5 Tips for Navigating Excel with Python Pandas
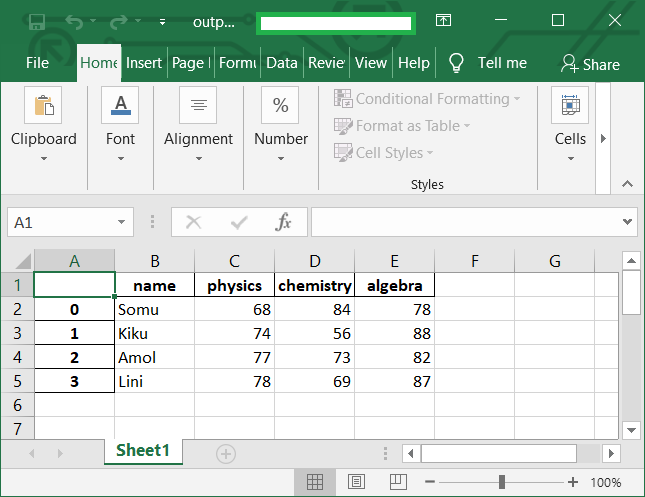
Are you looking to supercharge your data manipulation skills? Combining Excel with Python Pandas might just be the enhancement your toolkit needs. Pandas, Python's data manipulation library, offers dynamic ways to interact with Excel files, transforming your workflow into something more efficient and powerful. Let's delve into some essential tips on how to navigate Excel spreadsheets using Pandas.
Understanding Pandas Excel Integration


Pandas provides a robust set of tools to handle Excel files, offering functionalities to read, write, and manipulate spreadsheets directly from within Python. Here's how you can get started:
- Ensure you have the necessary library installed with:
pip install pandas openpyxl
- Import the libraries:
import pandas as pd
import openpyxl
Reading Excel Files

Reading Excel files is a common first step in data analysis. Here’s how you can do it:
data = pd.read_excel(‘yourfile.xlsx’, sheet_name=‘Sheet1’)
🚨 Note: The 'sheet_name' parameter can accept integers or strings for sheet selection.
Writing to Excel Files

Once your analysis is complete, you might want to save your DataFrame back into Excel:
data.to_excel(‘newfile.xlsx’, sheet_name=‘Analysis’)
Navigating and Manipulating Data

Pandas offers a multitude of methods to manipulate your data:
- Select Columns:
data['Column Name']
- Filter Data:
data[data['Column'] == 'Some Value']
- Add New Columns:
data['New Column'] = new_values
- Drop Columns:
data = data.drop(columns=['Column to Drop'])
Handling Excel-Specific Features

Excel has its own quirks, like merged cells or special formatting. Here are tips to manage those:
Excel Feature | Pandas Equivalent |
---|---|
Merged Cells | Can be handled by cleaning data or choosing to skip reading problematic areas. |
Formatting | Formatting can be ignored or preserved through openpyxl's styling capabilities. |
Formulas | Pandas doesn't read formulas as formulas; they are converted to values. |

🔍 Note: While Pandas handles most Excel features well, some Excel-specific features might need additional tools or workarounds.
Automating Workflows

Use Pandas to automate repetitive Excel tasks:
- Looping through files:
import os
for file in os.listdir('path/to/excel/files'): if file.endswith(".xlsx"): process_file(file) - Batch Processing: Use this loop to perform operations on each file.
With these tips, you're now equipped to handle Excel data like a pro with the power of Python Pandas. From reading and writing to complex data manipulation, Pandas makes Excel navigation a breeze, enhancing your ability to quickly process and analyze data.
Summing it Up

Incorporating Python Pandas with Excel not only makes data analysis faster but also more systematic and less prone to errors. You can leverage the programming capabilities of Python while still making use of the familiarity and flexibility of Excel. Whether it’s automating routine tasks, performing complex data operations, or handling the intricacies of Excel spreadsheets, Pandas stands out as an invaluable tool for data analysts.
Can Pandas handle multiple sheets in an Excel file?

+
Yes, Pandas can read multiple sheets from an Excel file. You can use the ‘sheet_name’ parameter to specify either a single sheet or a list of sheets to read.
How do I handle Excel dates in Pandas?

+
Excel dates can be converted to Python datetime objects using the ‘parse_dates’ parameter in the ‘read_excel’ function. Alternatively, you can parse date strings manually with Pandas’ date conversion functions.
What if my Excel file contains formulas?

+
When Pandas reads an Excel file, formulas are calculated and the results are read as values, not as formulas. If you need to retain formulas, you might need to use additional libraries like openpyxl to read and write Excel files while preserving the formulas.