Effortlessly Add Sheets in Excel with VBA Code

When working with large amounts of data in Microsoft Excel, efficiency is key. Adding sheets manually can be a time-consuming task, especially if you need to insert several new sheets at once. Fortunately, Visual Basic for Applications (VBA) provides a streamlined solution to automate this process, saving you precious time and reducing the risk of human error. In this post, we'll dive into how you can use VBA to add sheets to your Excel workbook effortlessly.
Understanding VBA and Its Benefits

Before we get into the specifics of adding sheets, it's crucial to understand what VBA is and why it's beneficial:
- Automation: VBA allows you to automate repetitive tasks, like adding sheets, which can be tedious when done manually.
- Accuracy: Automating tasks with VBA reduces the likelihood of mistakes that might occur when performing operations by hand.
- Efficiency: VBA can execute complex operations in a fraction of the time it would take to do them manually, boosting your productivity.
- Flexibility: With VBA, you can tailor actions to fit exactly what you need, whether it's adding sheets with specific names or placing them at particular locations in the workbook.
Setting Up Your VBA Environment

To start using VBA in Excel, you'll first need to access the VBA editor:
- Open your Excel workbook.
- Press Alt + F11 to open the Visual Basic Editor.
- In the Project Explorer, locate your workbook and right-click on any of the items (e.g., Workbook or Sheet). Select Insert > Module to add a new module where you'll write your VBA code.
Adding Sheets with VBA Code
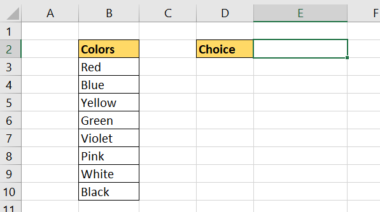
Now that you have your VBA environment set up, let's look at the simplest way to add a new sheet to your workbook:
Sub AddSheet()
Sheets.Add After:=Sheets(Sheets.Count)
End Sub
This code snippet will add a new sheet to your workbook immediately after the last existing sheet.
Adding Multiple Sheets

If you need to add more than one sheet, you can modify the code to suit your needs:
Sub AddMultipleSheets()
Dim i As Integer
For i = 1 To 5 ' Change 5 to the number of sheets you want to add
Sheets.Add After:=Sheets(Sheets.Count)
Next i
End Sub
⚙️ Note: This code will create five new sheets named Sheet1, Sheet2, etc., sequentially after the existing sheets.
Customizing Sheet Names

Often, you’ll want to name your sheets something more meaningful than the default names. Here’s how you can specify a name:
Sub AddNamedSheet()
Dim ws As Worksheet
Set ws = Sheets.Add(After:=Sheets(Sheets.Count))
ws.Name = "New Sheet"
End Sub
⚙️ Note: If the name you specify already exists, VBA will automatically modify it to avoid conflicts by appending a number, e.g., "New Sheet (2)".
Handling Errors and Edge Cases

When programming with VBA, it’s important to consider potential errors:
- Name Conflict: If a sheet with the same name exists, Excel will rename it. You might want to control this behavior.
- Workbook Protection: VBA will not be able to add sheets if the workbook or specific sheets are protected.
- Max Sheets Limit: Excel has a limit of 255 worksheets per workbook. VBA will error out if this limit is reached.
Sub AddSheetWithErrorHandler()
On Error GoTo ErrorHandler
Dim ws As Worksheet
Set ws = Sheets.Add(After:=Sheets(Sheets.Count))
ws.Name = "New Sheet"
Exit Sub
ErrorHandler:
MsgBox "Error " & Err.Number & ": " & Err.Description, vbCritical, "Error"
End Sub
Advanced VBA Techniques for Sheet Management

Here are some advanced VBA operations you might find useful:
- Sheet Location: Place the new sheet at a specific position:
Sub AddSheetAtIndex()
Dim ws As Worksheet
Set ws = Sheets.Add(Before:=Sheets(1)) ‘ Add before the first sheet
ws.Name = “Sheet at Position 1”
End Sub
Sub CopySheet()
Dim SourceSheet As Worksheet
Dim TargetSheet As Worksheet
Set SourceSheet = Sheets(“Sheet1”)
SourceSheet.Copy After:=Sheets(Sheets.Count)
End Sub
Recap

In this exploration of VBA to add sheets in Excel, we’ve covered:
- What VBA is and why it’s beneficial for automation.
- How to set up the VBA environment in Excel.
- Basic to advanced VBA code for adding, naming, and managing sheets.
- Handling potential errors during the process.
By incorporating VBA into your Excel workflow, you'll unlock a new level of efficiency in handling your data, making the task of adding sheets as seamless as clicking a button. Whether you're creating reports, tracking inventory, or managing large datasets, these VBA techniques will ensure your time is spent on analysis rather than administrative tasks. Remember, the key to mastering VBA is practice and experimentation, so don't hesitate to tweak and customize these scripts to fit your unique workflow.
How do I run VBA code in Excel?

+
To run VBA code, you can press Alt + F8, select the macro you want to run from the list, and then click “Run”. Alternatively, you can create a button or an icon on your Excel sheet linked to the macro.
What are the limitations of using VBA to add sheets?

+
VBA can’t overcome Excel’s built-in limits, like the maximum number of sheets (255), or issues related to workbook protection. Also, if sheets have complex data or formatting, copying might not preserve all aspects of the original sheet.
Can VBA automate more than just adding sheets?

+
Absolutely! VBA can be used to automate various tasks in Excel including data manipulation, formula application, formatting, and even creating interactive forms or dashboards. The possibilities are vast and only limited by your programming skills.
Is it possible to run VBA without opening the VBA editor?

+
Yes, you can run VBA macros from buttons or custom ribbon tabs within Excel, making the execution of VBA code a seamless part of your regular Excel workflow.
What security considerations should I keep in mind with VBA?

+
VBA macros can contain malicious code. It’s advisable to enable macro security settings, especially in corporate environments, to only run macros from trusted sources. Always be cautious when opening files from unknown origins.