5 Ways to Arrange Sheets in Excel with VBA

Using Visual Basic for Applications (VBA) in Excel can transform how you handle and organize your spreadsheets, making tasks like sheet arrangement effortless and precise. Here are five effective methods to arrange your sheets using VBA:
1. Alphabetize Sheets

Often, organizing sheets in alphabetical order can significantly improve navigation. Here is a VBA script that will automatically sort your sheets:
Sub SortSheetsAlphabetically() Dim ws As Worksheet Dim i As Integer, j As Integer Application.ScreenUpdating = False
For i = 1 To Sheets.Count - 1 For j = i + 1 To Sheets.Count If LCase(Sheets(j).Name) < LCase(Sheets(i).Name) Then Sheets(j).Move Before:=Sheets(i) End If Next j Next i Application.ScreenUpdating = True
End Sub
This script works by comparing sheet names case-insensitively and moving sheets when they’re out of order.
💡 Note: This method might fail if the sheet names are very similar or if leading/trailing spaces exist.
2. Move to a Specific Position

If you know exactly where you want a sheet to be, use this code:
Sub MoveSheetToPosition()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets(“Sheet1”)
ws.Move Before:=ThisWorkbook.Sheets(3) ‘Move sheet to be the third from the left
End Sub
- Replace “Sheet1” with your target sheet’s name.
- Modify the number in
Sheets(3)
to where you want the sheet to appear.
3. Rearrange Based on Content

You might want to arrange sheets based on data in a particular cell for better categorization:
Sub ArrangeSheetsByContent() Dim ws As Worksheet Dim rng As Range, cell As Range Dim sortArr() As Variant Dim i As Integer
ReDim sortArr(1 To Sheets.Count) i = 1 For Each ws In ThisWorkbook.Worksheets sortArr(i) = ws.Range("A1").Value 'Change A1 to whatever cell you're checking i = i + 1 Next ws For i = 1 To Sheets.Count - 1 For j = i + 1 To Sheets.Count If sortArr(i) > sortArr(j) Then ThisWorkbook.Sheets(i).Move After:=ThisWorkbook.Sheets(j) 'Swap array elements temp = sortArr(i) sortArr(i) = sortArr(j) sortArr(j) = temp End If Next j Next i
End Sub
4. Arrange Sheets in Chronological Order

For spreadsheets where dates or time-based data matters, arrange sheets according to chronological order:
Sub ArrangeSheetsByDate() Dim ws As Worksheet Dim dtArr() As Date Dim i As Integer, j As Integer
ReDim dtArr(1 To Sheets.Count) i = 1 For Each ws In ThisWorkbook.Worksheets 'Assumes the date is in A1 of each sheet, change as needed dtArr(i) = CDate(ws.Range("A1").Value) i = i + 1 Next ws For i = 1 To Sheets.Count - 1 For j = i + 1 To Sheets.Count If dtArr(i) > dtArr(j) Then ThisWorkbook.Sheets(j).Move Before:=ThisWorkbook.Sheets(i) 'Swap dates in array tempDate = dtArr(i) dtArr(i) = dtArr(j) dtArr(j) = tempDate End If Next j Next i
End Sub
5. Custom Sorting with User-Defined Order
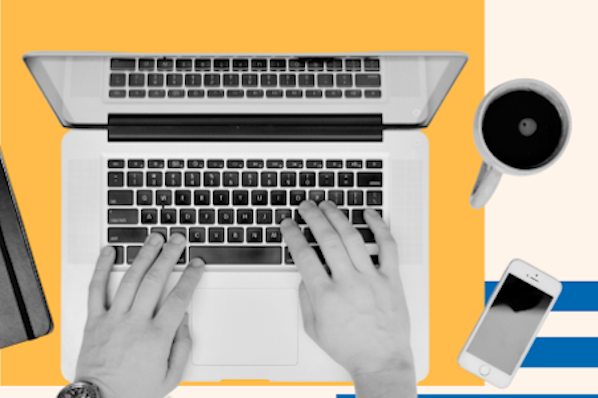
Create a custom sorting algorithm where users can define the exact order:
Sub CustomSheetSort() Dim userInput As Variant Dim ws As Worksheet Dim i As Integer
userInput = InputBox("Enter the sheet names separated by commas, in the order you want them to appear.") If userInput = "" Then Exit Sub ' Exit if no input is provided ' Split the input string into an array Dim sheetNames() As String sheetNames = Split(userInput, ",") For i = LBound(sheetNames) To UBound(sheetNames) On Error Resume Next Set ws = Sheets(Trim(sheetNames(i))) If Not ws Is Nothing Then ws.Move After:=Sheets(Sheets.Count) Else MsgBox "Sheet '" & sheetNames(i) & "' not found!", vbExclamation, "Error" End If On Error GoTo 0 Next i
End Sub
💡 Note: Use this method when the sheet arrangement should follow a specific sequence that you control.
In summary, arranging sheets in Excel with VBA can significantly streamline your data management tasks. Whether you're looking to alphabetize, sort by content or date, place sheets in a specific order, or customize the arrangement, these scripts provide versatile tools to enhance your productivity. VBA's automation capabilities allow for the creation of more efficient workflows, enabling you to focus on analysis rather than organization.
Why should I use VBA to sort Excel sheets?

+
VBA offers automation which can save time on repetitive tasks like sorting sheets, especially when dealing with large spreadsheets.
Can these VBA scripts be adapted for other sorting methods?

+
Yes, you can modify these scripts or create new ones tailored to your specific sorting needs within the constraints of VBA’s functionality.
Are there any security concerns with running VBA macros?

+
Yes, macros can execute code which might pose security risks. Ensure you understand the code or come from a trusted source, and always enable macros from trusted locations.