Effortlessly Make Any Sheet Active in Excel with VBA

In the world of Microsoft Excel, efficiency is key. If you frequently work with Excel worksheets, you understand the hassle of manually switching between sheets, especially in workbooks with numerous tabs. This process can be particularly time-consuming and disrupts your workflow. Fortunately, Visual Basic for Applications (VBA) in Excel provides a powerful way to automate and enhance your Excel operations. In this post, we'll explore how you can use VBA to make any sheet active quickly and efficiently, thereby saving time and boosting your productivity.
Understanding VBA and Excel Sheets
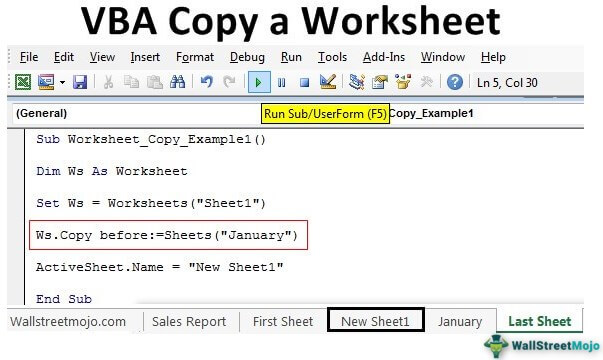
VBA, a programming language developed by Microsoft, is integrated into Excel to automate almost any task you can perform manually. Excel sheets, or worksheets, are the layers within your workbook where data resides. Here’s why mastering VBA to manage these sheets is beneficial:
- Automation: Automate repetitive tasks, like switching to a specific worksheet.
- Efficiency: VBA can execute a set of operations much faster than manual work.
- Customization: Tailor the behavior of Excel to your specific needs.
Preparing Your Excel Environment for VBA
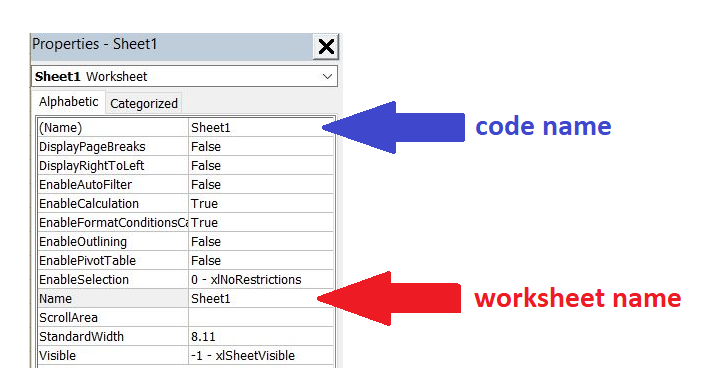
Before diving into coding, ensure your Excel environment is set up for VBA:
- Open Excel.
- Go to Developer tab. If not visible, enable it through File > Options > Customize Ribbon.
- Click on Visual Basic or press Alt+F11 to open the VBA editor.
- In the VBA editor, insert a new module by right-clicking on your workbook’s name in the Project Explorer, then selecting Insert > Module.
Writing VBA Code to Activate Sheets

To programmatically activate any sheet in Excel using VBA, you’ll need to understand the structure of your code:
Sub ActivateSheet()
Sheets(“SheetName”).Activate
End Sub
Here’s a step-by-step guide to writing and executing the code:
- In the VBA editor, ensure you’re in the new module you just created.
- Write the above code, replacing SheetName with the actual name of your sheet.
- To run this subroutine, place the cursor inside it and press F5 or use the "Run" button in the VBA editor.
💡 Note: VBA is case-sensitive when it comes to sheet names, so ensure you type the name exactly as it appears in Excel.
Adding Complexity to Sheet Activation

Excel workbooks often have dynamic content where you might not know the name or position of a sheet beforehand. Here are more sophisticated methods:
Activating Sheets by Index

Sub ActivateSheetByIndex()
Sheets(2).Activate ‘ Activates the second sheet in the workbook
End Sub
This method is particularly useful when dealing with numbered sheets, where the sheet order matters, but not their names.
Activating Sheets with a List or Loop

If you want to activate sheets in a specific order or from a list:
Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Sheets
If ws.Name = “YourSheetName” Then
ws.Activate
Exit For
End If
Next ws
End Sub
This code loops through all sheets in the workbook and activates the one named “YourSheetName”.
👨💻 Note: When looping through sheets, consider using Exit For to exit the loop once the target sheet is activated to save processing time.
Advanced VBA Techniques for Sheet Activation

Using InputBox for Sheet Selection

Provide a user interface for sheet activation:
Sub ActivateSheetInput()
Dim sheetName As String
sheetName = InputBox(“Enter the sheet name to activate:”)
On Error Resume Next
Sheets(sheetName).Activate
If Err.Number <> 0 Then
MsgBox “Sheet not found. Please check the name and try again.”, vbExclamation
End If
On Error GoTo 0
End Sub
Activating the Last Sheet
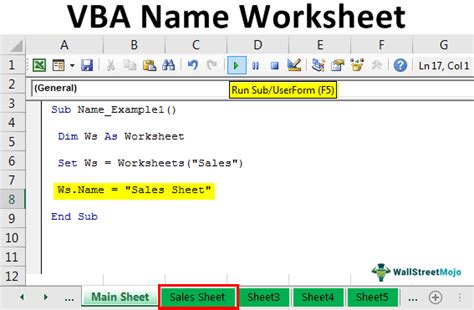
Sub ActivateLastSheet()
Sheets(ThisWorkbook.Sheets.Count).Activate
End Sub
Activating Sheets Based on Conditions

If your sheets follow a pattern, like prefixes or suffixes:
Sub ActivatePatternedSheet()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Sheets
If Left(ws.Name, 2) = “XY” Then
ws.Activate
Exit For
End If
Next ws
End Sub
Handling Errors

VBA provides error handling capabilities to deal with issues like non-existent sheets:
Sub ActivateSheetWithErrorHandling()
On Error GoTo ErrorHandler
Sheets(“SheetName”).Activate
Exit Sub
ErrorHandler:
MsgBox “The specified sheet does not exist.”, vbExclamation
End Sub
Common VBA Code Practices
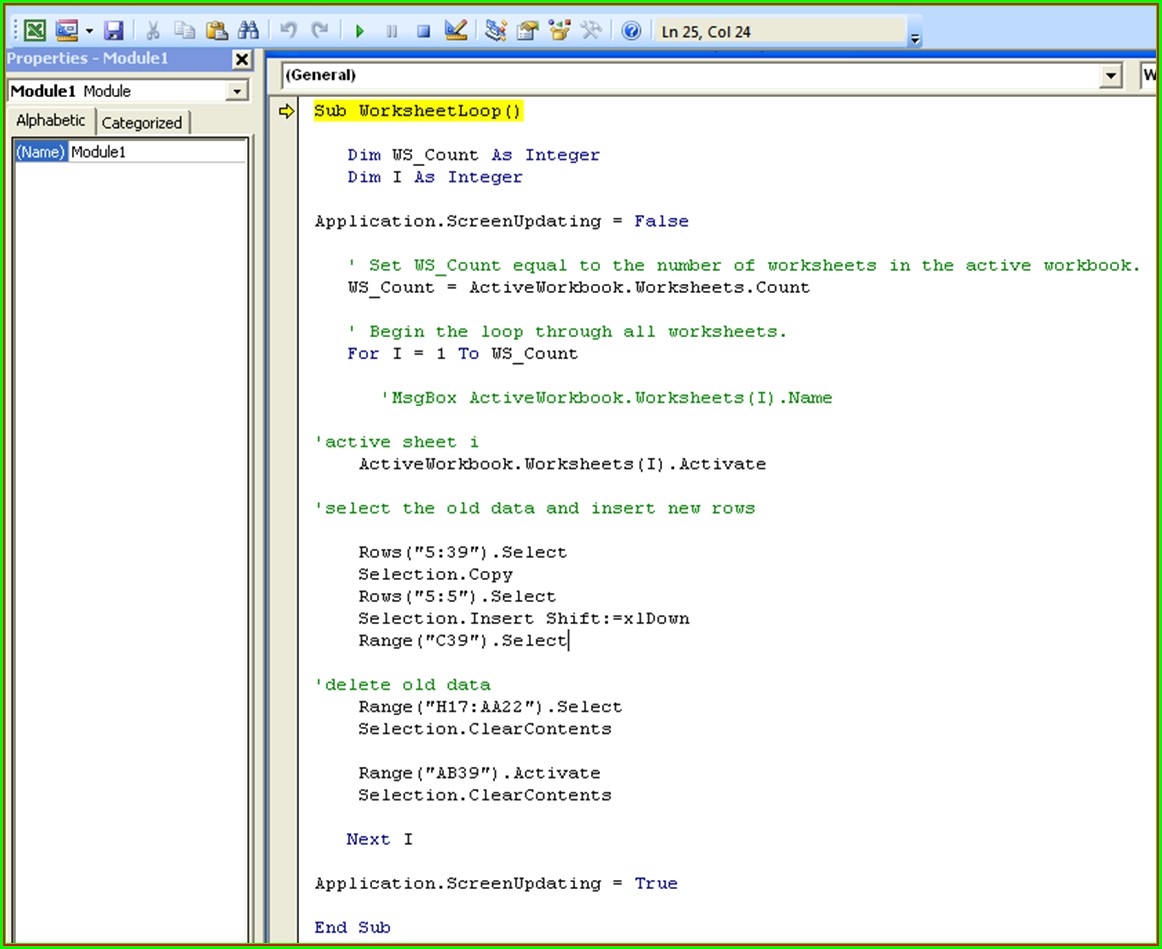
- Document your code: Add comments explaining what each section does.
- Structure with Subs and Functions: Use ‘Sub’ for procedures and ‘Function’ for returning values.
- Naming conventions: Use clear and consistent naming for variables and procedures.
- Error Handling: Implement error handling to manage exceptions gracefully.
💡 Note: Clean code practices can significantly improve the readability and maintainability of your VBA scripts.
The journey through Excel VBA to automate sheet activation is a testament to how you can harness technology to make your workday more efficient. Not only does VBA allow you to save time, but it also lets you customize Excel's behavior to your unique workflow needs. By mastering a few lines of VBA code, you gain the power to switch between sheets seamlessly, handle sheets dynamically, and even incorporate user interaction for more intuitive operations. Remember, while this post provides an overview, Excel VBA is a vast subject with numerous applications beyond sheet activation, ready to be explored and utilized for even greater productivity gains.
How do I enable the Developer tab in Excel?
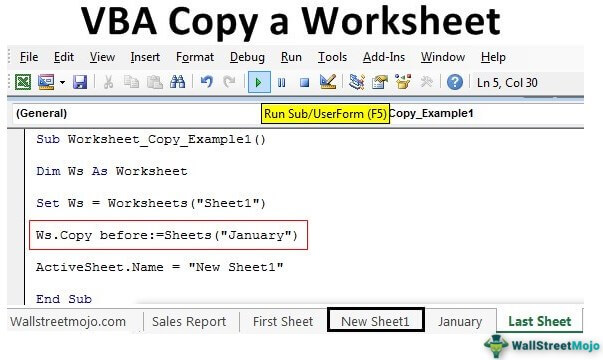
+
Go to File > Options > Customize Ribbon, check the Developer checkbox, and click OK.
Can I use VBA to rename sheets?

+
Yes, use Sheets("OldName").Name = "NewName"
to rename a sheet via VBA.
What if the sheet name I want to activate doesn’t exist?
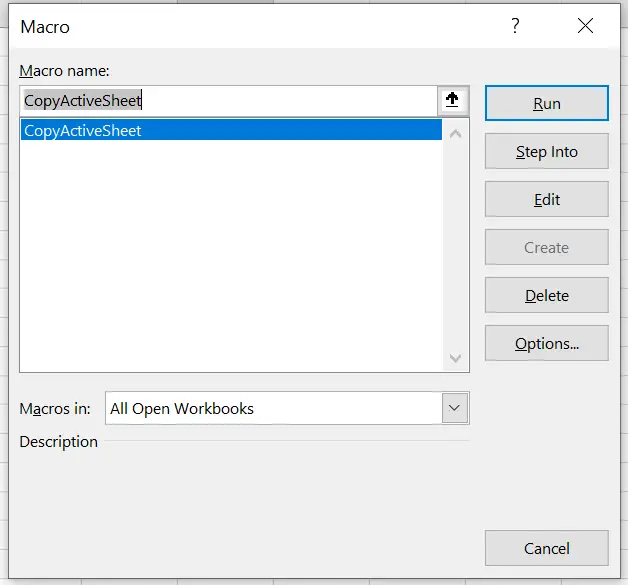
+
You should handle this with error checking, like using On Error Resume Next
to skip the error or showing a message to the user indicating the sheet was not found.