Create Excel Sheets in Android Studio: A Simple Guide

In the digital age, the ability to create and manage spreadsheets has become essential for numerous tasks, ranging from personal finance tracking to data analysis for businesses. While most of us are familiar with using spreadsheets on traditional platforms like desktops, the need to perform these tasks on mobile devices has never been greater. Android, being the most widely used mobile operating system, has seen a surge in demand for apps that can handle Excel functionality. This guide will walk you through the steps of creating Excel sheets in Android Studio, helping you tap into this demand.
Setting Up Your Environment

Before diving into the coding process, you need to prepare your development environment:
- Ensure you have Android Studio installed. If not, download and install it from the official Android website.
- Verify that Java Development Kit (JDK) is installed as Android Studio requires Java to run.
- Optional but recommended: Set up an Android Virtual Device (AVD) for testing your app.
Starting Your Project
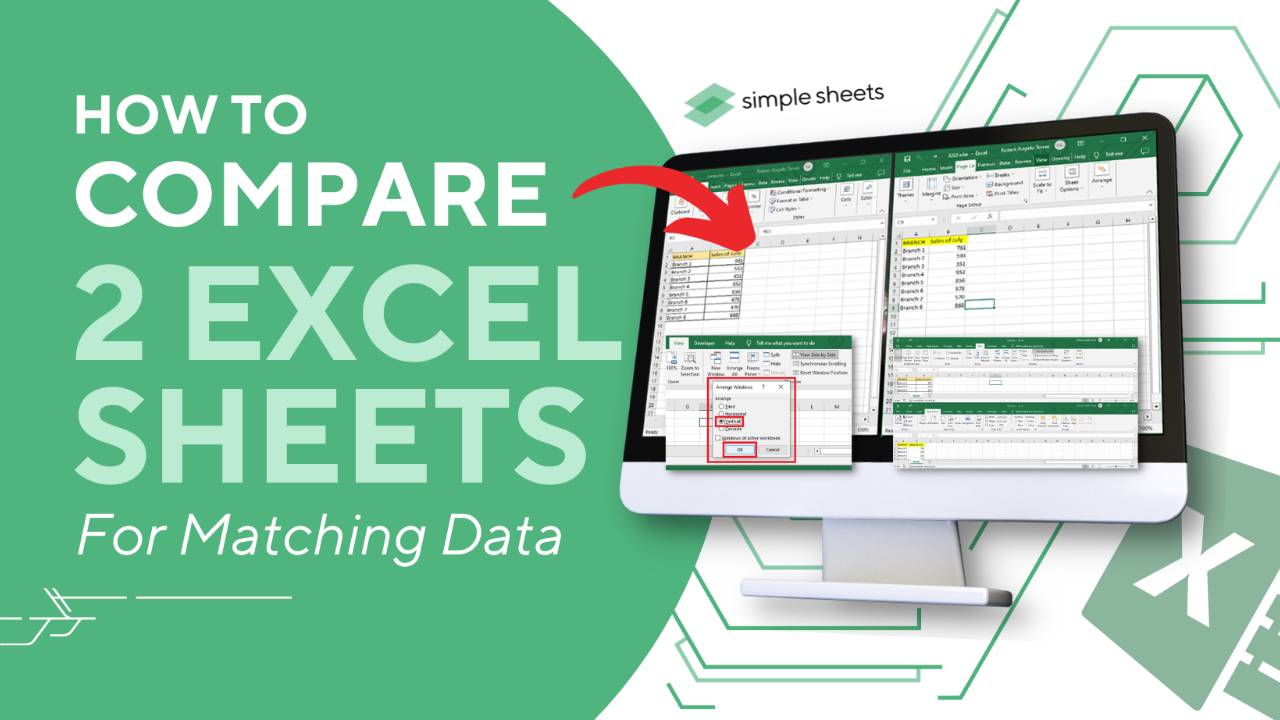
Here’s how you can start a new project in Android Studio to create an app for Excel sheet manipulation:
- Launch Android Studio.
- Click on “Start a new Android Studio project”.
- Select “Empty Activity” and proceed with the setup wizard, naming your project something like “ExcelSheetApp”.
Adding Dependencies

To handle Excel files, you need to integrate a library that can read and write Excel files. The most popular choice is Apache POI, but since it’s heavy for mobile, consider using lighter alternatives like:
- JXLS
- XlsxWriter - This library, however, focuses only on writing to Excel files.
Add these dependencies in your build.gradle (Module: app) file:
dependencies {
implementation ‘com.github.mukeshsolanki:excella:1.2’
// If you choose JXLS:
implementation ‘org.jxls:jxls-poi:2.8.1’
}
Don’t forget to sync your project after adding dependencies.
Creating a User Interface

Design a simple UI for entering data and displaying an Excel sheet preview:
- Open the activity_main.xml file.
- Add EditText fields for data input.
- Include a Button for exporting data to Excel.
- Optionally, add a WebView or RecyclerView to show Excel data.
Here’s an example layout:
<EditText android:id="@+id/etName" android:hint="Enter Name" ... /> <EditText android:id="@+id/etAge" android:hint="Enter Age" ... /> <Button android:id="@+id/btnExport" android:text="Export to Excel" ... />
Writing Excel Data
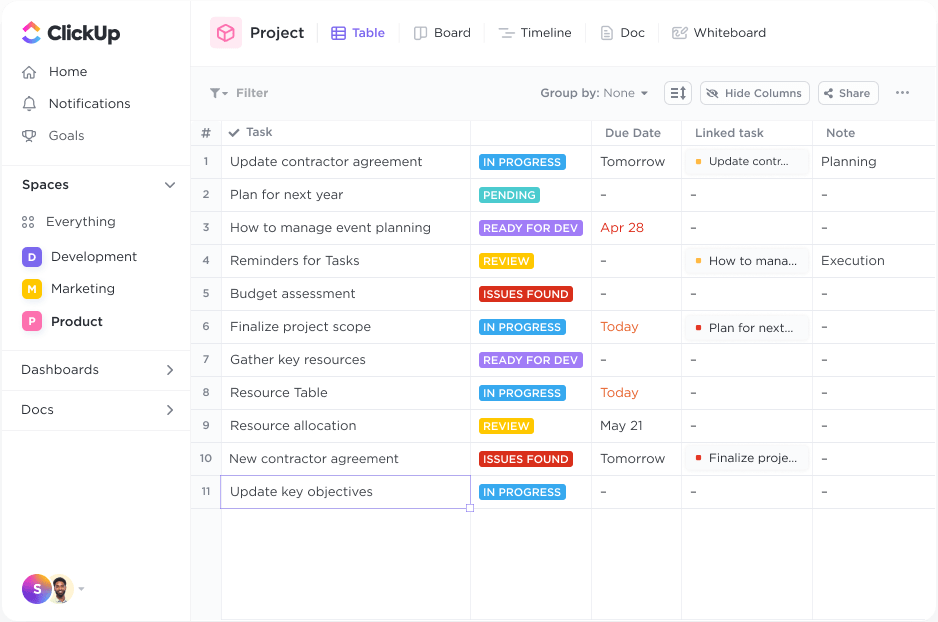
After setting up your UI, you’ll need to write the code to export this data into an Excel sheet:
- Create an ExcelUtil class or include necessary functions in your MainActivity.
- Implement methods to write data to an Excel file using the library you’ve added.
Here’s a basic example:
public void exportToExcel(View view) { EditText nameET = findViewById(R.id.etName); EditText ageET = findViewById(R.id.etAge);
String name = nameET.getText().toString(); int age = Integer.parseInt(ageET.getText().toString()); ExcelUtils.createExcelAndSave(context, "personnel", new String[]{"Name", "Age"}, new Object[][]{{name, age}});
}
💡 Note: Error handling and checks for empty inputs are essential for real-world applications.
Permissions

Since you’ll be writing files to the device storage, you need to request the necessary permissions:
- Add WRITE_EXTERNAL_STORAGE permission to your AndroidManifest.xml.
- Implement runtime permission requests if targeting Android 6.0 (API level 23) and above.
Summary

In this guide, we’ve explored how to set up an Android Studio project, integrate libraries for Excel functionality, design a user interface for data input, and write the code to export this data into an Excel sheet. By following these steps, you can create an app that performs Excel-related tasks on Android devices. This capability not only caters to the needs of individuals but also businesses looking to manage data on the go. The flexibility and portability of handling spreadsheets on Android devices open up numerous possibilities for productivity and data management.
Can I edit Excel files directly in Android Studio?

+
While you can write code to edit Excel files within an Android app, Android Studio itself does not have built-in functionality to directly edit Excel files. You’ll need to use an external library to manipulate the data.
Is there an alternative to Apache POI for lightweight Excel processing?

+
Yes, JXLS and XlsxWriter are popular lightweight alternatives that offer basic Excel processing capabilities which are more suited for mobile environments.
Do I need any permissions to write Excel files?

+
Yes, to write files to external storage, your app needs the WRITE_EXTERNAL_STORAGE permission, which must be declared in the AndroidManifest.xml. For Android 6.0+, you also need to request this permission at runtime.