Rename Excel Sheets Effortlessly with VBA Code

In the realm of Microsoft Excel, renaming sheets can be a tedious task, especially when dealing with numerous spreadsheets or a frequently updated workbook. While Excel's user interface does offer basic options for renaming sheets, the process can become monotonous and prone to errors if done manually. However, by leveraging Visual Basic for Applications (VBA), you can automate this process, making it faster and more accurate. Let's delve into how you can rename Excel sheets effortlessly with VBA.
Why Use VBA for Renaming Sheets?

- Automation: VBA scripts can automate repetitive tasks, like renaming sheets in bulk.
- Consistency: Ensure uniformity in naming conventions across your workbook.
- Speed: Rename sheets in seconds rather than manually going through each one.
- Accuracy: Minimize human errors by automating the naming process.
Basic VBA Syntax for Sheet Renaming

Before we dive into more complex examples, understanding the basic syntax is crucial. Here’s how you can rename a single sheet:
Sub RenameSheet()
Sheets(“Sheet1”).Name = “NewName”
End Sub
This code snippet renames the sheet named "Sheet1" to "NewName".
Example: Renaming Multiple Sheets
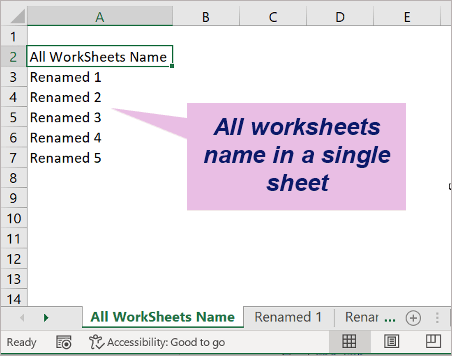
Imagine you have a workbook with many sheets that you want to rename systematically, perhaps by using the date or specific keywords. Here’s how you could achieve this:
Sub RenameMultipleSheets()
Dim ws As Worksheet
Dim i As Integer
i = 1
For Each ws In ThisWorkbook.Worksheets
If i < 10 Then
ws.Name = “Report-0” & i
Else
ws.Name = “Report-” & i
End If
i = i + 1
Next ws
End Sub
✍️ Note: This example assumes your workbook doesn’t have more than 99 sheets to avoid exceeding Excel’s character limit for sheet names.
More Advanced VBA Techniques

Renaming Sheets Based on Cell Content

Sometimes, you might want to name sheets based on the value in a specific cell within that sheet. Here’s how you could set this up:
Sub RenameSheetsFromCell()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
On Error Resume Next
If ws.Cells(1, 1).Value <> “” Then
ws.Name = Left(ws.Cells(1, 1).Value, 31) ‘ Limit to 31 chars
End If
On Error GoTo 0
Next ws
End Sub
🌟 Note: The sheet name is limited to 31 characters by Excel, so the Left
function is used to truncate any longer values.
Dynamic Sheet Naming with Pattern Recognition

You might also need to rename sheets dynamically based on certain patterns or keywords found within the current sheet name. This can be particularly useful when cleaning up or organizing data:
Sub RenamePatternBased()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
If InStr(ws.Name, “Report”) > 0 Then
ws.Name = Replace(ws.Name, “Report”, “RPT”)
End If
Next ws
End Sub
Handling Errors and Exceptions

VBA is powerful, but it’s also important to handle errors gracefully to avoid runtime errors, especially when renaming sheets:
- Error Handling: Use
On Error Resume Next
to skip over errors and continue executing. - Checking for Duplicate Names: Excel does not allow duplicate sheet names. Here’s how you can check for this:
Function SheetExists(strName As String) As Boolean On Error Resume Next SheetExists = (Sheets(strName).Name <> “”) On Error GoTo 0 End Function
Sub CheckAndRenameSheet() Dim ws As Worksheet Dim newName As String newName = “Budget” For Each ws In ThisWorkbook.Worksheets If Not SheetExists(newName) Then ws.Name = newName Exit Sub Else MsgBox “A sheet with the name ” & newName & “ already exists.”, vbExclamation End If Next ws End Sub
Conclusion

Mastering the art of renaming Excel sheets with VBA can significantly enhance your productivity when working with Excel. By automating this process, you not only save time but also reduce the likelihood of human error. The techniques discussed above offer a range of solutions, from simple renames to more complex, dynamic naming based on patterns or cell content. Whether you’re dealing with a few sheets or an entire workbook, these VBA scripts provide tools to streamline your workflow, ensuring that your Excel management tasks are done with efficiency and accuracy.
Can I rename sheets in Excel using keyboard shortcuts?

+
Unfortunately, there isn’t a direct keyboard shortcut to rename sheets in Excel. However, you can use Alt+H, then O, R to access the rename option, but this still requires manual entry of the new name.
Is it possible to rename sheets based on a column instead of a single cell?
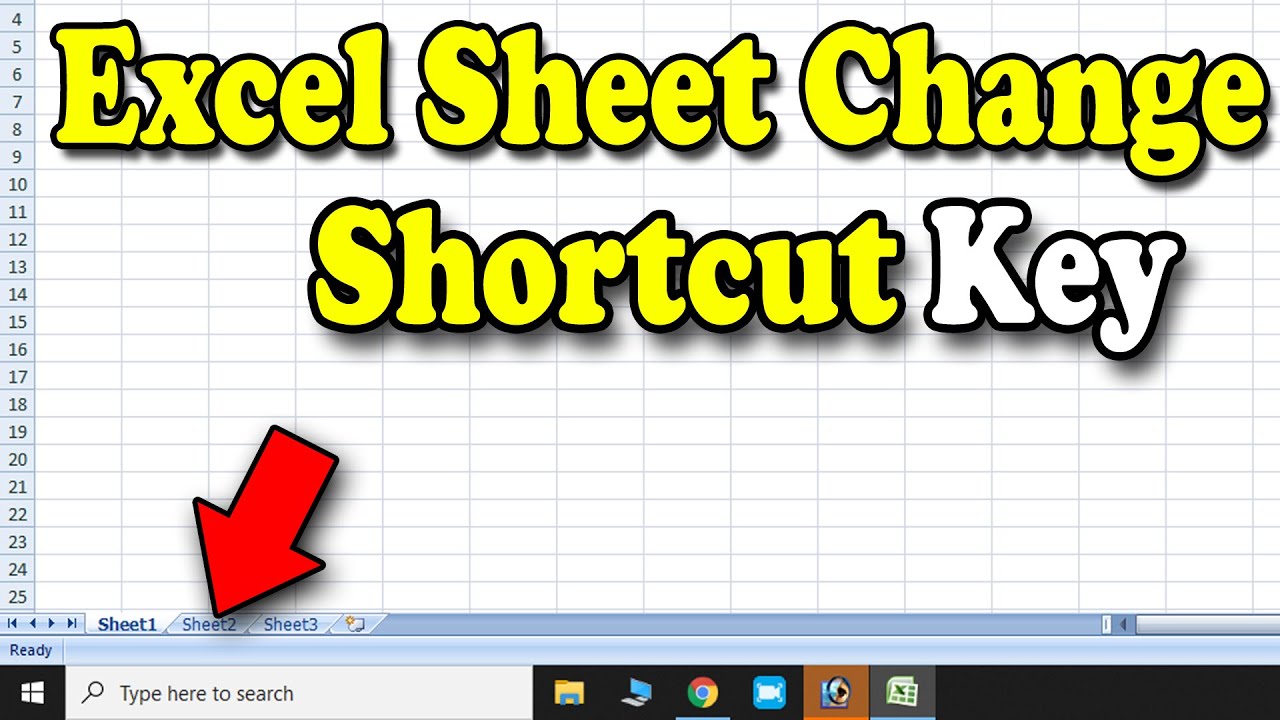
+
Yes, you can loop through each sheet and use a range of cells from that sheet to construct the new name. For example, you could concatenate values from multiple cells into a new sheet name.
What happens if I try to rename a sheet to a name already used?

+
Excel will not allow you to rename a sheet to a name that already exists. You would need to either rename or delete the existing sheet or modify the name to be unique.
How can I apply these renaming techniques to all open workbooks?

+
You can loop through Workbooks
instead of ThisWorkbook
. Here’s an example: Dim wb As Workbook; For Each wb In Application.Workbooks; For Each ws In wb.Worksheets; … Next ws; Next wb
.