How To Rename Sheet In Excel Using C

Welcome to our deep dive into Excel manipulation using C#. If you're curious about automating your Excel tasks, you're in the right place. Today, we'll walk through the steps to rename sheets in Excel programmatically using C# with the help of the Excel Interop library.
Prerequisites

Before we jump into coding, here’s what you’ll need:
- Visual Studio (or your preferred IDE)
- .NET Framework installed
- Excel installed on your system (for runtime execution of Excel)
Setting Up Excel Interop

To begin, you need to set up the Excel Interop library:
- Open Visual Studio and create a new C# Console Application project.
- Right-click on “References” in the Solution Explorer and select “Add Reference.”
- In the Reference Manager, search for “Microsoft.Office.Interop.Excel” and add it to your project.
💡 Note: This library allows C# to interact with Excel.
Code to Rename Sheet in Excel

Now let’s move to the fun part - writing the code:
using System;
using Excel = Microsoft.Office.Interop.Excel;
namespace ExcelRenameSheet
{
class Program
{
static void Main(string[] args)
{
// Create Excel application object
Excel.Application excelApp = new Excel.Application();
// Open workbook
Excel.Workbook workbook = excelApp.Workbooks.Open(@"C:\Path\To\Your\Workbook.xlsx");
try
{
// Get the Sheets collection
Excel.Sheets sheets = workbook.Sheets;
// Change the name of the first sheet
sheets[1].Name = "NewSheetName";
// Save and close the workbook
workbook.Save();
workbook.Close();
}
catch (Exception ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
finally
{
// Quit Excel and release COM objects
excelApp.Quit();
System.Runtime.InteropServices.Marshal.ReleaseComObject(sheets);
System.Runtime.InteropServices.Marshal.ReleaseComObject(workbook);
System.Runtime.InteropServices.Marshal.ReleaseComObject(excelApp);
}
}
}
}
This code:
- Creates a new instance of the Excel application.
- Opens a specified workbook.
- Renames the first sheet to "NewSheetName."
- Saves and closes the workbook.
- Properly releases COM objects to avoid memory leaks.
Understanding COM Interop and Memory Management

It's crucial to understand how C# interacts with Excel through COM Interop:
- Each Excel object is a COM object and must be properly managed to prevent memory leaks.
- Using
System.Runtime.InteropServices.Marshal.ReleaseComObject
is a standard practice to release these objects explicitly.
Error Handling and Edge Cases
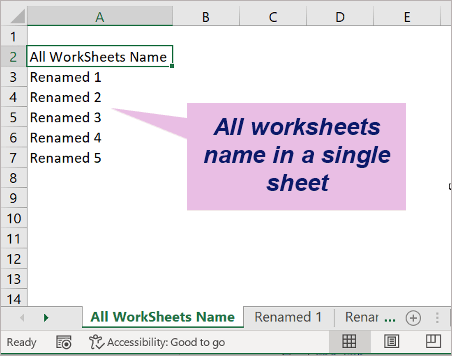
When dealing with Excel, consider:
- Name Length Limit: Sheet names are limited to 31 characters in Excel.
- Duplicate Names: Attempting to set a name already in use will throw an error.
- Sheet Index: Be cautious with sheet indices; ensure the sheet you're renaming exists.
To handle these issues, consider expanding your code:
try
{
if (sheets[1].Name.Length > 31)
throw new Exception("Sheet name exceeds the 31 character limit");
if (IsSheetNameExists(sheets, "NewSheetName"))
throw new Exception("Sheet name already exists");
sheets[1].Name = "NewSheetName";
}
Summary

By now, you should have a solid understanding of how to rename Excel sheets using C#. This approach not only automates mundane tasks but also opens up numerous possibilities for Excel automation. Remember:
- Ensure proper management of Excel COM objects to avoid memory issues.
- Be mindful of Excel’s limitations like sheet name length and uniqueness.
- Use error handling to manage unexpected scenarios gracefully.
Why do we need to release COM objects in C#?

+
COM objects can lead to memory leaks if not released, especially since Excel Interop manages COM objects from another process.
What happens if I try to rename a non-existent sheet?

+
If you attempt to rename a sheet that does not exist, an System.Runtime.InteropServices.COMException
will be thrown.
Can I rename multiple sheets at once?

+
Yes, you can loop through the sheets collection and rename each one individually.