Java: Storing Excel Data into Database Easily

Storing data from Excel into a database using Java is a common task for developers working on enterprise applications or data-centric projects. The process can streamline data handling, enabling seamless integration with backend systems and simplifying data analysis. Here's a comprehensive guide on how you can easily manage this task using Java with popular libraries like Apache POI for Excel manipulation and JDBC for database interaction.
Why Store Excel Data in a Database?

Before diving into the technical aspects, understanding the benefits of moving Excel data into a database is crucial:
- Automation: Automating the data transfer eliminates manual entry errors and reduces labor time.
- Data Integrity: Databases enforce data types and constraints, ensuring data consistency and integrity.
- Scalability: Handling large datasets in Excel can become unwieldy, whereas databases are designed for scalability.
- Multi-user Access: Databases provide secure, simultaneous access for multiple users.
- Data Analysis: SQL and other database query languages offer powerful tools for data analysis.
Setting Up Your Environment

To begin, you'll need to set up your development environment with the necessary tools:
1. JDK (Java Development Kit)

Ensure you have Java installed on your system, as this is essential for running Java applications.
2. IDE (Integrated Development Environment)

Choose an IDE like Eclipse, IntelliJ IDEA, or NetBeans to write, debug, and run your Java code.
3. Libraries

You’ll need:
- Apache POI for reading and writing Excel files (
poi-ooxml
andpoi-ooxml-schemas
). - JDBC driver for your specific database (e.g., MySQL JDBC Driver).
Here's how you can add these dependencies to your project using Maven:
org.apache.poi
poi-ooxml
5.2.3
org.apache.poi
poi-ooxml-schemas
4.1.2
mysql
mysql-connector-java
8.0.28
📝 Note: The version numbers used here are illustrative. Always check for the latest versions of these libraries.
Reading Excel Data with Apache POI

Here's how you can use Apache POI to read data from an Excel file:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelReader {
public static void main(String[] args) {
String filePath = "path/to/your/excel/file.xlsx";
try (FileInputStream fis = new FileInputStream(filePath);
Workbook workbook = new XSSFWorkbook(fis)) {
Sheet sheet = workbook.getSheetAt(0); // First sheet
// Iterate through rows and columns to fetch data
for (Row row : sheet) {
for (Cell cell : row) {
// Handle different cell types
switch (cell.getCellType()) {
case STRING: System.out.print(cell.getStringCellValue() + "\t"); break;
case NUMERIC: System.out.print(cell.getNumericCellValue() + "\t"); break;
case BOOLEAN: System.out.print(cell.getBooleanCellValue() + "\t"); break;
default: break;
}
}
System.out.println();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Inserting Excel Data into a Database

Once the data is extracted, you can insert it into your database. Here's how you might approach this using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class DatabaseInserter {
public static void insertData(String username, String password, String url, String tableName, DataRows rows) {
String sql = "INSERT INTO " + tableName + " (column1, column2, column3) VALUES (?, ?, ?)";
try (Connection conn = DriverManager.getConnection(url, username, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
for (DataRow row : rows.getRows()) {
pstmt.setString(1, row.getColumn1());
pstmt.setString(2, row.getColumn2());
pstmt.setString(3, row.getColumn3());
pstmt.addBatch();
}
pstmt.executeBatch();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
// Assuming 'rows' is an object containing all your Excel data
DataRows rows = ExcelReader.getRows();
insertData("user", "pass", "jdbc:mysql://localhost:3306/dbname", "MyTable", rows);
}
}
// Example class to hold row data, not part of POI or JDBC
class DataRows {
private List<DataRow> rows;
// Getters and setters
}
class DataRow {
private String column1;
private String column2;
private String column3;
// Getters and setters
}
🔍 Note: Replace 'MyTable', 'jdbc:mysql://localhost:3306/dbname', 'user', and 'pass' with your actual database details.
Handling Large Excel Files
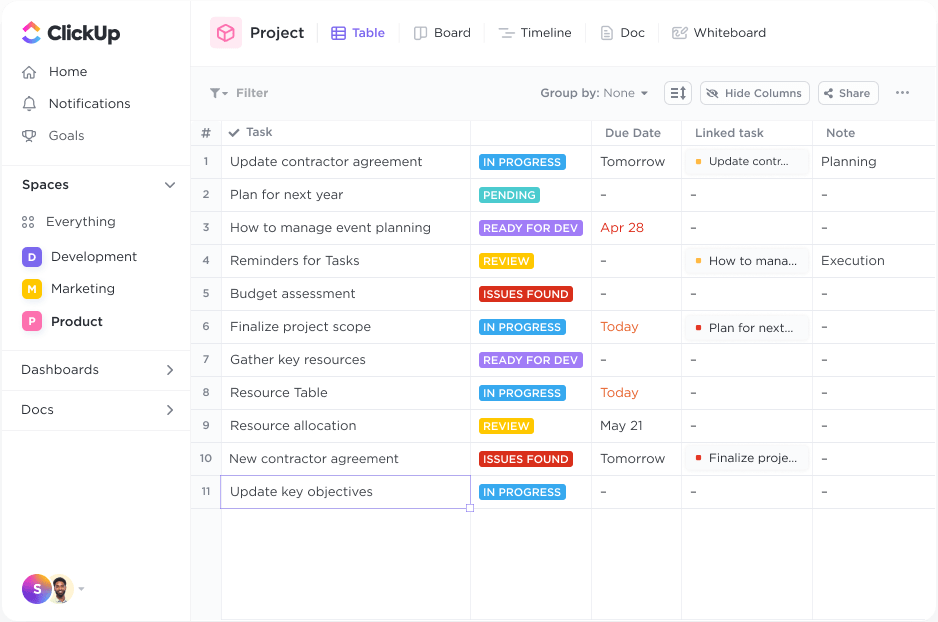
When dealing with large Excel files:
- Use Event API in Apache POI to avoid loading the entire file into memory.
- Consider batch inserting data into the database to manage memory better.
Summing Up

By following the steps outlined above, you can effectively store data from Excel files into databases using Java. This process not only automates data handling but also ensures data integrity, scalability, and multi-user access. Remember to use Apache POI for reading Excel files and JDBC for database interactions. Handling large files might require additional considerations like event-based reading and batch inserts.
What version of Java should I use for Excel database integration?

+
Java 8 or higher is recommended as it supports lambda expressions which can simplify working with POI and JDBC.
Can I use this method to store data from other file formats like CSV?

+
Yes, although the method described uses Excel files, you can easily adapt this to read CSV or other delimited files using appropriate libraries like OpenCSV for CSV files.
What if my database structure does not match the Excel sheet’s structure?

+
You would need to transform the data either in Excel before loading or in Java during the data extraction. This might involve mapping columns or using logic to insert data into different tables or join tables.