Effortlessly Set Excel Sheet Names with Apache POI

To manage and interact with Excel documents in Java, Apache POI provides an extensive library that lets you achieve a wide array of spreadsheet operations. Here, we'll delve into how you can change or set the sheet names within an Excel workbook, which can be particularly useful for organizing data or improving document readability.
Understanding Apache POI

Apache POI is a library for working with Microsoft Office formats. It’s open-source and widely used due to its robust functionality and ease of use. The POI stands for “Poor Obfuscation Implementation” humorously, but it’s also said to mean “Picture Oriented Investigation” when related to its graphics capabilities. Key components include:
- POIFS - OLE2 Filesystem
- HSSF - BIFF8 File Format for Excel ‘97-2007
- XSSF - OOXML File Format for Excel 2007 and later (.xlsx)
- HWPF - Microsoft Word File Formats
- HSLF - Microsoft PowerPoint File Formats
- DDF - Drawing Format
Setting Up Your Environment

Before diving into setting sheet names, you need to set up your development environment:
- Ensure you have Java installed.
- Add Apache POI to your project’s classpath. You can do this manually or use build tools like Maven or Gradle.
Importing Necessary Classes

Begin by importing the required classes from Apache POI:
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
Creating or Loading a Workbook

You can either create a new workbook or load an existing one. Here’s how to load an Excel workbook:
FileInputStream excelFile = new FileInputStream(new File(“path/to/your/file.xlsx”));
Workbook workbook = new XSSFWorkbook(excelFile);
Changing Sheet Names

To change or set the name of a sheet, you’ll use the Workbook
and Sheet
classes. Here are the steps:
Accessing Sheets

First, access the sheet whose name you wish to change:
Sheet sheet = workbook.getSheetAt(0); // Selects the first sheet
Renaming a Sheet

Now, rename the sheet:
sheet.getSheetName();
workbook.setSheetName(0, “New Sheet Name”);
💡 Note: Sheet names must adhere to Excel's naming conventions, like not exceeding 31 characters, no special characters except for underscore, space, or period, and cannot be blank.
Creating a New Sheet

If you need to create a new sheet rather than just renaming an existing one:
Sheet newSheet = workbook.createSheet(“My New Sheet”);
After making changes, you must save your workbook back to the file:
FileOutputStream fileOut = new FileOutputStream("path/to/your/file.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
Best Practices When Managing Sheet Names

- Ensure Uniqueness: Sheet names should be unique within the workbook.
- Character Limits: Adhere to Excel's sheet name limits.
- Avoid Special Characters: Use characters that are safe and readable in a file system.
The significance of correctly setting or changing Excel sheet names can't be overstated. It helps in organizing data, makes navigation within a workbook more intuitive, and improves the overall user experience. Understanding how to leverage Apache POI for this task not only enhances the capabilities of your Java applications but also aligns with modern data management practices. Remember that while manipulating Excel files programmatically, care must be taken to handle errors gracefully, use descriptive variable names, and maintain clean code for better maintainability and readability.
Can I use Apache POI to change sheet names dynamically?

+
Yes, you can change sheet names dynamically by using variables or user input to set the names. This allows for automated or user-driven changes to your Excel workbook.
Is it possible to recover the original sheet names if I rename them?
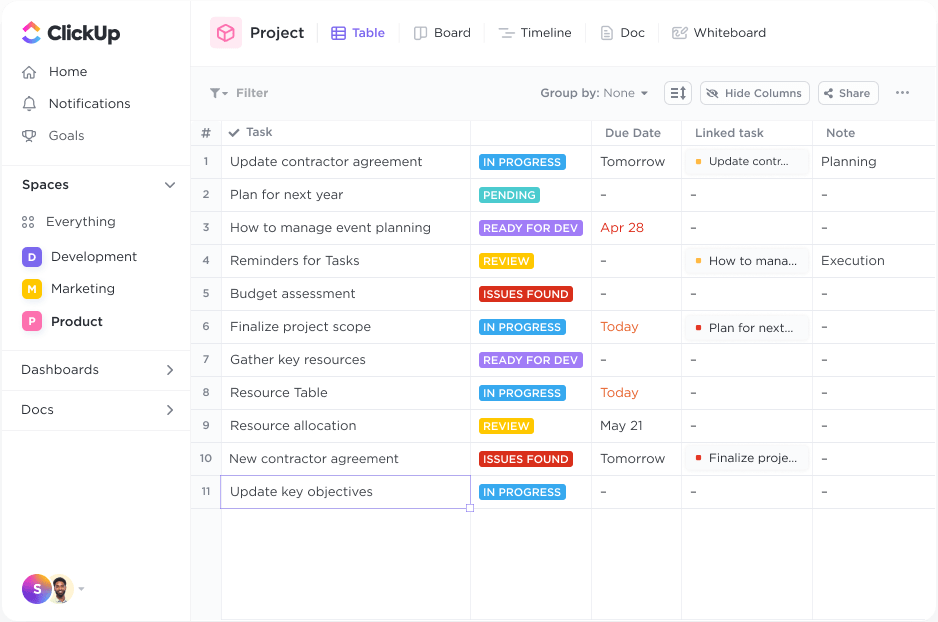
+
If you’ve stored the original sheet names elsewhere in your program or have access to a previous version of the workbook, you can revert to the original names. Apache POI does not keep a history of changes.
How many sheets can I have in an Excel workbook?

+
Excel supports up to 255 sheets in one workbook, although the practical limit might be lower due to system memory constraints.