Rename Excel Sheets in C: Quick Guide

Why Rename Excel Sheets in C#?

In the world of data manipulation and spreadsheet management, Excel sheets are a cornerstone for many business processes. Whether you’re dealing with data reports, financial models, or just organizing your weekly to-do list, Excel’s power is undeniable. But what happens when you need to dynamically manage these sheets using C#? Renaming Excel sheets programmatically can offer significant automation benefits:
- Efficiency: Automate repetitive tasks, saving time and reducing errors.
- Scalability: Handle operations on multiple sheets without manual intervention.
- Integration: Seamlessly integrate Excel sheets with C# applications.
Getting Started with Excel and C#

To start working with Excel sheets in C#, you need the Microsoft.Office.Interop.Excel assembly, which provides access to Excel’s COM objects. Here’s how to set up your environment:
- Open your Visual Studio project.
- Add references to Microsoft.Office.Interop.Excel. This can be done by:
- Right-clicking your project in Solution Explorer.
- Selecting ‘Add’ -> ‘Reference’.
- In the ‘COM’ tab, find and select ‘Microsoft Excel Object Library’.
- Make sure you’ve got Microsoft Excel installed on the machine where your code will run.
Creating a New Excel Workbook

Before you can rename a sheet, let’s create a new Excel workbook:
using Microsoft.Office.Interop.Excel;
//…
Application xlApp = new Application();
Workbook workbook = xlApp.Workbooks.Add();
This snippet initializes an Excel application and adds a new workbook.
Renaming a Sheet in C#

Once you have a workbook, renaming a sheet is straightforward:
// Get the active sheet or first sheet if none are active
Worksheet sheet = (Worksheet)workbook.Sheets[1];
sheet.Name = “NewSheetName”;
📝 Note: Be aware that Excel has limitations on sheet names. They must not exceed 31 characters and can't contain certain characters like '/', '\', '[', ']', '*', ':', '?', '!'. Also, remember that each name must be unique within the workbook.
Renaming Multiple Sheets

Let’s say you need to rename several sheets at once. Here’s how you could do it:
foreach (Worksheet sheet in workbook.Sheets)
{
if (sheet.Name.Contains(“OldSheetName”))
{
string newName = “NewPrefix” + sheet.Name.Replace(“OldSheetName”, “”);
sheet.Name = newName;
}
}
Using Tables for Sheet Management

If your Excel work involves complex operations or you’re working with a lot of sheets, using a table to track their names can be helpful:
Sheet Number | Current Name | New Name |
---|---|---|
1 | Sheet1 | DataSheet |
2 | Sheet2 | Summary |
3 | Sheet3 | Charts |

Here's how to rename these sheets based on the table:
var nameMappings = new Dictionary();
nameMappings.Add(1, "DataSheet");
nameMappings.Add(2, "Summary");
nameMappings.Add(3, "Charts");
foreach (KeyValuePair entry in nameMappings)
{
((Worksheet)workbook.Sheets[entry.Key]).Name = entry.Value;
}
Final Thoughts
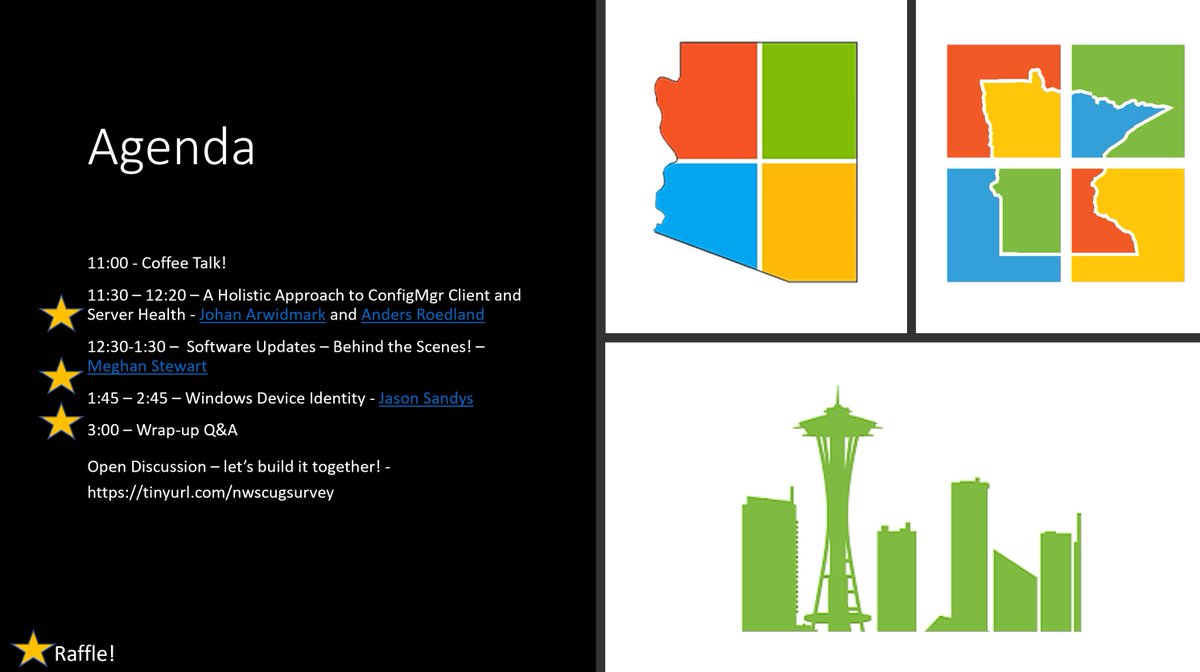
Automating Excel sheet renaming with C# not only enhances productivity but also allows for dynamic handling of data-driven tasks. By integrating Excel with C# applications, you can leverage the power of both tools, making data manipulation more seamless and efficient. Remember to handle errors appropriately, especially with file operations, and ensure your code respects Excel's naming rules to avoid runtime errors.
Can I rename Excel sheets in C# without Microsoft Office installed?
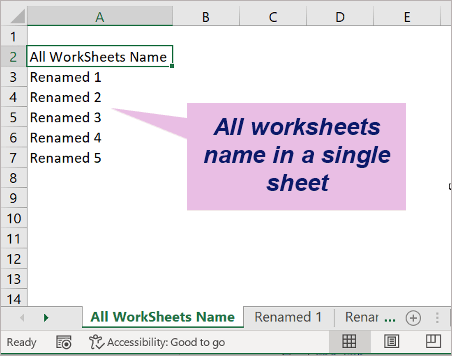
+
Yes, by using third-party libraries like ExcelDataReader or EPPlus, you can manipulate Excel files without needing Microsoft Office.
How do I ensure that the new sheet name is unique within the workbook?

+
Before renaming, iterate through all sheet names to check for uniqueness or append a number if the name already exists.
What happens if I rename a sheet to a name that already exists?

+
Excel will throw an error because sheet names must be unique. You would need to catch this exception and handle it accordingly.