3 Easy Ways to Unhide Sheets in Excel Macros
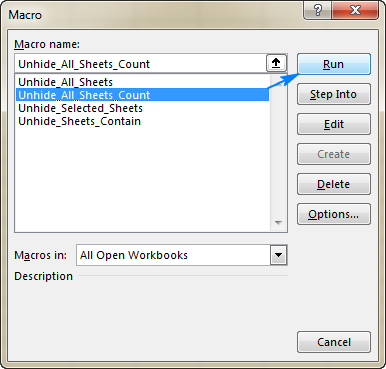
Excel macros are powerful tools for automating repetitive tasks, and hiding or unhiding sheets is one common functionality that can save users significant time. Whether you're dealing with sensitive data or simply organizing your workbook, knowing how to manage hidden sheets efficiently is key. Here are three easy ways to unhide sheets in Excel using VBA (Visual Basic for Applications) macros:
Method 1: Unhide All Sheets

If your goal is to reveal all hidden sheets in your workbook, this straightforward VBA macro will do the trick:
💡 Note: This method will unhide all sheets, including Very Hidden ones, which are not usually visible via the Excel UI.
Sub UnhideAllSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Visible = xlSheetVisible
Next ws
End Sub
Method 2: Unhide Specific Sheets by Name

Need to unhide only certain sheets? Here's how to target sheets by their exact names:
- Change "SheetName" to the name of the sheet(s) you want to unhide:
Sub UnhideSpecificSheet()
' Unhide "SheetName"
ThisWorkbook.Worksheets("SheetName").Visible = xlSheetVisible
End Sub
To unhide multiple sheets, you can list them as follows:
Sub UnhideMultipleSheets()
' List of sheet names to unhide
Dim sheetNames As Variant
sheetNames = Array("SheetName1", "SheetName2", "SheetName3")
' Loop through the array and unhide each sheet
Dim sheet As Variant
For Each sheet In sheetNames
If WorksheetExists(sheet) Then
ThisWorkbook.Worksheets(sheet).Visible = xlSheetVisible
End If
Next sheet
End Sub
Support Function

To ensure the sheet exists before trying to unhide it, include this helper function:
Function WorksheetExists(sheetName As String) As Boolean
Dim ws As Worksheet
On Error Resume Next
Set ws = ThisWorkbook.Worksheets(sheetName)
WorksheetExists = (Err.Number = 0)
Err.Clear
End Function
Method 3: Custom Unhide Sheet Dialogue

Providing users with a custom interface to unhide sheets can greatly enhance usability. Here's a macro to create a user form for selecting sheets to unhide:
💡 Note: This method requires you to insert a UserForm in the VBA Editor first, then name the listbox "lstHiddenSheets" and the command buttons "cmdUnhide" and "cmdCancel" respectively.
Sub UnhideSheetsDialog()
UserForm1.Show
End Sub
And here's the UserForm's code-behind:
Private Sub UserForm_Initialize()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If ws.Visible = xlSheetHidden Or ws.Visible = xlSheetVeryHidden Then
Me.lstHiddenSheets.AddItem ws.Name
End If
Next ws
End Sub
Private Sub cmdUnhide_Click()
Dim i As Integer
For i = 0 To Me.lstHiddenSheets.ListCount - 1
If Me.lstHiddenSheets.Selected(i) Then
ThisWorkbook.Worksheets(Me.lstHiddenSheets.List(i)).Visible = xlSheetVisible
End If
Next i
Me.Hide
End Sub
Private Sub cmdCancel_Click()
Me.Hide
End Sub
Incorporating these methods into your Excel toolkit can streamline your workflow and improve your productivity with hidden sheets. Whether you're unearthing all hidden sheets or selectively revealing only specific ones, VBA macros offer a robust solution.
At the end of the day, the choice of method depends on your specific needs:
- Unhiding all sheets is great for debugging or quickly accessing all data.
- Targeting specific sheets helps with controlling visibility in a controlled manner.
- A user interface for unhiding sheets makes the process user-friendly, especially in workbooks shared with others.
Can I unhide sheets in Excel without using VBA?
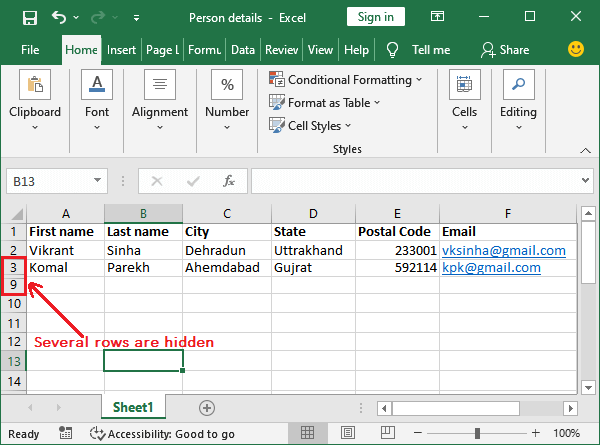
+
Yes, you can unhide a single sheet in Excel without VBA by right-clicking any visible sheet’s tab, selecting “Unhide”, and then choosing the sheet from the list provided. However, VBA is necessary for unhiding multiple sheets or Very Hidden sheets.
Is there a way to make a hidden sheet visible again using Excel’s standard interface?

+
Yes, if a sheet is hidden, you can make it visible by right-clicking on any tab, selecting “Unhide,” and then choosing the sheet from the list. However, this method does not apply to sheets set to “Very Hidden” as they don’t appear in this list.
What’s the difference between a “hidden” and “very hidden” sheet?

+
A “hidden” sheet can be unhidden via the Excel interface or VBA, whereas a “very hidden” sheet can only be made visible through VBA. This extra level of control is useful for protecting sensitive data.