Mastering Excel VBA: Count Sheets Easily

Excel, a powerhouse within the Microsoft Office suite, is renowned for its robust capabilities in data management, analysis, and visualization. However, many users leverage just a fraction of what Excel can offer. Visual Basic for Applications (VBA), Excel's programming language, significantly expands its functionality, turning routine tasks into simple, one-click operations. One such task that often requires automation is counting the number of sheets in an Excel workbook, especially for those managing large datasets or complex workbooks with numerous tabs. In this comprehensive guide, we'll explore how to master Excel VBA to count sheets effortlessly, ensuring efficiency and accuracy in your work.
Understanding VBA in Excel

VBA, short for Visual Basic for Applications, is an event-driven programming language from Microsoft that is built into most of its Office applications. In Excel, VBA allows for:
- Automation of repetitive tasks
- Complex data manipulation and analysis
- Dynamic report generation
- Custom function creation
💡 Note: Before diving into VBA, make sure you have the Developer tab enabled in Excel to access the VBA editor easily.
Why Use VBA to Count Sheets?

When working with large Excel files or those that are constantly updated with new sheets:
- Automation: VBA scripts can automate the process, reducing manual errors and time.
- Accuracy: Scripts ensure no sheets are missed or counted more than once, which is common in manual counting.
- Integration: The script can be part of a larger automation effort, perhaps feeding the count into other VBA-driven processes or reports.
Counting Sheets with VBA: A Step-by-Step Guide

Accessing the VBA Editor

To begin:
- Press Alt + F11 to open the VBA editor.
- In the Project Explorer, right-click on any of your workbook’s objects (e.g., “ThisWorkbook”) and choose “Insert” > “Module”.
🎯 Note: “ThisWorkbook” refers to the active Excel file where the VBA code is being written.
The Code

Here is a simple VBA script to count the number of sheets:
Sub CountSheets()
Dim sheetCount As Integer
sheetCount = ActiveWorkbook.Worksheets.Count
MsgBox “The workbook contains ” & sheetCount & “ sheets.”
End Sub
Execution

- Place the cursor anywhere within the macro code.
- Press F5 or click the “Run” button on the toolbar to execute the macro.
A message box will display the number of sheets in your workbook.
Enhancing Your VBA Sheet Counting Script

Counting Specific Sheet Types

If you’re interested in counting only visible sheets, hidden sheets, or specific sheet types (like charts):
Sub CountSpecificSheets() Dim ws As Worksheet Dim visibleCount As Integer, hiddenCount As Integer, chartCount As Integer
visibleCount = 0 hiddenCount = 0 chartCount = 0 For Each ws In ActiveWorkbook.Worksheets If ws.Visible = xlSheetVisible Then visibleCount = visibleCount + 1 ElseIf ws.Visible = xlSheetHidden Or ws.Visible = xlSheetVeryHidden Then hiddenCount = hiddenCount + 1 End If Next ws For Each Sheet In ActiveWorkbook.Sheets If TypeName(Sheet) = "Chart" Then chartCount = chartCount + 1 End If Next Sheet MsgBox "Visible Sheets: " & visibleCount & vbNewLine & _ "Hidden Sheets: " & hiddenCount & vbNewLine & _ "Chart Sheets: " & chartCount & vbNewLine & _ "Total Sheets: " & ActiveWorkbook.Sheets.Count
End Sub
Tips for Efficient VBA Scripts

- Organize Your Code: Use modules to segregate different functions, making your VBA project more manageable.
- Error Handling: Incorporate error handling to manage unexpected errors gracefully.
- Comment Your Code: Good comments improve code readability and maintainability.
- Optimization: Use ‘With’ blocks or collections to optimize code for speed.
🌐 Note: Consider using ‘With’ blocks for member properties access within objects for a cleaner and potentially faster script.
To sum up, mastering VBA to count sheets in Excel not only saves time but also significantly reduces the chances of human error. With the right knowledge, you can automate tasks that would otherwise consume hours of your day. This guide has walked you through the essentials of counting sheets using VBA, from the basics to more advanced techniques. Remember, while VBA can transform your Excel experience, always test your macros in a non-critical environment to ensure they perform as intended before using them on real work data.
Can I count sheets in a closed workbook?

+
VBA can’t directly count sheets in a closed workbook. However, you can retrieve some information by using an external function or by creating a custom function in another language like Python to interact with closed Excel files.
How can I add a button to run my macro?
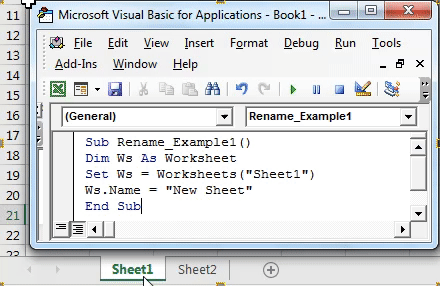
+
You can add a button from the Developer tab in Excel. Choose “Insert”, then “Button” under “Form Controls”, draw the button on your sheet, and assign your macro to it when prompted.
Is there a way to prevent users from running certain macros?

+
Yes, you can secure your VBA macros by password-protecting the VBA project or by using workbook-level security settings to restrict access to macros.