Adding Data to Excel with Java: Quick and Easy

If you are working with Java applications that require spreadsheet functionality, integrating Microsoft Excel for data manipulation or storage can significantly enhance productivity. This guide will introduce you to the basics of adding data to Excel spreadsheets using Java, providing a seamless way to manage and analyze data.
Setting Up Your Environment

Before you start writing code, ensure you have the following prerequisites:
- An Integrated Development Environment (IDE) like Eclipse, IntelliJ IDEA, or any Java-compatible editor.
- The Java Development Kit (JDK) installed, compatible with your system.
- Microsoft Excel installed on your machine, though not necessary for all methods.
- A Java library for Excel manipulation, such as Apache POI or JExcelApi.
💡 Note: Apache POI is widely used due to its extensive support for Excel features, while JExcelApi is simpler and faster for basic operations.
Using Apache POI

Adding Apache POI to Your Project

To use Apache POI, you need to add the library to your project. Here’s how you can do it:
- Download the POI jar files from the Apache POI website or use a dependency management tool like Maven or Gradle:
- Maven: Add these dependencies to your pom.xml file:
org.apache.poi poi 5.0.0 org.apache.poi poi-ooxml 5.0.0 - Gradle: Include these lines in your build.gradle file:
implementation ‘org.apache.poi:poi:5.0.0’ implementation ‘org.apache.poi:poi-ooxml:5.0.0’
Basic Example: Writing Data to an Excel File

Here’s how to create a basic Excel file and write some data:
import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.ss.usermodel.Sheet; import org.apache.poi.ss.usermodel.Workbook; import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream; import java.io.IOException;
public class ExcelWriter { public static void main(String[] args) throws IOException { // Create a Workbook Workbook workbook = new XSSFWorkbook();
// Create a Sheet Sheet sheet = workbook.createSheet("Employee Data"); // Create Rows and Cells Row headerRow = sheet.createRow(0); headerRow.createCell(0).setCellValue("Name"); headerRow.createCell(1).setCellValue("ID"); headerRow.createCell(2).setCellValue("Salary"); // Add some sample data Row dataRow = sheet.createRow(1); dataRow.createCell(0).setCellValue("John Doe"); dataRow.createCell(1).setCellValue(1001); dataRow.createCell(2).setCellValue(60000); // Write the output to a file FileOutputStream fileOut = new FileOutputStream("employees.xlsx"); workbook.write(fileOut); fileOut.close(); // Close the workbook workbook.close(); System.out.println("Excel file has been generated successfully."); }
}
📝 Note: Make sure to adjust file paths and names according to your environment setup.
Handling Excel Data

Reading Data from Excel Files

Reading data from an Excel file involves similar principles but in reverse. Here’s a simple example:
import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.ss.usermodel.Sheet; import org.apache.poi.ss.usermodel.Workbook; import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream; import java.io.IOException;
public class ExcelReader { public static void main(String[] args) throws IOException { FileInputStream file = new FileInputStream(“employees.xlsx”); Workbook workbook = new XSSFWorkbook(file);
// Get the first sheet Sheet sheet = workbook.getSheetAt(0); // Iterate through all rows and columns for (Row row : sheet) { for (Cell cell : row) { switch (cell.getCellType()) { case STRING: System.out.print(cell.getStringCellValue() + " "); break; case NUMERIC: System.out.print(cell.getNumericCellValue() + " "); break; default: System.out.print(" - "); } } System.out.println(); } workbook.close(); file.close(); }
}
Advanced Features

- Formatting Cells: You can format cells for appearance (like bold, color, font size).
- Working with Formulas: Apache POI allows you to set and evaluate Excel formulas.
- Merging Cells: Combine adjacent cells to create headers or other design features.
- Images: Insert images into cells or anywhere on the worksheet.
In wrapping up our guide on adding data to Excel with Java, we’ve explored several key aspects:
- Environment Setup: We discussed the necessary tools and libraries like Apache POI for Excel manipulation.
- Writing to Excel: We provided a simple yet functional example to write data into an Excel file.
- Reading from Excel: An overview of how to read data, including iterating through cells.
- Advanced Features: Mention of formatting, formulas, and more advanced Excel functionalities.
By following these steps, Java developers can effectively integrate Excel functionalities into their applications, enhancing data management and reporting capabilities. This approach not only streamlines data operations but also opens up a plethora of analytical possibilities with one of the most widely used office tools.
What are the advantages of using Apache POI over JExcelApi?
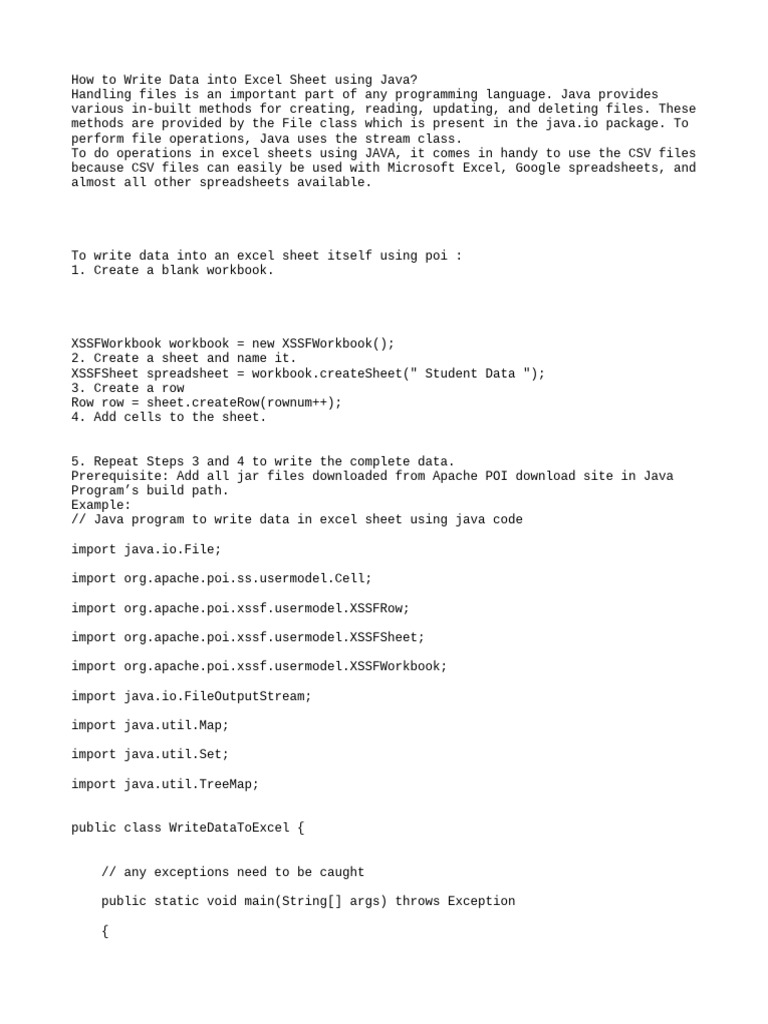
+
Apache POI supports a wider range of Excel features, including all versions of Excel, complex formulas, charts, and pivot tables, making it more versatile for advanced applications. JExcelApi is noted for its simplicity and speed, but it lacks support for newer Excel features.
How can I read data from a specific cell in an Excel file?

+
To read data from a specific cell, you first locate the row and then the cell within that row using methods like getRow(int)
and getCell(int)
.
Can Apache POI handle Excel formulas?

+
Yes, Apache POI can both create and evaluate formulas within Excel sheets. It uses HSSFFormulaEvaluator for .xls
files and XSSFFormulaEvaluator for .xlsx
files to manage formulas.