exit Sub
ErrorHandler:
MsgBox "Error: " & Err.Description, vbCritical
End Sub
Customizing Hyperlinks

You can customize hyperlinks with different text, tooltips, or even automate different navigation paths:
- Text to Display: Set the
TextToDisplay
parameter to customize what the user sees as the link. - ScreenTips: Use
ScreenTip
to provide additional information when hovering over the hyperlink.
Practical Applications and Examples

Here are some practical scenarios where VBA-generated hyperlinks can streamline your work:
- Automated Navigation in Large Financial Models: For complex financial models with numerous sheets, creating dynamic hyperlinks can simplify navigation.
- Document Management: Use hyperlinks to manage and navigate between related documents or different sections within a workbook.
- Interactive Reports: Allow users to jump between summary and detailed data sheets with ease.
Advanced Hyperlinking Techniques

Creating Hyperlinks to External Locations

- VBA can also create hyperlinks to external files or web addresses:
Sub CreateExternalLink()
Dim ws As Worksheet, rng As Range
Set ws = ThisWorkbook.Sheets("Sheet1")
Set rng = ws.Range("A1")
rng.Hyperlinks.Add Anchor:=rng, _
Address:="https://www.example.com", _
TextToDisplay:="Go to Example", _
ScreenTip:="Visit our website"
End Sub
Interactive Hyperlink Management

You might want to add features like:
- Delete Hyperlinks: Provide a function to remove hyperlinks from selected cells.
Sub RemoveHyperlinks()
Dim cell As Range
For Each cell In Selection
If cell.Hyperlinks.Count > 0 Then
cell.Hyperlinks.Delete
End If
Next cell
End Sub
Using Hyperlinks for Navigation Flow

Creating a structured navigation system using VBA can enhance the user experience:
Sub SetupHyperlinks()
Dim shSource As Worksheet, shDest As Worksheet
Set shSource = ThisWorkbook.Sheets("Overview")
Set shDest = ThisWorkbook.Sheets("Details")
shSource.Hyperlinks.Add Anchor:=shSource.Range("A1"), _
Address:="", _
SubAddress:="'" & shDest.Name & "'!A1", _
TextToDisplay:="View Details"
shDest.Hyperlinks.Add Anchor:=shDest.Range("A1"), _
Address:="", _
SubAddress:="'" & shSource.Name & "'!A1", _
TextToDisplay:="Back to Overview"
End Sub
The ability to automate hyperlink creation in Excel using VBA provides a significant productivity boost. It turns static spreadsheets into dynamic, interactive tools, enhancing usability, navigation, and data management. From simple navigation aids to complex interactive reports, VBA hyperlinks can transform how you interact with your Excel data.
Next Steps:
As you delve deeper into Excel VBA, consider exploring more advanced features like conditional hyperlinks, which respond based on data or user input, or creating a custom dialog box to manage hyperlinks in your workbook.
Remember to:
- Test Your Macros: Always test your VBA code on a sample workbook to avoid unintentional changes to important data.
- Keep Your VBA Code Clean: Comment your code for clarity, and keep it organized for easier future modifications.
- Learn More: Excel VBA has a vast ecosystem. Keep learning and experimenting to harness its full potential.
What are the benefits of using VBA for hyperlinks?

+
Using VBA to create hyperlinks automates navigation within complex workbooks, saving time and reducing errors from manual hyperlink creation. It also allows for dynamic and conditional hyperlink behavior, making spreadsheets more interactive and user-friendly.
Can VBA create hyperlinks to external websites or files?
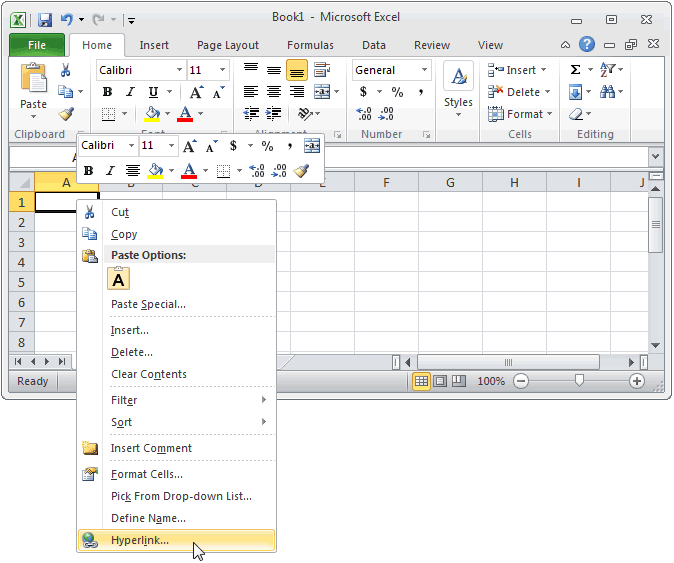
+
Yes, VBA can create hyperlinks to external websites, documents, or other locations outside the workbook, offering great flexibility in document management and data linking.
How can I prevent errors when using VBA for hyperlink creation?

+
Implement error handling in your VBA code to catch and manage exceptions. Always verify the existence of sheets or cells before adding hyperlinks and use On Error
statements to provide user-friendly error messages or log errors for debugging.