Effortlessly Add Excel Sheets with Pandas: A Quick Guide

Understanding how to manipulate and integrate Excel sheets into your data processing pipeline can significantly streamline your workflow, especially when dealing with complex datasets. With Python's Pandas library, you can effortlessly add or merge Excel sheets, analyze data, and automate tasks. This comprehensive guide will walk you through the process, from basic to advanced techniques, ensuring you can leverage the full potential of Excel within your Python environment.
Getting Started with Pandas

Before diving into the specifics of handling Excel sheets with Pandas, it's essential to ensure you have Python installed along with the Pandas library. Here's how to set it up:
- Install Python: If you don't already have Python, you can download it from the official Python website.
- Install Pandas: Run the command
pip install pandas
orconda install pandas
if you use Anaconda.
Reading Excel Sheets with Pandas

Pandas offers robust functionalities to read Excel files. Here's how to get started:
import pandas as pd
# Read an Excel file
df = pd.read_excel('your_excel_file.xlsx', sheet_name='Sheet1')
print(df)
💡 Note: Ensure the Excel file path is correct and the file is in a readable format. You might need to install openpyxl with `pip install openpyxl` to read Excel files.
Merging Multiple Excel Sheets

When dealing with multiple sheets, merging them into a single DataFrame is often necessary. Here's how you can do it:
def merge_excel_sheets(file_path):
xls = pd.ExcelFile(file_path)
sheet_list = xls.sheet_names
df_list = [pd.read_excel(xls, sheet_name=sheet) for sheet in sheet_list]
merged_df = pd.concat(df_list, ignore_index=True)
return merged_df
merged_data = merge_excel_sheets('your_excel_file.xlsx')
print(merged_data)
Advanced Data Manipulation with Excel

Excel often contains complex data structures that require advanced manipulation. Here are some techniques:
- Concatenating: Join multiple DataFrames vertically or horizontally.
- Merging: Combine sheets based on keys or indices using merge or join functions.
- Pivoting: Turn data from row-level to columnar structure or vice versa.
# Example of pivoting
pivot_table = df.pivot(index='Date', columns='Category', values='Value').fillna(0)
Exporting Data Back to Excel

Once you've processed your data, exporting it back into Excel is straightforward:
merged_data.to_excel('processed_data.xlsx', index=False)
Working with Multiple Excel Files

If you need to combine data from multiple Excel files, here's a scalable approach:
from glob import glob
def combine_excel_files(pattern):
files = glob(pattern)
df_list = []
for file in files:
xls = pd.ExcelFile(file)
for sheet_name in xls.sheet_names:
df_list.append(pd.read_excel(xls, sheet_name=sheet_name))
combined_df = pd.concat(df_list, ignore_index=True)
return combined_df
combined_data = combine_excel_files('*.xlsx') # Adjust pattern to match your files
🔍 Note: Use the glob pattern wisely to avoid unintended file inclusions.
Handling Large Excel Files

Working with large datasets can be memory-intensive. Pandas offers techniques to handle these situations:
- Chunking: Process the Excel file in smaller parts:
for df in pd.read_excel('large_file.xlsx', chunksize=1000):
# Process each chunk
print(df.head())
In this guide, we've explored various ways to work with Excel sheets using Pandas, from basic file operations to advanced data manipulation techniques. The ability to integrate Excel with Python's powerful data processing capabilities opens up a world of possibilities for data analysts, scientists, and developers alike. Remember, the key to mastering data manipulation lies in understanding the tools at your disposal and knowing when to apply them for maximum efficiency.
What if my Excel file has multiple sheets? How do I access them?

+
You can specify the sheet you want to read by using the sheet_name
parameter in pd.read_excel()
. For example, pd.read_excel('file.xlsx', sheet_name='Sheet2')
will load ‘Sheet2’.
Can I read Excel files without headers?
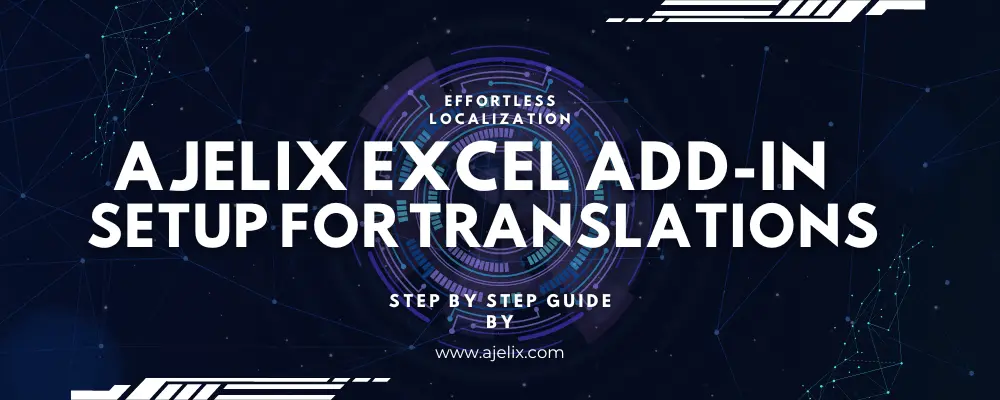
+
Yes, you can set the header=None
parameter to treat the first row as data:
df = pd.read_excel(‘file.xlsx’, header=None)
How can I avoid loading the entire Excel file if it’s very large?

+
Use the chunksize
parameter in pd.read_excel()
to read the file in smaller parts:
for df in pd.read_excel(‘large_file.xlsx’, chunksize=1000):
# Process each chunk here