5 Simple Tips for Naming Excel Sheets in Java

In the world of data management, organizing and manipulating spreadsheet data efficiently can make a significant difference in productivity, especially when working within Java applications. A crucial aspect of this is naming Excel sheets properly to ensure clarity, traceability, and ease of navigation. Here are five simple yet powerful tips for naming Excel sheets in Java, tailored to enhance your workflow and data management:
1. Use Descriptive Names


Naming Excel sheets descriptively is a fundamental step in ensuring that your data management practices are both effective and user-friendly. Here’s how to approach it in Java:
- Be Clear and Concise: Use names that instantly convey the purpose or content of the sheet. For instance, naming a sheet “SalesData_2023” is much clearer than something like “Sheet2”.
- Avoid Spaces and Special Characters: Java’s APIs can struggle with spaces or special characters in sheet names. Instead, use underscores or hyphens to separate words, like “Annual_RevenueAnalysis”.
- Follow Naming Conventions: Adopting a consistent naming strategy can help in maintaining uniformity across your spreadsheets. For example, you might use prefixes to categorize sheets (“FIN” for finance-related sheets).
💡 Note: When using descriptive names, always consider the limitations of Excel sheet names. They can be up to 31 characters long and cannot contain certain characters like slashes or square brackets.
2. Automate Naming with Date and Time

Using Java to automate the naming process can not only save time but also reduce human error. Here are steps to implement this:
- Current Date: Append or include the current date to the sheet name for time-specific reports or logs. Use Java’s
LocalDate
class to format the date. - Time Stamps: For sheets that are updated frequently, adding a timestamp can prevent confusion between versions. The
LocalDateTime
class can be utilized for this.
Date Format | Example |
---|---|
yyyyMMdd | Sales_20231115 |
dd-MM-yyyy | Accounts_15-11-2023 |

⏰ Note: When naming sheets with dates, consider readability; a date like 12-01-2023 might be confusing without context (December 1, 2023 or January 12, 2023?).
3. Utilize Enumerations for Consistency

To maintain consistency in sheet naming across different parts of your application:
- Define Enumerations: Use Java enums to define fixed sets of sheet names, ensuring that names are used consistently throughout the codebase.
- Switch-Case Logic: Implement switch-case statements or lookup mechanisms to map enum values to sheet names.
4. Implement Naming Validation

Proper validation helps in maintaining data integrity:
- Check for Duplicates: Ensure that no two sheets have the same name by checking before renaming or creating new sheets.
- Character Validation: Use regex or custom validation to ensure sheet names only contain allowed characters. Java’s
Pattern
class can be used for this purpose.
5. Use Metadata for Extended Information

While not directly related to naming, adding metadata can enhance the understanding and management of Excel sheets:
- Include Metadata: In your Java code, you can add metadata to the workbook or sheets which provides context or instructions without affecting the sheet names.
By following these five tips for naming Excel sheets in Java, you can significantly improve the organization, accessibility, and maintainability of your data. Proper naming conventions facilitate quicker data retrieval, reduce errors, and streamline collaboration among team members. Remember, effective data management starts with well-organized Excel sheets, and with these strategies, your Java applications will handle spreadsheets more efficiently.
What are the limitations of Excel sheet names?
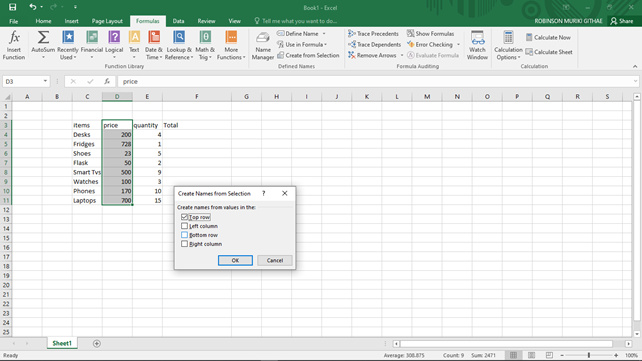
+
Excel sheet names are limited to 31 characters and cannot contain characters like [: \ / ? * [ ]].
How can I automate sheet naming in Java?

+
Java’s SimpleDateFormat
or DateTimeFormatter
classes can be used to format dates and time for automatic sheet naming. Libraries like Apache POI or JXL help manipulate Excel files programmatically.
Why should I use enums for sheet names?

+
Using enums helps maintain consistency across different parts of your application, reducing errors and ensuring that sheet names are fixed and uniform.
What is metadata in the context of Excel sheets?

+
Metadata refers to additional information about the sheet or workbook, like creation date, creator, or purpose, stored either in custom properties or in a separate sheet.
Can I rename sheets dynamically?

+
Yes, you can dynamically rename sheets in Java by using libraries like Apache POI or JXL to access and modify the sheet properties in real-time.